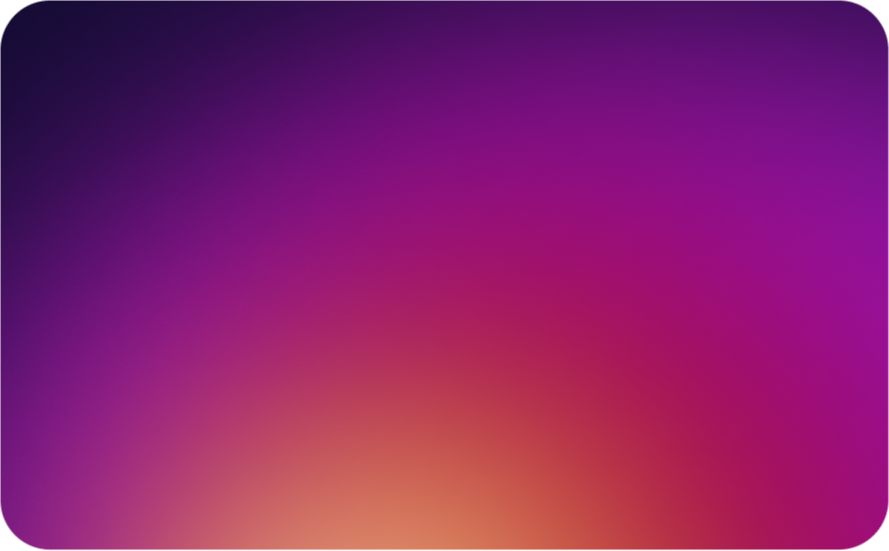
Audit Logging and Monitoring: Best Practices for Tempo Labs
Introduction
In the rapidly evolving landscape of vibe coding, security often takes a backseat to functionality and speed of development. This is particularly concerning when it comes to audit logging and monitoring - critical components that provide visibility into application behavior and potential security incidents. Tempo Labs, a popular vibe coding platform, offers powerful capabilities for building modern applications, but without proper logging and monitoring, you’re essentially flying blind when it comes to security.
The Blind Spots in Tempo Labs Applications
When developers use Tempo Labs for vibe coding, they often focus on rapidly building features and functionality. The platform excels at generating code quickly based on natural language prompts, but this convenience comes with security trade-offs. Common audit logging and monitoring blind spots in Tempo Labs applications include:
-
Insufficient Event Logging: By default, Tempo Labs applications often lack comprehensive event logging, recording only basic system events while missing critical user actions and security-relevant activities.
-
Missing Monitoring Infrastructure: Many Tempo Labs projects are deployed without proper monitoring solutions, making it impossible to detect anomalies or potential security breaches in real-time.
-
Inadequate Log Storage and Retention: Even when logging is implemented, logs are frequently stored insecurely or with insufficient retention periods, hampering incident investigation and compliance efforts.
These blind spots create significant security vulnerabilities that malicious actors can exploit without detection. Let’s explore how to address these issues with specific prompts for Tempo Labs.
Prompt 1: Implementing Comprehensive Audit Logging
When working with Tempo Labs, you can use the following prompt to generate code for a robust audit logging system:
Create a comprehensive audit logging system for my Tempo Labs application with the following requirements:
1. Log all authentication events (login attempts, successes, failures, logouts)
2. Track all user actions that modify data (create, update, delete operations)
3. Record administrative actions separately with higher detail level
4. Include contextual information in each log entry: timestamp, user ID, IP address, action type, affected resource, and result
5. Ensure logs are tamper-resistant by implementing digital signatures
6. Store logs securely with encryption at rest
7. Implement proper log rotation and retention policies (90 days online, 1 year archived)
8. Ensure the logging system doesn't impact application performance through asynchronous processing
Use best practices for secure logging and ensure the implementation is production-ready.
This prompt will guide Tempo Labs to generate code that implements a robust audit logging system. Here’s an example of what Tempo Labs might generate:
// Audit Logging Service for Tempo Labs Application
const winston = require('winston');
const { createHmac } = require('crypto');
const fs = require('fs');
const path = require('path');
class AuditLogger {
constructor(config) {
this.config = {
logDirectory: process.env.LOG_DIRECTORY || './logs',
retentionDays: 90,
encryptionKey: process.env.LOG_ENCRYPTION_KEY,
hmacSecret: process.env.LOG_HMAC_SECRET,
...config
};
this.setupLogDirectory();
this.initializeLogger();
this.logQueue = [];
this.processingQueue = false;
// Process logs asynchronously
setInterval(() => this.processLogQueue(), 1000);
}
setupLogDirectory() {
if (!fs.existsSync(this.config.logDirectory)) {
fs.mkdirSync(this.config.logDirectory, { recursive: true });
}
}
initializeLogger() {
this.logger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
transports: [
new winston.transports.File({
filename: path.join(this.config.logDirectory, 'audit.log'),
maxsize: 10485760, // 10MB
maxFiles: this.config.retentionDays,
tailable: true
})
]
});
// Also set up admin logs separately
this.adminLogger = winston.createLogger({
level: 'info',
format: winston.format.combine(
winston.format.timestamp(),
winston.format.json()
),
transports: [
new winston.transports.File({
filename: path.join(this.config.logDirectory, 'admin-audit.log'),
maxsize: 10485760, // 10MB
maxFiles: this.config.retentionDays,
tailable: true
})
]
});
}
// Create digital signature for log entry to prevent tampering
createSignature(logEntry) {
return createHmac('sha256', this.config.hmacSecret)
.update(JSON.stringify(logEntry))
.digest('hex');
}
// Queue log entry for asynchronous processing
queueLog(logEntry) {
this.logQueue.push(logEntry);
}
// Process queued logs asynchronously
async processLogQueue() {
if (this.processingQueue || this.logQueue.length === 0) return;
this.processingQueue = true;
const batchSize = Math.min(100, this.logQueue.length);
const batch = this.logQueue.splice(0, batchSize);
for (const entry of batch) {
// Add digital signature
entry.signature = this.createSignature(entry);
// Write to appropriate log
if (entry.category === 'admin') {
this.adminLogger.info(entry);
} else {
this.logger.info(entry);
}
}
this.processingQueue = false;
}
// Log authentication events
logAuthentication(event) {
const logEntry = {
timestamp: new Date().toISOString(),
category: 'authentication',
userId: event.userId || 'anonymous',
ipAddress: event.ipAddress,
action: event.action, // login_attempt, login_success, login_failure, logout
result: event.result,
details: event.details || {}
};
this.queueLog(logEntry);
}
// Log data modification events
logDataModification(event) {
const logEntry = {
timestamp: new Date().toISOString(),
category: 'data_modification',
userId: event.userId,
ipAddress: event.ipAddress,
action: event.action, // create, update, delete
resourceType: event.resourceType,
resourceId: event.resourceId,
changes: event.changes,
result: event.result
};
this.queueLog(logEntry);
}
// Log administrative actions
logAdminAction(event) {
const logEntry = {
timestamp: new Date().toISOString(),
category: 'admin',
userId: event.userId,
ipAddress: event.ipAddress,
action: event.action,
resourceType: event.resourceType,
resourceId: event.resourceId,
changes: event.changes,
result: event.result,
details: event.details || {}
};
this.queueLog(logEntry);
}
// Archive logs older than retention period
archiveLogs() {
// Implementation for archiving logs to long-term storage
// This would typically move logs to a more cost-effective storage
// while maintaining their integrity and accessibility for compliance
}
}
module.exports = new AuditLogger();
This implementation provides a solid foundation for audit logging in your Tempo Labs application. It captures essential security events, ensures logs are tamper-resistant with digital signatures, and processes logs asynchronously to minimize performance impact.
Prompt 2: Setting Up Real-Time Monitoring and Alerting
Logging is only half the battle - you also need effective monitoring to detect and respond to security incidents. Use this prompt to generate code for a monitoring system:
Create a comprehensive monitoring and alerting system for my Tempo Labs application that will help detect security incidents. Include:
1. Real-time dashboard for visualizing application metrics and security events
2. Anomaly detection for identifying unusual patterns in user behavior
3. Threshold-based alerts for critical security events (multiple failed logins, unusual data access patterns, etc.)
4. Integration with popular notification channels (email, Slack, PagerDuty)
5. Automated response capabilities for common security incidents
6. Performance monitoring to detect potential DoS attacks
7. API endpoint monitoring to identify potential abuse
8. Database query monitoring to detect potential injection attacks
Ensure the system is lightweight and doesn't significantly impact application performance.
Here’s an example of what Tempo Labs might generate:
// Security Monitoring and Alerting System for Tempo Labs Application
const express = require('express');
const prometheus = require('prom-client');
const nodemailer = require('nodemailer');
const { WebClient } = require('@slack/web-api');
const axios = require('axios');
class SecurityMonitor {
constructor(config) {
this.config = {
alertThresholds: {
failedLogins: 5, // 5 failed logins within timeWindow
unusualDataAccess: 10, // 10 unusual data access patterns within timeWindow
apiRateLimit: 100, // 100 requests per minute from same IP
databaseQueryTime: 5000 // queries taking longer than 5 seconds
},
timeWindows: {
failedLogins: 5 * 60 * 1000, // 5 minutes
unusualDataAccess: 10 * 60 * 1000, // 10 minutes
apiRateLimit: 60 * 1000 // 1 minute
},
notificationChannels: {
email: process.env.ALERT_EMAIL,
slack: {
token: process.env.SLACK_TOKEN,
channel: process.env.SLACK_CHANNEL
},
pagerDuty: {
routingKey: process.env.PAGERDUTY_ROUTING_KEY
}
},
...config
};
this.setupMetrics();
this.setupEventTracking();
this.setupDashboard();
// Start anomaly detection system
setInterval(() => this.detectAnomalies(), 60000);
}
setupMetrics() {
// Initialize Prometheus metrics
prometheus.collectDefaultMetrics();
// Custom security metrics
this.loginAttemptsCounter = new prometheus.Counter({
name: 'login_attempts_total',
help: 'Total number of login attempts',
labelNames: ['status', 'user_type']
});
this.dataAccessCounter = new prometheus.Counter({
name: 'data_access_total',
help: 'Total number of data access operations',
labelNames: ['operation', 'resource_type', 'user_type']
});
this.apiRequestsCounter = new prometheus.Counter({
name: 'api_requests_total',
help: 'Total number of API requests',
labelNames: ['endpoint', 'method', 'status_code']
});
this.databaseQueryTimer = new prometheus.Histogram({
name: 'database_query_duration_seconds',
help: 'Database query duration in seconds',
labelNames: ['query_type'],
buckets: [0.1, 0.5, 1, 2, 5, 10]
});
}
setupEventTracking() {
// Track security events
this.securityEvents = {
failedLogins: [], // {timestamp, userId, ipAddress}
dataAccess: [], // {timestamp, userId, ipAddress, resourceType, resourceId}
apiRequests: {}, // {ipAddress: {count, timestamps}}
longQueries: [] // {timestamp, queryType, duration, query}
};
}
setupDashboard() {
// Create Express server for metrics endpoint and dashboard
const app = express();
// Expose metrics endpoint for Prometheus scraping
app.get('/metrics', (req, res) => {
res.set('Content-Type', prometheus.register.contentType);
res.end(prometheus.register.metrics());
});
// Simple dashboard for real-time monitoring
app.get('/dashboard', (req, res) => {
// In a real implementation, this would serve a proper dashboard
// For this example, we'll just return some basic HTML
res.send(`
<html>
<head>
<title>Security Monitoring Dashboard</title>
<meta http-equiv="refresh" content="30">
<style>
body { font-family: Arial, sans-serif; margin: 20px; }
.metric { margin-bottom: 20px; padding: 10px; border: 1px solid #ccc; }
.alert { background-color: #ffdddd; }
</style>
</head>
<body>
<h1>Security Monitoring Dashboard</h1>
<div class="metric">
<h2>Failed Logins (Last Hour)</h2>
<p>${this.securityEvents.failedLogins.filter(e =>
e.timestamp > Date.now() - 3600000).length}</p>
</div>
<div class="metric">
<h2>Unusual Data Access (Last Hour)</h2>
<p>${this.securityEvents.dataAccess.filter(e =>
e.timestamp > Date.now() - 3600000 && e.unusual).length}</p>
</div>
<div class="metric">
<h2>API Rate Limit Violations (Last Hour)</h2>
<p>${Object.values(this.securityEvents.apiRequests)
.filter(ip => ip.violations > 0).length}</p>
</div>
<div class="metric">
<h2>Long-Running Queries (Last Hour)</h2>
<p>${this.securityEvents.longQueries.filter(e =>
e.timestamp > Date.now() - 3600000).length}</p>
</div>
<h2>Recent Alerts</h2>
<div id="alerts">
${this.recentAlerts.map(alert =>
`<div class="metric alert">
<h3>${alert.type} - ${new Date(alert.timestamp).toLocaleString()}</h3>
<p>${alert.message}</p>
</div>`
).join('')}
</div>
</body>
</html>
`);
});
// Start the server
const port = process.env.MONITOR_PORT || 3001;
app.listen(port, () => {
console.log(`Security monitoring dashboard available at http://localhost:${port}/dashboard`);
});
}
// Track login attempts
trackLoginAttempt(event) {
this.loginAttemptsCounter.inc({
status: event.success ? 'success' : 'failure',
user_type: event.userType || 'standard'
});
if (!event.success) {
this.securityEvents.failedLogins.push({
timestamp: Date.now(),
userId: event.userId,
ipAddress: event.ipAddress
});
// Check for threshold violation
this.checkFailedLoginThreshold(event.userId, event.ipAddress);
}
}
// Track data access
trackDataAccess(event) {
this.dataAccessCounter.inc({
operation: event.operation,
resource_type: event.resourceType,
user_type: event.userType || 'standard'
});
// Store the event for anomaly detection
this.securityEvents.dataAccess.push({
timestamp: Date.now(),
userId: event.userId,
ipAddress: event.ipAddress,
resourceType: event.resourceType,
resourceId: event.resourceId,
unusual: false // Will be updated by anomaly detection
});
}
// Track API requests
trackApiRequest(event) {
this.apiRequestsCounter.inc({
endpoint: event.endpoint,
method: event.method,
status_code: event.statusCode
});
// Track for rate limiting
const ip = event.ipAddress;
if (!this.securityEvents.apiRequests[ip]) {
this.securityEvents.apiRequests[ip] = {
count: 0,
timestamps: [],
violations: 0
};
}
const ipData = this.securityEvents.apiRequests[ip];
ipData.count++;
ipData.timestamps.push(Date.now());
// Remove timestamps outside the time window
const timeWindow = this.config.timeWindows.apiRateLimit;
ipData.timestamps = ipData.timestamps.filter(t => t > Date.now() - timeWindow);
// Check for rate limit violation
if (ipData.timestamps.length > this.config.alertThresholds.apiRateLimit) {
ipData.violations++;
this.triggerAlert('API_RATE_LIMIT', {
ipAddress: ip,
requestCount: ipData.timestamps.length,
timeWindow: timeWindow / 1000
});
}
}
// Track database queries
trackDatabaseQuery(event) {
const durationSeconds = event.duration / 1000;
this.databaseQueryTimer.observe({
query_type: event.queryType
}, durationSeconds);
// Check for long-running queries
if (event.duration > this.config.alertThresholds.databaseQueryTime) {
this.securityEvents.longQueries.push({
timestamp: Date.now(),
queryType: event.queryType,
duration: event.duration,
query: event.query
});
this.triggerAlert('LONG_QUERY', {
queryType: event.queryType,
duration: event.duration,
query: event.query
});
}
}
// Check for failed login threshold violations
checkFailedLoginThreshold(userId, ipAddress) {
const timeWindow = this.config.timeWindows.failedLogins;
const threshold = this.config.alertThresholds.failedLogins;
// Count failed logins for this user/IP within the time window
const recentFailures = this.securityEvents.failedLogins.filter(event =>
event.timestamp > Date.now() - timeWindow &&
(event.userId === userId || event.ipAddress === ipAddress)
);
if (recentFailures.length >= threshold) {
this.triggerAlert('FAILED_LOGINS', {
userId,
ipAddress,
count: recentFailures.length,
timeWindow: timeWindow / 1000 / 60
});
// Automated response - could implement account lockout here
}
}
// Detect anomalies in user behavior
detectAnomalies() {
// This is a simplified implementation
// A real system would use more sophisticated algorithms
// Group data access by user
const userAccessMap = {};
this.securityEvents.dataAccess.forEach(event => {
if (!userAccessMap[event.userId]) {
userAccessMap[event.userId] = [];
}
userAccessMap[event.userId].push(event);
});
// For each user, check if current access patterns differ from historical patterns
Object.entries(userAccessMap).forEach(([userId, events]) => {
// Get recent events
const recentEvents = events.filter(e =>
e.timestamp > Date.now() - this.config.timeWindows.unusualDataAccess
);
// Count access by resource type
const resourceTypeCounts = {};
recentEvents.forEach(event => {
if (!resourceTypeCounts[event.resourceType]) {
resourceTypeCounts[event.resourceType] = 0;
}
resourceTypeCounts[event.resourceType]++;
});
// Check for unusual access patterns
// This is a very simplified approach - real systems would use more sophisticated methods
Object.entries(resourceTypeCounts).forEach(([resourceType, count]) => {
// If a user suddenly accesses a resource type many times in a short period
if (count > this.config.alertThresholds.unusualDataAccess) {
// Mark these events as unusual
recentEvents
.filter(e => e.resourceType === resourceType)
.forEach(e => { e.unusual = true; });
this.triggerAlert('UNUSUAL_DATA_ACCESS', {
userId,
resourceType,
count,
timeWindow: this.config.timeWindows.unusualDataAccess / 1000 / 60
});
}
});
});
// Clean up old events
const oldestTimestamp = Date.now() - 24 * 60 * 60 * 1000; // 24 hours
this.securityEvents.failedLogins = this.securityEvents.failedLogins
.filter(e => e.timestamp > oldestTimestamp);
this.securityEvents.dataAccess = this.securityEvents.dataAccess
.filter(e => e.timestamp > oldestTimestamp);
this.securityEvents.longQueries = this.securityEvents.longQueries
.filter(e => e.timestamp > oldestTimestamp);
// Clean up old API request data
Object.keys(this.securityEvents.apiRequests).forEach(ip => {
if (this.securityEvents.apiRequests[ip].timestamps.length === 0) {
delete this.securityEvents.apiRequests[ip];
}
});
}
// Store recent alerts for dashboard display
recentAlerts = [];
// Trigger an alert
triggerAlert(type, data) {
console.log(`SECURITY ALERT: ${type}`, data);
// Create alert message
let message;
switch (type) {
case 'FAILED_LOGINS':
message = `Multiple failed login attempts (${data.count}) for user ${data.userId} from IP ${data.ipAddress} within ${data.timeWindow} minutes`;
break;
case 'UNUSUAL_DATA_ACCESS':
message = `Unusual data access pattern detected for user ${data.userId}: ${data.count} accesses to ${data.resourceType} resources within ${data.timeWindow} minutes`;
break;
case 'API_RATE_LIMIT':
message = `API rate limit exceeded: ${data.requestCount} requests from IP ${data.ipAddress} within ${data.timeWindow} seconds`;
break;
case 'LONG_QUERY':
message = `Long-running database query detected: ${data.queryType} query took ${data.duration}ms`;
break;
default:
message = `Security alert: ${type}`;
}
// Add to recent alerts
this.recentAlerts.unshift({
type,
timestamp: Date.now(),
message,
data
});
// Keep only the 10 most recent alerts
if (this.recentAlerts.length > 10) {
this.recentAlerts.pop();
}
// Send notifications
this.sendNotifications(type, message, data);
// Implement automated responses
this.automatedResponse(type, data);
}
// Send notifications to configured channels
async sendNotifications(type, message, data) {
// Email notification
if (this.config.notificationChannels.email) {
try {
const transporter = nodemailer.createTransport({
service: 'gmail',
auth: {
user: process.env.EMAIL_USER,
pass: process.env.EMAIL_PASSWORD
}
});
await transporter.sendMail({
from: process.env.EMAIL_USER,
to: this.config.notificationChannels.email,
subject: `Security Alert: ${type}`,
text: message,
html: `<p><strong>Security Alert: ${type}</strong></p><p>${message}</p><pre>${JSON.stringify(data, null, 2)}</pre>`
});
} catch (error) {
console.error('Failed to send email notification:', error);
}
}
// Slack notification
if (this.config.notificationChannels.slack?.token) {
try {
const slack = new WebClient(this.config.notificationChannels.slack.token);
await slack.chat.postMessage({
channel: this.config.notificationChannels.slack.channel,
text: `*Security Alert: ${type}*\n${message}`,
blocks: [
{
type: 'section',
text: {
type: 'mrkdwn',
text: `*Security Alert: ${type}*\n${message}`
}
},
{
type: 'section',
text: {
type: 'mrkdwn',
text: '```' + JSON.stringify(data, null, 2) + '```'
}
}
]
});
} catch (error) {
console.error('Failed to send Slack notification:', error);
}
}
// PagerDuty notification for critical alerts
if (this.config.notificationChannels.pagerDuty?.routingKey &&
['FAILED_LOGINS', 'UNUSUAL_DATA_ACCESS'].includes(type)) {
try {
await axios.post('https://events.pagerduty.com/v2/enqueue', {
routing_key: this.config.notificationChannels.pagerDuty.routingKey,
event_action: 'trigger',
payload: {
summary: `Security Alert: ${type}`,
source: 'Security Monitoring System',
severity: 'critical',
custom_details: {
message,
...data
}
}
});
} catch (error) {
console.error('Failed to send PagerDuty notification:', error);
}
}
}
// Implement automated responses to security incidents
automatedResponse(type, data) {
switch (type) {
case 'FAILED_LOGINS':
// Could implement temporary account lockout
console.log(`[AUTOMATED RESPONSE] Temporarily locking account for user ${data.userId}`);
// In a real implementation, this would call an account management service
break;
case 'API_RATE_LIMIT':
// Could implement IP blocking
console.log(`[AUTOMATED RESPONSE] Temporarily blocking requests from IP ${data.ipAddress}`);
// In a real implementation, this would update a blocklist
break;
case 'UNUSUAL_DATA_ACCESS':
// Could implement additional verification requirements
console.log(`[AUTOMATED RESPONSE] Requiring additional verification for user ${data.userId}`);
// In a real implementation, this would flag the user for additional verification
break;
}
}
}
module.exports = new SecurityMonitor();
This implementation provides a comprehensive monitoring and alerting system for your Tempo Labs application. It tracks security-relevant events, detects anomalies, and sends alerts through multiple channels when potential security incidents are detected.
Prompt 3: Creating a Security Dashboard for Visualization
To complete your audit logging and monitoring setup, you need a way to visualize security events and metrics. Use this prompt to generate code for a security dashboard:
Create a security dashboard for my Tempo Labs application that visualizes audit logs and security metrics. The dashboard should:
1. Display real-time security metrics (login attempts, failed logins, data access patterns)
2. Visualize security events over time with interactive charts
3. Show recent security alerts with severity levels
4. Provide filtering capabilities to focus on specific time periods or event types
5. Include a user activity timeline to track suspicious behavior
6. Offer drill-down capabilities for investigating specific events
7. Present geographic visualizations of access attempts
8. Include a summary of system health and security posture
Use modern frontend technologies that integrate well with Tempo Labs.
Here’s an example of what Tempo Labs might generate:
// Security Dashboard for Tempo Labs Application
// frontend/src/components/SecurityDashboard.jsx
import React, { useState, useEffect } from 'react';
import {
LineChart, Line, BarChart, Bar, PieChart, Pie,
AreaChart, Area, XAxis, YAxis, CartesianGrid,
Tooltip, Legend, ResponsiveContainer
} from 'recharts';
import {
Card, CardHeader, CardContent, Grid, Typography,
Table, TableBody, TableCell, TableContainer,
TableHead, TableRow, Paper, Button, Select,
MenuItem, FormControl, InputLabel, Box, Chip,
Dialog, DialogTitle, DialogContent, DialogActions
} from '@mui/material';
import {
Warning as WarningIcon,
Error as ErrorIcon,
Info as InfoIcon,
CheckCircle as CheckCircleIcon,
Security as SecurityIcon,
Timeline as TimelineIcon,
Map as MapIcon,
Person as PersonIcon
} from '@mui/icons-material';
import { MapContainer, TileLayer, Marker, Popup } from 'react-leaflet';
import 'leaflet/dist/leaflet.css';
import moment from 'moment';
// Mock API service - in a real app, this would connect to your backend
const securityApi = {
getMetrics: async (timeRange) => {
// This would be a real API call in production
return new Promise((resolve) => {
setTimeout(() => {
resolve({
loginAttempts: {
total: 1243,
successful: 1156,
failed: 87,
byHour: Array(24).fill().map((_, i) => ({
hour: i,
successful: Math.floor(Math.random() * 100),
failed: Math.floor(Math.random() * 10)
}))
},
dataAccess: {
total: 5678,
byResourceType: {
users: 1245,
products: 2456,
orders: 1977
},
byOperation: {
read: 4532,
create: 567,
update: 456,
delete: 123
}
},
apiRequests: {
total: 12435,
byEndpoint: {
'/api/users': 2345,
'/api/products': 5678,
'/api/orders': 4412
},
byStatusCode: {
'200': 11234,
'400': 876,
'401': 234,
'403': 56,
'500': 35
}
}
});
}, 500);
});
},
getAlerts: async (timeRange) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve([
{
id: 1,
type: 'FAILED_LOGINS',
severity: 'high',
timestamp: Date.now() - 1000 * 60 * 15,
message: 'Multiple failed login attempts (6) for user john.doe from IP 192.168.1.105 within 5 minutes',
data: {
userId: 'john.doe',
ipAddress: '192.168.1.105',
count: 6,
timeWindow: 5
},
status: 'open'
},
{
id: 2,
type: 'UNUSUAL_DATA_ACCESS',
severity: 'medium',
timestamp: Date.now() - 1000 * 60 * 45,
message: 'Unusual data access pattern detected for user admin: 15 accesses to users resources within 10 minutes',
data: {
userId: 'admin',
resourceType: 'users',
count: 15,
timeWindow: 10
},
status: 'investigating'
},
{
id: 3,
type: 'API_RATE_LIMIT',
severity: 'medium',
timestamp: Date.now() - 1000 * 60 * 120,
message: 'API rate limit exceeded: 120 requests from IP 203.0.113.42 within 60 seconds',
data: {
ipAddress: '203.0.113.42',
requestCount: 120,
timeWindow: 60
},
status: 'resolved'
},
{
id: 4,
type: 'LONG_QUERY',
severity: 'low',
timestamp: Date.now() - 1000 * 60 * 180,
message: 'Long-running database query detected: SELECT query took 6532ms',
data: {
queryType: 'SELECT',
duration: 6532,
query: 'SELECT * FROM orders WHERE...'
},
status: 'resolved'
}
]);
}, 300);
});
},
getUserActivity: async (userId) => {
return new Promise((resolve) => {
setTimeout(() => {
resolve({
user: {
id: userId,
name: 'John Doe',
email: 'john.doe@example.com',
role: 'admin'
},
activity: [
{
type: 'login',
timestamp: Date.now() - 1000 * 60 * 10,
ipAddress: '192.168.1.105',
userAgent: 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36'
},
{
type: 'data_access',
timestamp: Date.now() - 1000 * 60 * 9,
resourceType: 'users',
operation: 'read',
resourceId: 'all'
},
{
type: 'data_access',
timestamp: Date.now() - 1000 * 60 * 8,
resourceType: 'users',
operation: 'update',
resourceId: 'user_123'
},
{
type: 'api_request',
timestamp: Date.now() - 1000 * 60 * 7,
endpoint: '/api/users/123',
method: 'PUT',
statusCode: 200
},
{
type: 'data_access',
timestamp: Date.now() - 1000 * 60 * 5,
resourceType: 'products',
operation: 'create',
resourceId: 'product_456'
}
],
locations: [
{
ipAddress: '192.168.1.105',
location: {
lat: 37.7749,
lng: -122.4194,
city: 'San Francisco',
country: 'United States'
},
count: 12
},
{
ipAddress: '203.0.113.42',
location: {
lat: 40.7128,
lng: -74.0060,
city: 'New York',
country: 'United States'
},
count: 3
}
]
});
}, 400);
});
},
getSystemHealth: async () => {
return new Promise((resolve) => {
setTimeout(() => {
resolve({
overall: 'healthy',
components: {
api: 'healthy',
database: 'healthy',
authentication: 'healthy',
storage: 'degraded'
},
securityPosture: {
vulnerabilities: {
critical: 0,
high: 2,
medium: 5,
low: 12
},
patchStatus: '92% up to date',
lastSecurityScan: Date.now() - 1000 * 60 * 60 * 12
}
});
}, 300);
});
}
};
// Severity icon mapping
const SeverityIcon = ({ severity }) => {
switch (severity) {
case 'critical':
return <ErrorIcon color="error" />;
case 'high':
return <WarningIcon style={{ color: '#ff9800' }} />;
case 'medium':
return <WarningIcon style={{ color: '#ffb74d' }} />;
case 'low':
return <InfoIcon color="primary" />;
default:
return <InfoIcon />;
}
};
// Status chip mapping
const StatusChip = ({ status }) => {
switch (status) {
case 'open':
return <Chip label="Open" color="error" size="small" />;
case 'investigating':
return <Chip label="Investigating" color="warning" size="small" />;
case 'resolved':
return <Chip label="Resolved" color="success" size="small" />;
default:
return <Chip label={status} size="small" />;
}
};
const SecurityDashboard = () => {
const [timeRange, setTimeRange] = useState('24h');
const [metrics, setMetrics] = useState(null);
const [alerts, setAlerts] = useState([]);
const [selectedUser, setSelectedUser] = useState(null);
const [userActivity, setUserActivity] = useState(null);
const [systemHealth, setSystemHealth] = useState(null);
const [selectedAlert, setSelectedAlert] = useState(null);
const [loading, setLoading] = useState(true);
useEffect(() => {
const fetchData = async () => {
setLoading(true);
try {
const [metricsData, alertsData, healthData] = await Promise.all([
securityApi.getMetrics(timeRange),
securityApi.getAlerts(timeRange),
securityApi.getSystemHealth()
]);
setMetrics(metricsData);
setAlerts(alertsData);
setSystemHealth(healthData);
} catch (error) {
console.error('Error fetching security data:', error);
} finally {
setLoading(false);
}
};
fetchData();
// Set up polling for real-time updates
const interval = setInterval(fetchData, 30000);
return () => clearInterval(interval);
}, [timeRange]);
useEffect(() => {
if (selectedUser) {
securityApi.getUserActivity(selectedUser)
.then(data => setUserActivity(data))
.catch(error => console.error('Error fetching user activity:', error));
} else {
setUserActivity(null);
}
}, [selectedUser]);
const handleTimeRangeChange = (event) => {
setTimeRange(event.target.value);
};
const handleAlertClick = (alert) => {
setSelectedAlert(alert);
};
const handleCloseAlertDialog = () => {
setSelectedAlert(null);
};
const handleUserClick = (userId) => {
setSelectedUser(userId);
};
if (loading && !metrics) {
return (
<Box display="flex" justifyContent="center" alignItems="center" height="100vh">
<Typography variant="h5">Loading security dashboard...</Typography>
</Box>
);
}
return (
<Box p={3}>
<Box display="flex" justifyContent="space-between" alignItems="center" mb={3}>
<Typography variant="h4" component="h1">
<SecurityIcon fontSize="large" style={{ marginRight: 8, verticalAlign: 'middle' }} />
Security Dashboard
</Typography>
<FormControl variant="outlined" style={{ minWidth: 120 }}>
<InputLabel>Time Range</InputLabel>
<Select
value={timeRange}
onChange={handleTimeRangeChange}
label="Time Range"
>
<MenuItem value="1h">Last Hour</MenuItem>
<MenuItem value="6h">Last 6 Hours</MenuItem>
<MenuItem value="24h">Last 24 Hours</MenuItem>
<MenuItem value="7d">Last 7 Days</MenuItem>
<MenuItem value="30d">Last 30 Days</MenuItem>
</Select>
</FormControl>
</Box>
<Grid container spacing={3}>
{/* System Health Card */}
<Grid item xs={12} md={6}>
<Card>
<CardHeader title="System Health & Security Posture" />
<CardContent>
{systemHealth && (
<>
<Box display="flex" alignItems="center" mb={2}>
<Typography variant="h6" component="span">Overall Status: </Typography>
<Chip
label={systemHealth.overall.toUpperCase()}
color={systemHealth.overall === 'healthy' ? 'success' : 'warning'}
style={{ marginLeft: 8 }}
/>
</Box>
<Typography variant="subtitle1">Component Status:</Typography>
<Grid container spacing={1} mb={2}>
{Object.entries(systemHealth.components).map(([component, status]) => (
<Grid item xs={6} sm={3} key={component}>
<Chip
label={`${component}: ${status}`}
color={status === 'healthy' ? 'success' : 'warning'}
size="small"
style={{ margin: 4 }}
/>
</Grid>
))}
</Grid>
<Typography variant="subtitle1">Security Vulnerabilities:</Typography>
<ResponsiveContainer width="100%" height={150}>
<PieChart>
<Pie
data={[
{ name: 'Critical', value: systemHealth.securityPosture.vulnerabilities.critical, fill: '#f44336' },
{ name: 'High', value: systemHealth.securityPosture.vulnerabilities.high, fill: '#ff9800' },
{ name: 'Medium', value: systemHealth.securityPosture.vulnerabilities.medium, fill: '#ffb74d' },
{ name: 'Low', value: systemHealth.securityPosture.vulnerabilities.low, fill: '#2196f3' }
]}
cx="50%"
cy="50%"
outerRadius={60}
dataKey="value"
label={({ name, value }) => `${name}: ${value}`}
/>
<Tooltip />
</PieChart>
</ResponsiveContainer>
<Typography variant="body2">
Patch Status: {systemHealth.securityPosture.patchStatus}
</Typography>
<Typography variant="body2">
Last Security Scan: {moment(systemHealth.securityPosture.lastSecurityScan).fromNow()}
</Typography>
</>
)}
</CardContent>
</Card>
</Grid>
{/* Login Metrics Card */}
<Grid item xs={12} md={6}>
<Card>
<CardHeader title="Authentication Activity" />
<CardContent>
{metrics && (
<>
<Box display="flex" justifyContent="space-around" mb={2}>
<Box textAlign="center">
<Typography variant="h4">{metrics.loginAttempts.total}</Typography>
<Typography variant="body2">Total Login Attempts</Typography>
</Box>
<Box textAlign="center">
<Typography variant="h4" color="success.main">{metrics.loginAttempts.successful}</Typography>
<Typography variant="body2">Successful Logins</Typography>
</Box>
<Box textAlign="center">
<Typography variant="h4" color="error.main">{metrics.loginAttempts.failed}</Typography>
<Typography variant="body2">Failed Logins</Typography>
</Box>
</Box>
<ResponsiveContainer width="100%" height={200}>
<AreaChart data={metrics.loginAttempts.byHour}>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="hour" label={{ value: 'Hour', position: 'insideBottom', offset: -5 }} />
<YAxis />
<Tooltip />
<Legend />
<Area type="monotone" dataKey="successful" stackId="1" stroke="#4caf50" fill="#4caf50" />
<Area type="monotone" dataKey="failed" stackId="1" stroke="#f44336" fill="#f44336" />
</AreaChart>
</ResponsiveContainer>
</>
)}
</CardContent>
</Card>
</Grid>
{/* Data Access Metrics Card */}
<Grid item xs={12} md={6}>
<Card>
<CardHeader title="Data Access Patterns" />
<CardContent>
{metrics && (
<>
<Typography variant="h6" gutterBottom>
Total Data Access Operations: {metrics.dataAccess.total}
</Typography>
<Typography variant="subtitle1">By Resource Type:</Typography>
<ResponsiveContainer width="100%" height={150}>
<PieChart>
<Pie
data={Object.entries(metrics.dataAccess.byResourceType).map(([key, value]) => ({
name: key,
value
}))}
cx="50%"
cy="50%"
outerRadius={60}
dataKey="value"
label={({ name, value }) => `${name}: ${value}`}
/>
<Tooltip />
</PieChart>
</ResponsiveContainer>
<Typography variant="subtitle1">By Operation:</Typography>
<ResponsiveContainer width="100%" height={150}>
<BarChart
data={Object.entries(metrics.dataAccess.byOperation).map(([key, value]) => ({
name: key,
value
}))}
>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="name" />
<YAxis />
<Tooltip />
<Bar dataKey="value" fill="#8884d8" />
</BarChart>
</ResponsiveContainer>
</>
)}
</CardContent>
</Card>
</Grid>
{/* API Metrics Card */}
<Grid item xs={12} md={6}>
<Card>
<CardHeader title="API Request Metrics" />
<CardContent>
{metrics && (
<>
<Typography variant="h6" gutterBottom>
Total API Requests: {metrics.apiRequests.total}
</Typography>
<Typography variant="subtitle1">By Status Code:</Typography>
<ResponsiveContainer width="100%" height={150}>
<BarChart
data={Object.entries(metrics.apiRequests.byStatusCode).map(([key, value]) => ({
name: key,
value
}))}
>
<CartesianGrid strokeDasharray="3 3" />
<XAxis dataKey="name" />
<YAxis />
<Tooltip />
<Bar
dataKey="value"
fill={(entry) => {
const code = entry.name;
if (code.startsWith('2')) return '#4caf50';
if (code.startsWith('4')) return '#ff9800';
if (code.startsWith('5')) return '#f44336';
return '#2196f3';
}}
/>
</BarChart>
</ResponsiveContainer>
</>
)}
</CardContent>
</Card>
</Grid>
{/* Security Alerts Card */}
<Grid item xs={12}>
<Card>
<CardHeader
title="Security Alerts"
action={
<Button
variant="outlined"
color="primary"
onClick={() => {/* View all alerts */}}
>
View All
</Button>
}
/>
<CardContent>
<TableContainer component={Paper}>
<Table>
<TableHead>
<TableRow>
<TableCell>Severity</TableCell>
<TableCell>Type</TableCell>
<TableCell>Message</TableCell>
<TableCell>Time</TableCell>
<TableCell>Status</TableCell>
<TableCell>Actions</TableCell>
</TableRow>
</TableHead>
<TableBody>
{alerts.map((alert) => (
<TableRow key={alert.id}>
<TableCell>
<SeverityIcon severity={alert.severity} />
</TableCell>
<TableCell>{alert.type}</TableCell>
<TableCell>{alert.message}</TableCell>
<TableCell>{moment(alert.timestamp).fromNow()}</TableCell>
<TableCell>
<StatusChip status={alert.status} />
</TableCell>
<TableCell>
<Button
size="small"
onClick={() => handleAlertClick(alert)}
>
Details
</Button>
</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
</CardContent>
</Card>
</Grid>
{/* User Activity Card - Shown when a user is selected */}
{userActivity && (
<Grid item xs={12}>
<Card>
<CardHeader
title={
<Box display="flex" alignItems="center">
<PersonIcon style={{ marginRight: 8 }} />
User Activity: {userActivity.user.name}
</Box>
}
action={
<Button
variant="outlined"
color="primary"
onClick={() => setSelectedUser(null)}
>
Close
</Button>
}
/>
<CardContent>
<Grid container spacing={3}>
<Grid item xs={12} md={6}>
<Typography variant="h6" gutterBottom>
<TimelineIcon style={{ verticalAlign: 'middle', marginRight: 8 }} />
Activity Timeline
</Typography>
<TableContainer component={Paper} style={{ maxHeight: 400, overflow: 'auto' }}>
<Table size="small">
<TableHead>
<TableRow>
<TableCell>Time</TableCell>
<TableCell>Activity</TableCell>
<TableCell>Details</TableCell>
</TableRow>
</TableHead>
<TableBody>
{userActivity.activity.map((activity, index) => (
<TableRow key={index}>
<TableCell>{moment(activity.timestamp).format('HH:mm:ss')}</TableCell>
<TableCell>{activity.type.replace('_', ' ')}</TableCell>
<TableCell>
{activity.type === 'login' && (
<>IP: {activity.ipAddress}</>
)}
{activity.type === 'data_access' && (
<>{activity.operation} {activity.resourceType} {activity.resourceId}</>
)}
{activity.type === 'api_request' && (
<>{activity.method} {activity.endpoint} ({activity.statusCode})</>
)}
</TableCell>
</TableRow>
))}
</TableBody>
</Table>
</TableContainer>
</Grid>
<Grid item xs={12} md={6}>
<Typography variant="h6" gutterBottom>
<MapIcon style={{ verticalAlign: 'middle', marginRight: 8 }} />
Access Locations
</Typography>
<Box height={400} border="1px solid #ccc" borderRadius={1}>
<MapContainer
center={[39.8283, -98.5795]}
zoom={3}
style={{ height: '100%', width: '100%' }}
>
<TileLayer
url="https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png"
attribution='© <a href="https://www.openstreetmap.org/copyright">OpenStreetMap</a> contributors'
/>
{userActivity.locations.map((location, index) => (
<Marker
key={index}
position={[location.location.lat, location.location.lng]}
>
<Popup>
<div>
<strong>IP:</strong> {location.ipAddress}<br />
<strong>Location:</strong> {location.location.city}, {location.location.country}<br />
<strong>Access Count:</strong> {location.count}
</div>
</Popup>
</Marker>
))}
</MapContainer>
</Box>
</Grid>
</Grid>
</CardContent>
</Card>
</Grid>
)}
</Grid>
{/* Alert Details Dialog */}
<Dialog
open={!!selectedAlert}
onClose={handleCloseAlertDialog}
maxWidth="md"
fullWidth
>
{selectedAlert && (
<>
<DialogTitle>
<Box display="flex" alignItems="center">
<SeverityIcon severity={selectedAlert.severity} />
<Typography variant="h6" style={{ marginLeft: 8 }}>
{selectedAlert.type} Alert
</Typography>
</Box>
</DialogTitle>
<DialogContent>
<Typography variant="body1" gutterBottom>
{selectedAlert.message}
</Typography>
<Typography variant="subtitle1" gutterBottom style={{ marginTop: 16 }}>
Alert Details:
</Typography>
<TableContainer component={Paper}>
<Table size="small">
<TableBody>
<TableRow>
<TableCell><strong>Alert ID</strong></TableCell>
<TableCell>{selectedAlert.id}</TableCell>
</TableRow>
<TableRow>
<TableCell><strong>Severity</strong></TableCell>
<TableCell>{selectedAlert.severity}</TableCell>
</TableRow>
<TableRow>
<TableCell><strong>Time</strong></TableCell>
<TableCell>{moment(selectedAlert.timestamp).format('YYYY-MM-DD HH:mm:ss')}</TableCell>
</TableRow>
<TableRow>
<TableCell><strong>Status</strong></TableCell>
<TableCell><StatusChip status={selectedAlert.status} /></TableCell>
</TableRow>
{selectedAlert.data.userId && (
<TableRow>
<TableCell><strong>User</strong></TableCell>
<TableCell>
<Button
size="small"
variant="outlined"
onClick={() => {
handleUserClick(selectedAlert.data.userId);
handleCloseAlertDialog();
}}
>
View User Activity
</Button>
</TableCell>
</TableRow>
)}
</TableBody>
</Table>
</TableContainer>
<Typography variant="subtitle1" gutterBottom style={{ marginTop: 16 }}>
Raw Data:
</Typography>
<Paper style={{ padding: 16, backgroundColor: '#f5f5f5' }}>
<pre style={{ margin: 0, overflow: 'auto' }}>
{JSON.stringify(selectedAlert.data, null, 2)}
</pre>
</Paper>
</DialogContent>
<DialogActions>
{selectedAlert.status === 'open' && (
<Button color="primary">
Investigate
</Button>
)}
{selectedAlert.status === 'investigating' && (
<Button color="success">
Mark as Resolved
</Button>
)}
<Button onClick={handleCloseAlertDialog}>
Close
</Button>
</DialogActions>
</>
)}
</Dialog>
</Box>
);
};
export default SecurityDashboard;
This implementation provides a comprehensive security dashboard for your Tempo Labs application. It visualizes security metrics, displays alerts, and allows for detailed investigation of security events.
Best Practices for Audit Logging and Monitoring in Tempo Labs
To ensure your Tempo Labs applications maintain robust security through proper audit logging and monitoring, follow these best practices:
1. Implement Comprehensive Logging
- Log All Security-Relevant Events: Authentication attempts, authorization decisions, data modifications, administrative actions, and system changes should all be logged.
- Include Contextual Information: Each log entry should contain timestamp, user ID, IP address, action type, affected resource, and result.
- Standardize Log Formats: Use consistent formats across your application to facilitate log analysis and integration with security tools.
- Separate Security Logs: Keep security-relevant logs separate from general application logs to simplify analysis and reduce noise.
2. Ensure Log Security and Integrity
- Protect Logs from Tampering: Implement digital signatures or cryptographic hashing to detect log tampering.
- Encrypt Sensitive Log Data: Ensure logs containing sensitive information are encrypted both in transit and at rest.
- Implement Proper Access Controls: Only authorized personnel should have access to security logs.
- Establish Retention Policies: Define how long logs should be kept based on compliance requirements and investigation needs.
3. Set Up Effective Monitoring
- Implement Real-Time Alerting: Configure alerts for suspicious activities like multiple failed login attempts, unusual data access patterns, or API abuse.
- Use Anomaly Detection: Leverage machine learning to identify unusual patterns that might indicate security incidents.
- Monitor System Health: Track system performance metrics to detect potential denial-of-service attacks.
- Establish Baselines: Understand normal behavior patterns to better identify deviations that might indicate security issues.
4. Create Actionable Visualizations
- Build Security Dashboards: Develop dashboards that provide at-a-glance visibility into your security posture.
- Implement Drill-Down Capabilities: Allow security personnel to investigate specific events in detail.
- Visualize Trends Over Time: Track security metrics over time to identify patterns and improvements.
- Include Geographic Visualizations: Map access attempts to detect suspicious login locations.
5. Develop Incident Response Procedures
- Define Escalation Paths: Establish clear procedures for responding to different types of security alerts.
- Automate Initial Responses: Implement automated responses for common security incidents, such as temporarily blocking suspicious IP addresses.
- Document Investigation Steps: Create playbooks for investigating different types of security incidents.
- Conduct Regular Reviews: Periodically review logs and alerts to identify potential security improvements.
Conclusion
Audit logging and monitoring are critical components of a secure Tempo Labs application. By implementing the prompts and best practices outlined in this post, you can significantly enhance your application’s security posture and ensure you have the visibility needed to detect and respond to security incidents.
Remember that security is an ongoing process. Regularly review and update your logging and monitoring systems to address new threats and vulnerabilities. By making security a priority in your Tempo Labs vibe coding projects, you can build applications that are not only functional but also secure and trustworthy.
The next time you’re using Tempo Labs for vibe coding, take a moment to implement proper audit logging and monitoring. Your future self (and your security team) will thank you when you need to investigate a security incident or demonstrate compliance with security requirements.