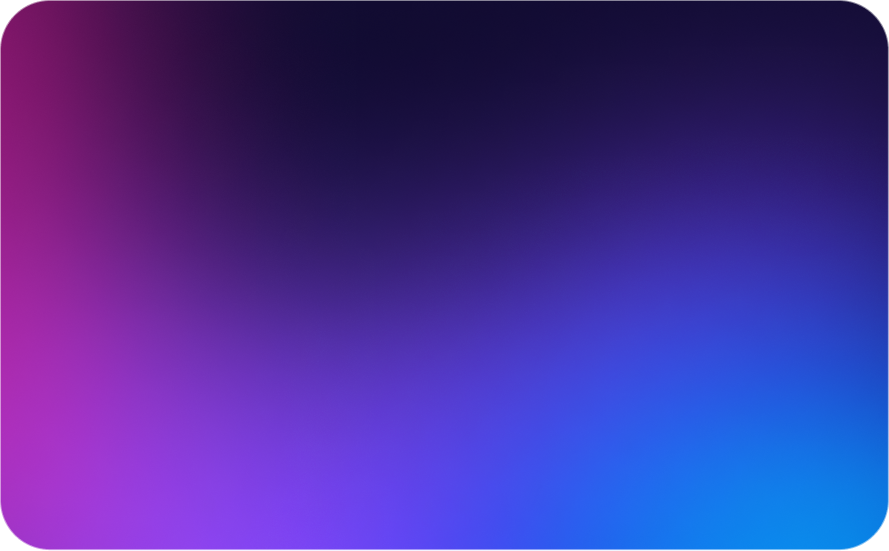
Data Encryption in Lovable: Best Practices and Implementation
Introduction
In the rapidly evolving landscape of vibe coding, Lovable has emerged as a powerful platform that enables developers to create applications through natural language prompts. While this approach dramatically accelerates development, it also introduces unique security challenges, particularly when it comes to protecting sensitive data. Proper encryption is not just a best practice—it’s essential for safeguarding user information and maintaining compliance with data protection regulations.
According to recent studies, applications built with AI coding assistants are more likely to contain encryption vulnerabilities compared to traditionally coded applications. This is because AI tools typically focus on functionality rather than security, generating code that works but may not adequately protect data at rest or in transit.
Common Encryption Vulnerabilities
1. Unencrypted Data Storage
One of the most common vulnerabilities in Lovable-generated applications is inadequate encryption of stored data:
// Example of insecure data storage
const userSchema = new Schema({
name: String,
email: String,
creditCardNumber: String, // Stored as plain text
address: String,
phoneNumber: String
});
app.post('/users', async (req, res) => {
try {
const { name, email, creditCardNumber, address, phoneNumber } = req.body;
const newUser = new User({
name,
email,
creditCardNumber, // Storing sensitive data as plain text
address,
phoneNumber
});
await newUser.save();
res.status(201).json({ message: 'User created successfully' });
} catch (error) {
res.status(500).json({ message: 'Server error' });
}
});
2. Insecure Data Transmission
Many applications lack proper encryption for data in transit:
// Example of insecure data transmission
const app = express();
app.listen(3000, () => {
console.log('Server running on port 3000');
});
// Client-side API call without TLS
fetch('http://api.example.com/users', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({
name: 'John Doe',
creditCardNumber: '1234-5678-9012-3456'
})
});
3. Hardcoded Encryption Keys
A critical vulnerability is the use of hardcoded encryption keys:
// Example of hardcoded encryption keys
const crypto = require('crypto');
const encryptionKey = 'mySecretKey12345'; // Hardcoded key
const iv = 'myInitVector123'; // Hardcoded IV
function encryptData(data) {
const cipher = crypto.createCipheriv('aes-256-cbc', encryptionKey, iv);
let encrypted = cipher.update(data, 'utf8', 'hex');
encrypted += cipher.final('hex');
return encrypted;
}
Implementing Secure Encryption
1. Field-Level Encryption
Here’s how to implement secure field-level encryption:
const crypto = require('crypto');
require('dotenv').config();
class FieldEncryption {
constructor() {
this.key = Buffer.from(process.env.ENCRYPTION_KEY, 'hex');
this.algorithm = 'aes-256-gcm';
this.ivLength = 16;
}
encrypt(text) {
const iv = crypto.randomBytes(this.ivLength);
const cipher = crypto.createCipheriv(this.algorithm, this.key, iv);
let encrypted = cipher.update(text, 'utf8', 'hex');
encrypted += cipher.final('hex');
const authTag = cipher.getAuthTag().toString('hex');
return {
iv: iv.toString('hex'),
encryptedData: encrypted,
authTag
};
}
decrypt(encryptedObj) {
const iv = Buffer.from(encryptedObj.iv, 'hex');
const authTag = Buffer.from(encryptedObj.authTag, 'hex');
const decipher = crypto.createDecipheriv(this.algorithm, this.key, iv);
decipher.setAuthTag(authTag);
let decrypted = decipher.update(encryptedObj.encryptedData, 'hex', 'utf8');
decrypted += decipher.final('utf8');
return decrypted;
}
}
// Usage in schema
const userSchema = new Schema({
name: String,
email: String,
creditCardNumber: {
iv: String,
encryptedData: String,
authTag: String
}
});
// Middleware to handle encryption/decryption
const fieldEncryption = new FieldEncryption();
userSchema.pre('save', function(next) {
if (this.isModified('creditCardNumber')) {
const encrypted = fieldEncryption.encrypt(this.creditCardNumber);
this.creditCardNumber = encrypted;
}
next();
});
userSchema.methods.getDecryptedCard = function() {
return fieldEncryption.decrypt(this.creditCardNumber);
};
2. Secure Key Management
Implement proper key management using a key management service:
class KeyManager {
constructor() {
this.kms = new AWS.KMS({
region: process.env.AWS_REGION,
accessKeyId: process.env.AWS_ACCESS_KEY_ID,
secretAccessKey: process.env.AWS_SECRET_ACCESS_KEY
});
}
async generateDataKey() {
const params = {
KeyId: process.env.KMS_KEY_ID,
KeySpec: 'AES_256'
};
const { Plaintext, CiphertextBlob } = await this.kms
.generateDataKey(params)
.promise();
return {
dataKey: Plaintext,
encryptedDataKey: CiphertextBlob
};
}
async decryptDataKey(encryptedDataKey) {
const params = {
CiphertextBlob: encryptedDataKey
};
const { Plaintext } = await this.kms
.decrypt(params)
.promise();
return Plaintext;
}
}
// Usage example
const keyManager = new KeyManager();
async function encryptUserData(userData) {
const { dataKey, encryptedDataKey } = await keyManager.generateDataKey();
const cipher = crypto.createCipheriv('aes-256-gcm', dataKey, iv);
let encrypted = cipher.update(JSON.stringify(userData), 'utf8', 'hex');
encrypted += cipher.final('hex');
return {
encryptedData: encrypted,
encryptedDataKey
};
}
3. Secure Data Transmission
Implement secure data transmission with proper TLS configuration:
const https = require('https');
const fs = require('fs');
const options = {
key: fs.readFileSync('private-key.pem'),
cert: fs.readFileSync('certificate.pem'),
ciphers: [
'TLS_AES_128_GCM_SHA256',
'TLS_AES_256_GCM_SHA384',
'TLS_CHACHA20_POLY1305_SHA256'
].join(':'),
minVersion: 'TLSv1.2',
honorCipherOrder: true
};
const app = express();
const server = https.createServer(options, app);
// Client-side secure fetch
async function secureApiCall(endpoint, data) {
const response = await fetch(endpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'X-Content-Type-Options': 'nosniff'
},
body: JSON.stringify(data),
credentials: 'include',
mode: 'cors'
});
return response.json();
}
Best Practices
-
Key Management:
- Use a key management service (KMS)
- Implement key rotation
- Never store keys in code
- Use envelope encryption
-
Data Classification:
- Identify sensitive data
- Apply appropriate encryption levels
- Document data handling procedures
- Regular security audits
-
Encryption Implementation:
- Use strong algorithms (AES-256-GCM)
- Implement proper IV handling
- Secure key storage
- Regular security updates
-
Transport Security:
- Use TLS 1.2 or higher
- Configure secure ciphers
- Implement certificate pinning
- Regular security scans
Conclusion
Implementing proper encryption in Lovable applications is crucial for protecting sensitive data. By following these best practices and implementing secure encryption patterns, you can ensure that your applications maintain both the development efficiency that Lovable provides and the security that modern applications require.