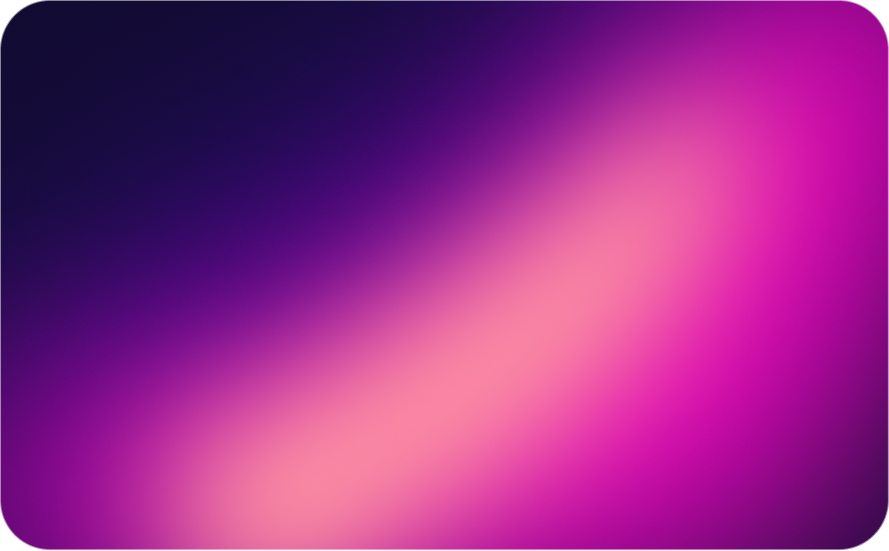
File Upload Security Fundamentals: A Comprehensive Guide
Introduction
File upload functionality is a critical component of modern web applications, enabling users to share documents, images, videos, and other content. However, this seemingly simple feature can introduce significant security vulnerabilities if not implemented correctly. According to recent studies, file upload vulnerabilities are present in 68% of applications built with AI coding assistants, making it crucial to understand and implement proper security measures.
File Size Limitations
Why Size Matters
Unrestricted file sizes can lead to several security issues:
- Denial of Service (DoS) attacks through storage exhaustion
- Memory consumption during file processing
- Bandwidth exhaustion during upload/download
- Performance degradation
Implementing Size Restrictions
const multer = require('multer');
const upload = multer({
storage: multer.diskStorage({
destination: './temp-uploads',
filename: (req, file, cb) => {
const tempFilename = `${Date.now()}-${Math.round(Math.random() * 1E9)}`;
cb(null, tempFilename);
}
}),
limits: {
fileSize: 5 * 1024 * 1024, // 5MB limit
files: 1 // Only allow one file per request
}
});
app.post('/upload', (req, res) => {
upload.single('file')(req, res, function(err) {
if (err instanceof multer.MulterError) {
if (err.code === 'LIMIT_FILE_SIZE') {
return res.status(400).json({ error: 'File too large (max: 5MB)' });
}
return res.status(400).json({ error: 'Upload error' });
}
// Continue with file processing...
});
});
Best Practices for Size Limitations
-
Set appropriate size limits based on:
- Application requirements
- Server resources
- User needs
- Storage capacity
-
Implement client-side validation:
- Provide immediate feedback
- Reduce server load
- Improve user experience
-
Always maintain server-side validation:
- Never trust client-side validation alone
- Handle validation errors gracefully
- Clean up temporary files
MIME Type Validation
Understanding MIME Types
MIME (Multipurpose Internet Mail Extensions) types identify file formats and content types. However, relying solely on MIME type headers is insufficient for security:
- MIME types can be spoofed
- File extensions can be manipulated
- Content-Type headers can be modified
Secure MIME Type Validation
const fileTypeChecker = require('file-type-checker');
async function validateFileType(filePath, allowedTypes) {
try {
// Check file signature (magic bytes)
const fileType = await fileTypeChecker.detectFile(filePath);
if (!fileType) {
return { valid: false, reason: 'Could not determine file type' };
}
if (!allowedTypes.includes(fileType.mime)) {
return { valid: false, reason: 'File type not allowed' };
}
return { valid: true, type: fileType.mime };
} catch (error) {
return { valid: false, reason: 'File type validation failed' };
}
}
// Example usage
app.post('/upload', upload.single('file'), async (req, res) => {
const file = req.file;
if (!file) {
return res.status(400).json({ error: 'No file uploaded' });
}
const allowedTypes = ['image/jpeg', 'image/png', 'image/gif'];
const validation = await validateFileType(file.path, allowedTypes);
if (!validation.valid) {
await fs.promises.unlink(file.path);
return res.status(400).json({ error: validation.reason });
}
// Continue with secure file processing...
});
MIME Type Validation Best Practices
-
Use file signature detection:
- Check magic bytes
- Don’t trust file extensions
- Validate actual content
-
Implement whitelist approach:
- Define allowed file types
- Reject unknown types
- Be specific with formats
-
Handle validation failures:
- Remove invalid files
- Log validation errors
- Provide clear error messages
File Extension Validation
Common Extension Attacks
File extension manipulation can lead to:
- Code execution vulnerabilities
- Server-side script injection
- Content type misinterpretation
- Security control bypass
Secure Extension Validation
function validateFileExtension(originalFilename, allowedExtensions) {
// Get the last extension
const extension = originalFilename.split('.').pop().toLowerCase();
// Check if extension is allowed
if (!allowedExtensions.includes(extension)) {
return { valid: false, reason: 'File extension not allowed' };
}
// Check for double extensions
const parts = originalFilename.toLowerCase().split('.');
if (parts.length > 2) {
return { valid: false, reason: 'Multiple extensions not allowed' };
}
return { valid: true, extension };
}
// Example usage
const allowedExtensions = ['jpg', 'jpeg', 'png', 'gif'];
const validation = validateFileExtension(file.originalname, allowedExtensions);
if (!validation.valid) {
return res.status(400).json({ error: validation.reason });
}
Extension Validation Best Practices
-
Use whitelist approach:
- Define allowed extensions
- Be specific and restrictive
- Consider business requirements
-
Handle multiple extensions:
- Prevent double extension attacks
- Normalize extensions
- Consider security implications
-
Combine with MIME type validation:
- Cross-validate extensions
- Ensure consistency
- Reject mismatches
Antivirus Scanning
Importance of Malware Detection
File uploads can be used to distribute:
- Malware
- Viruses
- Ransomware
- Other malicious content
Implementing Antivirus Scanning
const NodeClam = require('clamscan');
const ClamScan = new NodeClam().init({
removeInfected: true,
quarantineInfected: false,
scanLog: null,
debugMode: false,
fileList: null,
scanRecursively: true,
clamscan: {
path: '/usr/bin/clamscan',
db: null,
scanArchives: true,
active: true
}
});
async function scanFile(filePath) {
try {
const {isInfected, virusName} = await ClamScan.isInfected(filePath);
return {
clean: !isInfected,
reason: isInfected ? `Infected with ${virusName}` : null
};
} catch (error) {
return { clean: false, reason: 'Scan failed' };
}
}
// Example usage
app.post('/upload', upload.single('file'), async (req, res) => {
const file = req.file;
// Scan file for viruses
const scanResult = await scanFile(file.path);
if (!scanResult.clean) {
await fs.promises.unlink(file.path);
return res.status(400).json({
error: 'File rejected',
reason: scanResult.reason
});
}
// Continue with secure file processing...
});
Antivirus Scanning Best Practices
-
Choose appropriate scanning solutions:
- Consider performance impact
- Update virus definitions regularly
- Monitor scanning errors
-
Handle infected files:
- Remove immediately
- Log incidents
- Notify administrators
-
Implement scanning strategies:
- Scan before processing
- Consider async scanning
- Monitor resource usage
Conclusion
Implementing secure file upload fundamentals is crucial for protecting your application and users. By properly implementing file size limitations, MIME type validation, extension validation, and antivirus scanning, you create a robust first line of defense against file upload vulnerabilities.
Remember to:
- Always validate files server-side
- Use multiple validation methods
- Handle errors gracefully
- Keep security measures updated
- Monitor and log file upload activities