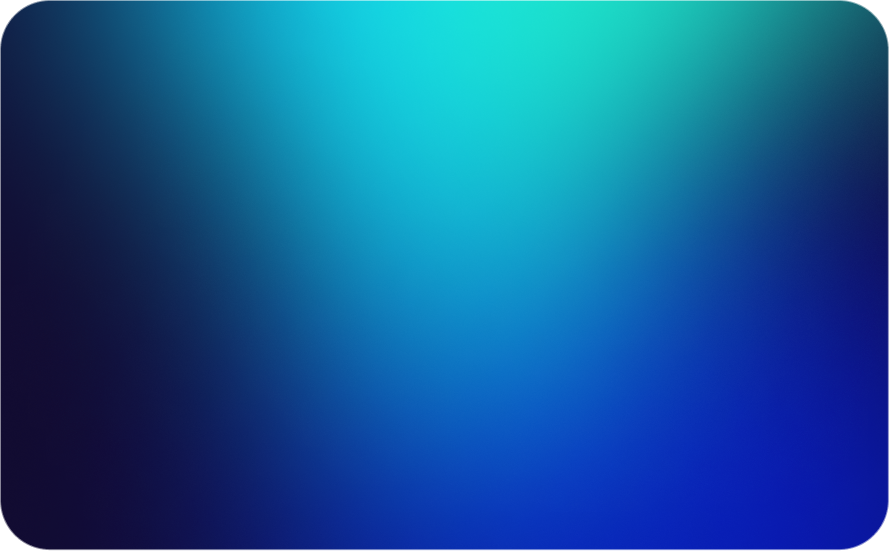
Cloud Storage Security: Best Practices and Implementation
Introduction
Cloud storage has become an integral part of modern applications, offering scalability, reliability, and cost-effectiveness. However, it also introduces unique security challenges that must be carefully addressed. This guide covers essential practices for implementing secure cloud storage, with a focus on AWS S3 and related services.
S3 Bucket Security
Understanding S3 Security
S3 bucket security involves multiple layers:
- Access control policies
- Encryption settings
- Logging and monitoring
- Network security
Implementing Secure S3 Configuration
const AWS = require('aws-sdk');
const crypto = require('crypto');
class SecureS3Storage {
constructor(config) {
this.s3 = new AWS.S3({
accessKeyId: config.accessKeyId,
secretAccessKey: config.secretAccessKey,
region: config.region,
// Force SSL/TLS
sslEnabled: true,
// Enforce encryption
s3ForcePathStyle: true
});
this.bucket = config.bucket;
this.defaultExpiry = 3600; // 1 hour
}
async uploadFile(file, key, options = {}) {
try {
// Generate server-side encryption key
const sseKey = crypto.randomBytes(32);
const sseKeyMd5 = crypto.createHash('md5')
.update(sseKey)
.digest('base64');
// Upload with encryption
const result = await this.s3.putObject({
Bucket: this.bucket,
Key: key,
Body: file,
// Server-side encryption
ServerSideEncryption: 'aws:kms',
SSEKMSKeyId: options.kmsKeyId,
// Object metadata
Metadata: {
'original-name': options.originalName || '',
'content-type': options.contentType || 'application/octet-stream',
'upload-date': new Date().toISOString()
},
// Security tags
Tagging: this.generateSecurityTags(options)
}).promise();
return {
key,
versionId: result.VersionId,
encryption: result.ServerSideEncryption
};
} catch (error) {
console.error('Upload error:', error);
throw new Error('Failed to upload file');
}
}
generateSecurityTags(options) {
const tags = [
`classification=${options.classification || 'private'}`,
`retention=${options.retention || 'standard'}`,
`owner=${options.owner || 'system'}`
];
return tags.join('&');
}
async configureSecureBucket() {
try {
// Enable versioning
await this.s3.putBucketVersioning({
Bucket: this.bucket,
VersioningConfiguration: {
Status: 'Enabled'
}
}).promise();
// Enable encryption
await this.s3.putBucketEncryption({
Bucket: this.bucket,
ServerSideEncryptionConfiguration: {
Rules: [{
ApplyServerSideEncryptionByDefault: {
SSEAlgorithm: 'aws:kms',
KMSMasterKeyID: process.env.KMS_KEY_ID
}
}]
}
}).promise();
// Configure public access block
await this.s3.putPublicAccessBlock({
Bucket: this.bucket,
PublicAccessBlockConfiguration: {
BlockPublicAcls: true,
IgnorePublicAcls: true,
BlockPublicPolicy: true,
RestrictPublicBuckets: true
}
}).promise();
// Enable logging
await this.s3.putBucketLogging({
Bucket: this.bucket,
BucketLoggingStatus: {
LoggingEnabled: {
TargetBucket: process.env.LOGGING_BUCKET,
TargetPrefix: `${this.bucket}/`
}
}
}).promise();
return true;
} catch (error) {
console.error('Bucket configuration error:', error);
throw error;
}
}
}
// Example usage
const s3Storage = new SecureS3Storage({
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY,
region: process.env.AWS_REGION,
bucket: process.env.S3_BUCKET
});
app.post('/upload', upload.single('file'), async (req, res) => {
try {
const file = req.file;
if (!file) {
return res.status(400).json({ error: 'No file uploaded' });
}
// Generate secure key
const key = `uploads/${Date.now()}-${crypto.randomBytes(16).toString('hex')}`;
// Upload to S3
const result = await s3Storage.uploadFile(file.buffer, key, {
originalName: file.originalname,
contentType: file.mimetype,
classification: 'confidential',
retention: '7-years',
owner: req.user.id
});
res.json(result);
} catch (error) {
console.error('Upload error:', error);
res.status(500).json({ error: 'Upload failed' });
}
});
S3 Security Best Practices
-
Access control:
- Use IAM roles
- Implement least privilege
- Regular policy review
- Enable versioning
-
Encryption:
- Server-side encryption
- Client-side encryption
- Key management
- Rotation policies
-
Monitoring:
- Enable logging
- Set up alerts
- Regular audits
- Compliance checks
Signed URLs
Implementing Secure URL Generation
class SecureUrlGenerator {
constructor(s3Client, config) {
this.s3 = s3Client;
this.bucket = config.bucket;
this.defaultExpiry = config.defaultExpiry || 3600;
}
async generateSignedUrl(key, options = {}) {
try {
const params = {
Bucket: this.bucket,
Key: key,
Expires: options.expiry || this.defaultExpiry
};
// Add additional security headers
if (options.contentDisposition) {
params.ResponseContentDisposition = options.contentDisposition;
}
if (options.contentType) {
params.ResponseContentType = options.contentType;
}
// Generate signed URL
const signedUrl = await this.s3.getSignedUrlPromise('getObject', params);
// Log URL generation
await this.logUrlGeneration(key, options);
return signedUrl;
} catch (error) {
console.error('URL generation error:', error);
throw error;
}
}
async generateUploadUrl(key, options = {}) {
try {
const params = {
Bucket: this.bucket,
Key: key,
Expires: options.expiry || this.defaultExpiry,
ContentType: options.contentType,
// Enforce server-side encryption
ServerSideEncryption: 'aws:kms',
SSEKMSKeyId: options.kmsKeyId
};
// Generate upload URL
const uploadUrl = await this.s3.getSignedUrlPromise('putObject', params);
return {
url: uploadUrl,
fields: {
key,
'Content-Type': options.contentType,
'x-amz-server-side-encryption': 'aws:kms',
'x-amz-server-side-encryption-aws-kms-key-id': options.kmsKeyId
}
};
} catch (error) {
console.error('Upload URL generation error:', error);
throw error;
}
}
async logUrlGeneration(key, options) {
await saveAuditLog({
type: 'signed_url_generated',
key,
expiry: options.expiry || this.defaultExpiry,
userId: options.userId,
timestamp: new Date()
});
}
}
// Example usage
const urlGenerator = new SecureUrlGenerator(s3Storage.s3, {
bucket: process.env.S3_BUCKET,
defaultExpiry: 3600
});
app.get('/files/:key/download-url', async (req, res) => {
try {
const key = req.params.key;
// Generate download URL
const signedUrl = await urlGenerator.generateSignedUrl(key, {
expiry: 3600,
contentDisposition: 'attachment',
userId: req.user.id
});
res.json({ url: signedUrl });
} catch (error) {
console.error('URL generation error:', error);
res.status(500).json({ error: 'Failed to generate URL' });
}
});
Signed URL Best Practices
-
URL configuration:
- Short expiration times
- Enforce HTTPS
- Include security headers
- Validate parameters
-
Access control:
- User authentication
- IP restrictions
- Rate limiting
- Usage tracking
-
Security headers:
- Content disposition
- Content type
- Cache control
- Custom metadata
CDN Security
Implementing Secure CDN
const CloudFront = require('aws-sdk/clients/cloudfront');
class SecureCDN {
constructor(config) {
this.cloudfront = new CloudFront({
accessKeyId: config.accessKeyId,
secretAccessKey: config.secretAccessKey,
region: config.region
});
this.distribution = config.distributionId;
this.keyPairId = config.keyPairId;
this.privateKey = config.privateKey;
}
async generateSecureUrl(path, options = {}) {
try {
const signer = new CloudFront.Signer(
this.keyPairId,
this.privateKey
);
// Generate signed URL
const signedUrl = signer.getSignedUrl({
url: `https://${options.domain}/${path}`,
expires: Math.floor(Date.now() / 1000) + (options.expiry || 3600)
});
return signedUrl;
} catch (error) {
console.error('CDN URL generation error:', error);
throw error;
}
}
async invalidateCache(paths) {
try {
await this.cloudfront.createInvalidation({
DistributionId: this.distribution,
InvalidationBatch: {
CallerReference: Date.now().toString(),
Paths: {
Quantity: paths.length,
Items: paths
}
}
}).promise();
return true;
} catch (error) {
console.error('Cache invalidation error:', error);
throw error;
}
}
async configureSecurity() {
try {
await this.cloudfront.updateDistribution({
Id: this.distribution,
DistributionConfig: {
// Enable HTTPS only
ViewerCertificate: {
CloudFrontDefaultCertificate: false,
ACMCertificateArn: process.env.ACM_CERT_ARN,
SSLSupportMethod: 'sni-only',
MinimumProtocolVersion: 'TLSv1.2_2021'
},
// Configure security headers
DefaultCacheBehavior: {
ViewerProtocolPolicy: 'https-only',
AllowedMethods: {
Quantity: 2,
Items: ['GET', 'HEAD']
},
CachedMethods: {
Quantity: 2,
Items: ['GET', 'HEAD']
},
ForwardedValues: {
QueryString: true,
Cookies: {
Forward: 'none'
},
Headers: {
Quantity: 1,
Items: ['Origin']
}
},
MinTTL: 0,
DefaultTTL: 86400,
MaxTTL: 31536000,
Compress: true
}
}
}).promise();
return true;
} catch (error) {
console.error('CDN configuration error:', error);
throw error;
}
}
}
// Example usage
const cdn = new SecureCDN({
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY,
region: process.env.AWS_REGION,
distributionId: process.env.CLOUDFRONT_DISTRIBUTION_ID,
keyPairId: process.env.CLOUDFRONT_KEY_PAIR_ID,
privateKey: process.env.CLOUDFRONT_PRIVATE_KEY
});
app.get('/cdn-url/:path', async (req, res) => {
try {
const path = req.params.path;
// Generate CDN URL
const url = await cdn.generateSecureUrl(path, {
domain: process.env.CDN_DOMAIN,
expiry: 3600
});
res.json({ url });
} catch (error) {
console.error('CDN URL error:', error);
res.status(500).json({ error: 'Failed to generate CDN URL' });
}
});
CDN Security Best Practices
-
HTTPS configuration:
- Force HTTPS
- Modern TLS versions
- Custom certificates
- HSTS implementation
-
Access control:
- Signed URLs
- Geographic restrictions
- Referrer checking
- Custom headers
-
Cache security:
- Cache invalidation
- TTL settings
- Private content
- Header forwarding
Cross-Origin Resource Sharing
Implementing CORS Security
class CORSManager {
constructor(s3Client, config) {
this.s3 = s3Client;
this.bucket = config.bucket;
this.allowedOrigins = config.allowedOrigins;
}
async configureCORS() {
try {
await this.s3.putBucketCors({
Bucket: this.bucket,
CORSConfiguration: {
CORSRules: [{
AllowedHeaders: [
'Authorization',
'Content-Type',
'x-amz-date',
'x-amz-security-token'
],
AllowedMethods: ['GET', 'PUT', 'POST', 'DELETE'],
AllowedOrigins: this.allowedOrigins,
ExposeHeaders: [
'ETag',
'x-amz-server-side-encryption',
'x-amz-request-id',
'x-amz-id-2'
],
MaxAgeSeconds: 3600
}]
}
}).promise();
return true;
} catch (error) {
console.error('CORS configuration error:', error);
throw error;
}
}
validateOrigin(origin) {
return this.allowedOrigins.some(allowed => {
if (allowed === '*') return true;
if (allowed instanceof RegExp) return allowed.test(origin);
return allowed === origin;
});
}
}
// Example usage
const corsManager = new CORSManager(s3Storage.s3, {
bucket: process.env.S3_BUCKET,
allowedOrigins: [
process.env.APP_ORIGIN,
/\.trusted-domain\.com$/
]
});
// Configure Express CORS
app.use((req, res, next) => {
const origin = req.headers.origin;
if (corsManager.validateOrigin(origin)) {
res.setHeader('Access-Control-Allow-Origin', origin);
res.setHeader('Access-Control-Allow-Methods', 'GET,PUT,POST,DELETE');
res.setHeader('Access-Control-Allow-Headers', 'Content-Type,Authorization');
res.setHeader('Access-Control-Expose-Headers', 'ETag');
res.setHeader('Access-Control-Max-Age', '3600');
}
next();
});
CORS Best Practices
-
Origin validation:
- Whitelist approach
- Domain patterns
- Subdomain handling
- Regular updates
-
Header control:
- Minimal exposure
- Required headers only
- Secure defaults
- Regular review
-
Method restrictions:
- Limit methods
- Validate requests
- Handle preflight
- Monitor usage
Conclusion
Implementing secure cloud storage requires careful attention to multiple security aspects. By properly implementing S3 bucket security, signed URLs, CDN security, and CORS, you create a comprehensive security strategy for cloud storage.
Key takeaways:
- Secure S3 bucket configuration
- Implement signed URLs
- Configure CDN security
- Manage CORS properly
- Monitor and audit regularly