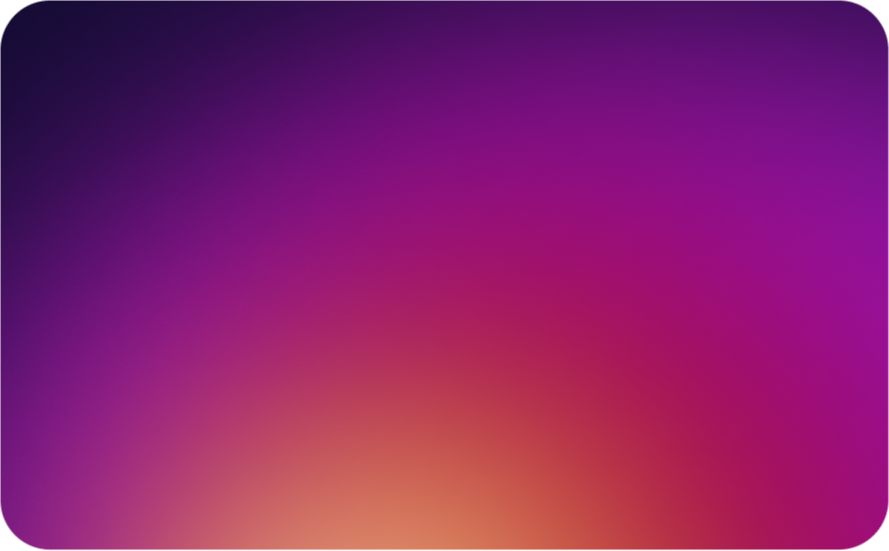
File Processing Security: Best Practices and Implementation
Introduction
Secure file processing is a critical aspect of file handling systems. When processing files, applications must handle various security concerns including memory management, resource allocation, and proper cleanup. This guide covers essential practices for implementing secure file processing.
Asynchronous Processing
Why Async Processing?
Asynchronous processing provides several benefits:
- Improved scalability
- Better resource utilization
- Enhanced user experience
- Reduced server load
Implementing Async Processing
const Queue = require('bull');
const fs = require('fs').promises;
const path = require('path');
class FileProcessor {
constructor() {
this.processQueue = new Queue('file-processing', {
redis: {
host: process.env.REDIS_HOST,
port: process.env.REDIS_PORT,
password: process.env.REDIS_PASSWORD
}
});
this.setupQueueHandlers();
}
setupQueueHandlers() {
this.processQueue.on('error', this.handleQueueError.bind(this));
this.processQueue.on('failed', async (job, error) => {
await this.handleProcessingFailure(job, error);
});
this.processQueue.process(async (job) => {
return this.processFile(job.data);
});
}
async queueFileProcessing(fileData) {
const job = await this.processQueue.add(fileData, {
attempts: 3,
backoff: {
type: 'exponential',
delay: 1000
},
removeOnComplete: true,
timeout: 300000 // 5 minutes
});
return job.id;
}
async processFile(fileData) {
try {
// Update processing status
await this.updateProcessingStatus(fileData.id, 'processing');
// Verify file exists
await this.verifyFile(fileData.path);
// Process file in chunks
await this.processFileInChunks(fileData);
// Update status to complete
await this.updateProcessingStatus(fileData.id, 'completed');
return { success: true };
} catch (error) {
console.error('Processing error:', error);
await this.updateProcessingStatus(fileData.id, 'failed');
throw error;
}
}
async processFileInChunks(fileData) {
const chunkSize = 1024 * 1024; // 1MB chunks
const fileHandle = await fs.open(fileData.path, 'r');
const stats = await fileHandle.stat();
try {
let bytesProcessed = 0;
const buffer = Buffer.alloc(chunkSize);
while (bytesProcessed < stats.size) {
const { bytesRead } = await fileHandle.read(
buffer,
0,
chunkSize,
bytesProcessed
);
if (bytesRead === 0) break;
// Process chunk
await this.processChunk(buffer.slice(0, bytesRead));
bytesProcessed += bytesRead;
// Update progress
const progress = Math.round((bytesProcessed / stats.size) * 100);
await this.updateProgress(fileData.id, progress);
}
} finally {
await fileHandle.close();
}
}
async handleQueueError(error) {
console.error('Queue error:', error);
// Implement error notification system
}
async handleProcessingFailure(job, error) {
console.error(`Job ${job.id} failed:`, error);
// Update processing status
await this.updateProcessingStatus(job.data.id, 'failed');
// Cleanup temporary files
await this.cleanupTempFiles(job.data);
}
}
// Example usage
const processor = new FileProcessor();
app.post('/process-file', async (req, res) => {
try {
const fileData = {
id: req.body.fileId,
path: req.body.filePath,
type: req.body.fileType
};
const jobId = await processor.queueFileProcessing(fileData);
res.json({
jobId,
message: 'File processing queued'
});
} catch (error) {
console.error('Queue error:', error);
res.status(500).json({ error: 'Failed to queue processing' });
}
});
Async Processing Best Practices
-
Queue management:
- Handle retries properly
- Implement backoff strategies
- Monitor queue health
- Clean up completed jobs
-
Progress tracking:
- Real-time updates
- Accurate progress calculation
- Error state handling
- User notifications
-
Resource management:
- Limit concurrent processing
- Monitor system resources
- Implement circuit breakers
- Handle cleanup properly
Secure File Deletion
Implementing Secure Deletion
const fs = require('fs').promises;
const crypto = require('crypto');
class SecureFileDeleter {
constructor() {
this.passes = 3; // Number of overwrite passes
}
async secureDelete(filePath) {
try {
// Get file size
const stats = await fs.stat(filePath);
const fileSize = stats.size;
// Perform multiple overwrite passes
for (let i = 0; i < this.passes; i++) {
await this.overwriteFile(filePath, fileSize);
}
// Delete the file
await fs.unlink(filePath);
return true;
} catch (error) {
console.error('Secure delete error:', error);
throw error;
}
}
async overwriteFile(filePath, size) {
const handle = await fs.open(filePath, 'r+');
try {
const buffer = Buffer.alloc(64 * 1024); // 64KB chunks
let position = 0;
while (position < size) {
// Fill buffer with random data
crypto.randomFillSync(buffer);
const writeSize = Math.min(buffer.length, size - position);
await handle.write(buffer, 0, writeSize, position);
position += writeSize;
}
// Flush changes to disk
await handle.sync();
} finally {
await handle.close();
}
}
async secureDeleteDirectory(dirPath) {
try {
const entries = await fs.readdir(dirPath, { withFileTypes: true });
// Process all entries
for (const entry of entries) {
const fullPath = path.join(dirPath, entry.name);
if (entry.isDirectory()) {
await this.secureDeleteDirectory(fullPath);
} else {
await this.secureDelete(fullPath);
}
}
// Remove empty directory
await fs.rmdir(dirPath);
return true;
} catch (error) {
console.error('Directory deletion error:', error);
throw error;
}
}
}
// Example usage
const deleter = new SecureFileDeleter();
app.delete('/files/:fileId', async (req, res) => {
try {
const fileId = req.params.fileId;
const fileMetadata = await getFileMetadata(fileId);
if (!fileMetadata) {
return res.status(404).json({ error: 'File not found' });
}
// Securely delete file
await deleter.secureDelete(fileMetadata.path);
// Remove metadata
await deleteFileMetadata(fileId);
res.json({ message: 'File securely deleted' });
} catch (error) {
console.error('Delete error:', error);
res.status(500).json({ error: 'Failed to delete file' });
}
});
Secure Deletion Best Practices
-
Overwrite strategies:
- Multiple passes
- Random data
- Full file coverage
- Verify deletion
-
Metadata cleanup:
- Remove all references
- Clean up databases
- Update indexes
- Remove cached data
-
Error handling:
- Verify deletion success
- Handle partial failures
- Maintain audit logs
- Cleanup remaining data
Memory Management
Implementing Memory Controls
const v8 = require('v8');
const stream = require('stream');
const util = require('util');
class MemoryManager {
constructor(options = {}) {
this.maxMemoryUsage = options.maxMemoryUsage || 1024 * 1024 * 1024; // 1GB
this.warningThreshold = options.warningThreshold || 0.8; // 80%
this.setupMemoryMonitoring();
}
setupMemoryMonitoring() {
// Monitor memory usage periodically
setInterval(() => {
this.checkMemoryUsage();
}, 30000); // Every 30 seconds
}
checkMemoryUsage() {
const memoryUsage = process.memoryUsage();
const heapUsed = memoryUsage.heapUsed;
if (heapUsed > this.maxMemoryUsage * this.warningThreshold) {
console.warn('Memory usage warning:', {
used: heapUsed,
max: this.maxMemoryUsage,
percentage: (heapUsed / this.maxMemoryUsage) * 100
});
// Force garbage collection if available
if (global.gc) {
global.gc();
}
}
}
async processLargeFile(inputPath, outputPath, processor) {
const pipeline = util.promisify(stream.pipeline);
try {
await pipeline(
fs.createReadStream(inputPath, {
highWaterMark: 64 * 1024 // 64KB chunks
}),
new stream.Transform({
transform: async function(chunk, encoding, callback) {
try {
// Process chunk with limited memory
const result = await processor(chunk);
callback(null, result);
} catch (error) {
callback(error);
}
}
}),
fs.createWriteStream(outputPath)
);
return true;
} catch (error) {
console.error('Processing error:', error);
throw error;
}
}
getMemoryStats() {
const memoryUsage = process.memoryUsage();
const heapStats = v8.getHeapStatistics();
return {
heapUsed: memoryUsage.heapUsed,
heapTotal: memoryUsage.heapTotal,
external: memoryUsage.external,
heapSizeLimit: heapStats.heap_size_limit,
totalAvailable: heapStats.total_available_size
};
}
}
// Example usage
const memoryManager = new MemoryManager({
maxMemoryUsage: 2 * 1024 * 1024 * 1024, // 2GB
warningThreshold: 0.7 // 70%
});
app.post('/process-large-file', async (req, res) => {
try {
const { inputPath, outputPath } = req.body;
// Process file with memory management
await memoryManager.processLargeFile(
inputPath,
outputPath,
async (chunk) => {
// Process chunk with memory-efficient operations
return chunk;
}
);
res.json({ message: 'File processed successfully' });
} catch (error) {
console.error('Processing error:', error);
res.status(500).json({ error: 'Processing failed' });
}
});
Memory Management Best Practices
-
Resource monitoring:
- Track memory usage
- Set usage limits
- Monitor leaks
- Implement warnings
-
Efficient processing:
- Use streams
- Process in chunks
- Clean up resources
- Optimize algorithms
-
Error prevention:
- Validate input sizes
- Handle out-of-memory
- Implement timeouts
- Monitor performance
Processing Timeouts
Implementing Timeout Controls
class ProcessingTimeoutManager {
constructor(options = {}) {
this.defaultTimeout = options.defaultTimeout || 300000; // 5 minutes
this.maxTimeout = options.maxTimeout || 3600000; // 1 hour
}
async executeWithTimeout(operation, timeout = this.defaultTimeout) {
// Ensure timeout is within limits
const actualTimeout = Math.min(timeout, this.maxTimeout);
const timeoutPromise = new Promise((_, reject) => {
setTimeout(() => {
reject(new Error('Operation timed out'));
}, actualTimeout);
});
try {
// Race between operation and timeout
return await Promise.race([
operation(),
timeoutPromise
]);
} catch (error) {
if (error.message === 'Operation timed out') {
await this.handleTimeout();
}
throw error;
}
}
async handleTimeout() {
// Implement cleanup and logging
console.error('Processing timeout occurred');
}
calculateTimeout(fileSize) {
// Calculate timeout based on file size
const baseTimeout = 60000; // 1 minute base
const timeoutPerMB = 1000; // 1 second per MB
const timeout = baseTimeout + (fileSize / (1024 * 1024)) * timeoutPerMB;
return Math.min(timeout, this.maxTimeout);
}
}
// Example usage
const timeoutManager = new ProcessingTimeoutManager({
defaultTimeout: 300000, // 5 minutes
maxTimeout: 3600000 // 1 hour
});
app.post('/process-with-timeout', async (req, res) => {
try {
const { fileSize } = req.body;
// Calculate appropriate timeout
const timeout = timeoutManager.calculateTimeout(fileSize);
// Execute with timeout
const result = await timeoutManager.executeWithTimeout(
async () => {
// Perform processing
return { success: true };
},
timeout
);
res.json(result);
} catch (error) {
console.error('Processing error:', error);
res.status(500).json({ error: 'Processing failed' });
}
});
Timeout Best Practices
-
Timeout configuration:
- Set appropriate limits
- Scale with file size
- Consider resources
- Monitor patterns
-
Cleanup handling:
- Resource release
- State cleanup
- Error notification
- Recovery procedures
-
User experience:
- Progress updates
- Clear messaging
- Retry options
- Status tracking
Conclusion
Implementing secure file processing requires careful attention to various aspects including asynchronous processing, secure deletion, memory management, and timeout controls. By following these best practices and implementing proper security measures, you can create a robust and secure file processing system.
Key takeaways:
- Use asynchronous processing
- Implement secure deletion
- Manage memory efficiently
- Control processing timeouts
- Monitor system resources