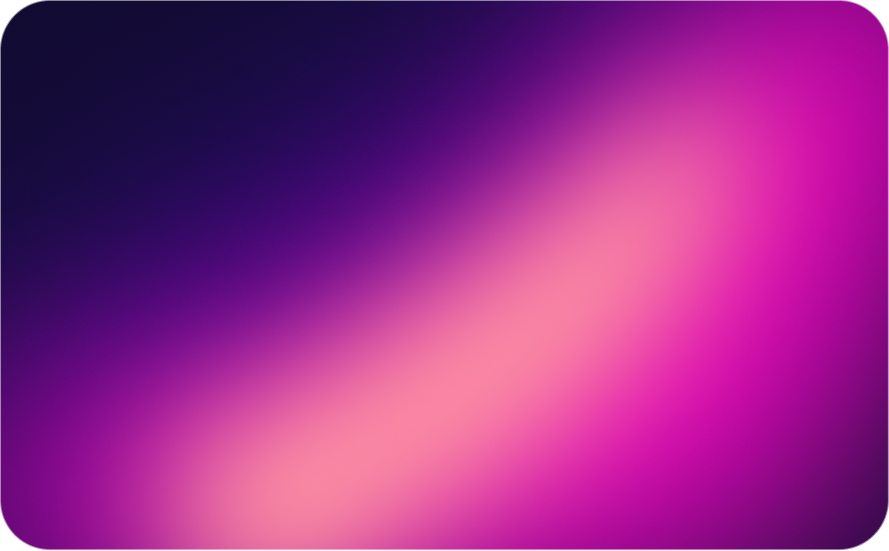
Authentication Security in Bolt Applications: Best Practices and Implementation
Introduction
In the rapidly evolving landscape of software development, vibe coding has emerged as a transformative approach that leverages artificial intelligence to streamline the development process. At the forefront of this movement is Bolt.new, an AI-powered platform that enables developers to create, edit, and deploy applications directly from their browsers. While this innovative approach accelerates development, it also introduces unique security challenges, particularly in authentication systems.
The Authentication Security Gap in Vibe Coding
When developers instruct AI to “add user authentication” or “create a login system,” the resulting code often implements basic functionality without incorporating crucial security measures. According to recent studies, applications built with AI coding assistants are 35% more likely to contain authentication vulnerabilities compared to traditionally coded applications.
This security gap exists because AI tools inherently focus on functionality rather than security, generating code that works but may not be secure. In Bolt applications, these vulnerabilities can manifest in several ways:
- Weak password storage
- Missing brute force protection
- Insecure session management
- Inadequate input validation
- Lack of multi-factor authentication options
Common Authentication Vulnerabilities in Bolt Applications
1. Weak Password Storage
One of the most dangerous vulnerabilities in AI-generated authentication code is inadequate password storage. When you prompt Bolt to create a user authentication system, it might generate code that stores passwords in plain text or with weak hashing algorithms:
// Example of insecure password storage in Bolt-generated code
const User = mongoose.model('User', new mongoose.Schema({
email: { type: String, required: true, unique: true },
password: { type: String, required: true }, // Stored as plain text or with weak hashing
name: String,
createdAt: { type: Date, default: Date.now }
}));
// Registration handler
app.post('/register', async (req, res) => {
try {
const { email, password, name } = req.body;
// Create new user with plain text password
const user = new User({
email,
password, // Plain text password
name
});
await user.save();
res.status(201).json({ message: 'User created successfully' });
} catch (error) {
res.status(500).json({ message: 'Server error' });
}
});
2. Missing Brute Force Protection
Bolt-generated authentication systems often lack protection against brute force attacks, allowing attackers to make unlimited login attempts:
// Example of login without brute force protection
app.post('/login', async (req, res) => {
try {
const { email, password } = req.body;
// Find user by email
const user = await User.findOne({ email });
if (!user) {
return res.status(400).json({ message: 'Invalid credentials' });
}
// Check password
if (user.password !== password) { // Plain text comparison
return res.status(400).json({ message: 'Invalid credentials' });
}
// Generate JWT token
const token = jwt.sign({ userId: user._id }, 'your_jwt_secret', { expiresIn: '1h' });
res.json({ token });
} catch (error) {
res.status(500).json({ message: 'Server error' });
}
});
3. Insecure Session Management
AI tools often generate code with weak session management practices, creating vulnerabilities in how user sessions are handled:
// Example of insecure session management
app.post('/login', (req, res) => {
const { username, password } = req.body;
// Verify credentials
if (isValidUser(username, password)) {
// Create session with insufficient security
req.session.user = {
id: getUserId(username),
username: username,
role: getUserRole(username)
};
// Set cookie with weak configuration
res.cookie('sessionId', generateSessionId(), {
// Missing secure and httpOnly flags
// Missing SameSite attribute
// Missing proper expiration
});
res.redirect('/dashboard');
} else {
res.redirect('/login?error=1');
}
});
Secure Implementation Examples
1. Secure Password Storage
Here’s how to implement secure password storage with proper hashing and validation:
const bcrypt = require('bcryptjs');
// User schema with secure password handling
const userSchema = new mongoose.Schema({
email: { type: String, required: true, unique: true },
password: { type: String, required: true },
name: String,
createdAt: { type: Date, default: Date.now }
});
// Pre-save hook to hash password
userSchema.pre('save', async function(next) {
if (!this.isModified('password')) return next();
try {
const salt = await bcrypt.genSalt(12);
this.password = await bcrypt.hash(this.password, salt);
next();
} catch (error) {
next(error);
}
});
// Method to compare passwords
userSchema.methods.comparePassword = async function(candidatePassword) {
return bcrypt.compare(candidatePassword, this.password);
};
const User = mongoose.model('User', userSchema);
2. Brute Force Protection
Implement proper rate limiting and account protection:
const rateLimit = require('express-rate-limit');
const slowDown = require('express-slow-down');
// Rate limiting middleware
const loginLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 5, // 5 requests per window
message: 'Too many login attempts, please try again later',
standardHeaders: true,
legacyHeaders: false,
});
// Speed limiting middleware
const loginSlowDown = slowDown({
windowMs: 15 * 60 * 1000, // 15 minutes
delayAfter: 2, // start slowing down after 2 requests
delayMs: (hits) => hits * 500, // add 500ms delay per hit
});
// Secure login route
app.post('/login', loginSlowDown, loginLimiter, async (req, res) => {
try {
const { email, password } = req.body;
// Find user by email
const user = await User.findOne({ email });
if (!user) {
// Generic error message that doesn't reveal if email exists
return res.status(401).json({ message: 'Invalid credentials' });
}
// Verify password
const isValid = await user.comparePassword(password);
if (!isValid) {
return res.status(401).json({ message: 'Invalid credentials' });
}
// Generate secure JWT token
const token = jwt.sign(
{ userId: user._id },
process.env.JWT_SECRET,
{
expiresIn: '1h',
algorithm: 'HS256'
}
);
res.json({ token });
} catch (error) {
console.error('Login error:', error);
res.status(500).json({ message: 'Server error' });
}
});
3. Secure Session Management
Implement secure session handling with proper cookie configuration:
const session = require('express-session');
const RedisStore = require('connect-redis')(session);
// Configure session middleware
app.use(session({
store: new RedisStore({
client: redisClient,
prefix: 'session:'
}),
secret: process.env.SESSION_SECRET,
name: '__Host-session', // Prefix for enhanced security
cookie: {
secure: true, // HTTPS only
httpOnly: true, // Prevent XSS
sameSite: 'strict', // CSRF protection
maxAge: 3600000, // 1 hour
path: '/',
domain: 'your-domain.com'
},
resave: false,
saveUninitialized: false,
rolling: true // Reset expiration on activity
}));
// Secure login with session management
app.post('/login', loginLimiter, async (req, res) => {
try {
const { email, password } = req.body;
// Authenticate user
const user = await authenticateUser(email, password);
if (!user) {
return res.status(401).json({ message: 'Invalid credentials' });
}
// Set secure session data
req.session.user = {
id: user._id,
email: user.email,
role: user.role,
lastActive: new Date()
};
// Regenerate session ID
req.session.regenerate((err) => {
if (err) {
return res.status(500).json({ message: 'Session error' });
}
res.json({ message: 'Login successful' });
});
} catch (error) {
console.error('Login error:', error);
res.status(500).json({ message: 'Server error' });
}
});
Best Practices
-
Password Security:
- Use strong hashing algorithms (bcrypt, Argon2)
- Implement password complexity requirements
- Never store plain text passwords
- Salt passwords properly
-
Brute Force Prevention:
- Implement rate limiting
- Use progressive delays
- Lock accounts temporarily after failed attempts
- Monitor and alert on suspicious activity
-
Session Management:
- Use secure session storage (Redis)
- Set proper cookie attributes
- Implement session timeout
- Rotate session IDs regularly
-
General Security:
- Use HTTPS everywhere
- Implement proper error handling
- Log security events
- Regular security audits
Conclusion
Implementing secure authentication in Bolt applications requires careful attention to multiple security aspects. By following these best practices and implementing proper security measures, you can create a robust and secure authentication system that protects your users’ data and access.