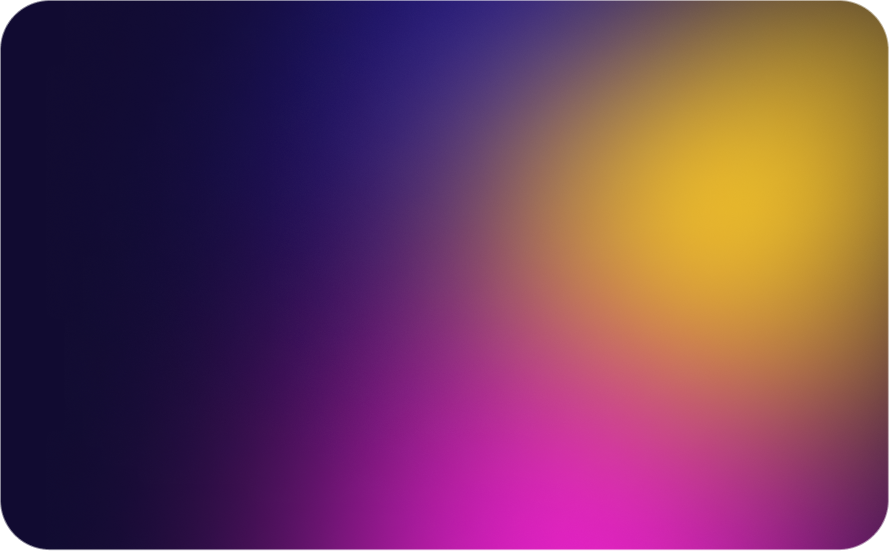
Getting Started with Vibe Coding: A Beginner's Guide to Full-Stack App Builders
Introduction
The software development landscape is undergoing a revolutionary transformation. For decades, building applications required extensive programming knowledge, years of practice, and mastery of complex languages and frameworks. Today, a new paradigm called “vibe coding” is democratizing software development, making it accessible to virtually anyone with an idea—regardless of their technical background.
Vibe coding represents a fundamental shift in how we approach building software. Instead of writing code line by line, you describe what you want in plain English, and AI tools generate the code for you. This approach is particularly powerful when combined with full-stack app builders that handle everything from user interfaces to databases and deployment.
In this comprehensive guide, we’ll explore the world of vibe coding through the lens of five leading full-stack app builders: Lovable.dev, Bolt.new/Bolt.diy, Tempo Labs, Base44, and Replit. Whether you’re a non-technical founder looking to build your first MVP, a product manager wanting to prototype ideas without engineering resources, or a curious developer exploring new ways to accelerate your workflow, this guide will help you understand and leverage these powerful tools.
We’ll walk through the fundamentals of vibe coding, compare the strengths and weaknesses of each platform, and provide practical examples to help you get started. By the end of this article, you’ll have a clear understanding of how to transform your ideas into functional applications using natural language prompts and AI-powered tools.
What is Vibe Coding?
Vibe coding, a term coined by Andrej Karpathy (former Director of AI at Tesla and a prominent AI researcher), refers to a development approach where users describe what they want in natural language and AI generates the code. In a viral tweet, Karpathy described it as coding where you “fully give in to the vibes, embrace exponentials, and forget that the code even exists.”
This approach has gained significant popularity with the advancement of large language models (LLMs) like GPT-4, Claude, and others that power today’s AI coding assistants. Unlike traditional coding, where developers must understand programming languages, data structures, algorithms, and frameworks, vibe coding allows you to focus on what you want to build rather than how to build it.
The key characteristics of vibe coding include:
- Natural language prompts: You describe features and functionality in plain English rather than programming languages.
- AI code generation: The AI interprets your prompts and generates the necessary code.
- Iterative refinement: You provide feedback and additional prompts to refine the generated code.
- Minimal technical knowledge required: While some understanding of software concepts helps, you don’t need to know how to code.
- Focus on outcomes: The emphasis is on what you want to achieve rather than implementation details.
Vibe coding is particularly powerful when combined with full-stack app builders that provide integrated environments for developing, testing, and deploying applications. These platforms handle the complexities of frontend interfaces, backend logic, database management, authentication, and deployment—allowing you to focus on your application’s unique features and value proposition.
Overview of Full-Stack App Builders
Let’s explore the five leading full-stack app builders that excel at vibe coding:
Lovable.dev
Lovable.dev is a user-friendly AI-powered full-stack app builder designed to make web application development accessible to everyone. It provides a visual interface where you can select parts of your application and request specific changes using natural language.
Key features:
- Visual editing interface with AI-powered code generation
- Integrated with Supabase for authentication and database operations
- GitHub repository integration for code management
- Real-time collaboration capabilities
- Deployment to production with a single click
Best for: Non-technical founders, product managers, and developers looking for rapid prototyping and development.
Bolt.new / Bolt.diy
Created by Stackblitz, Bolt.new enables building full-stack applications using AI visually. It stands out with its ability to import designs directly from Figma and convert them into functional code.
Key features:
- Figma design import and conversion to code
- Web containers that run Node.js in the browser
- Supabase integration for authentication and database functionality
- Visual editing with AI assistance
- One-click deployment
Best for: Designers and product teams who want to turn designs into functional applications quickly.
Tempo Labs
Tempo Labs provides controls for both low-code and intermediate programmers, with a focus on comprehensive application development.
Key features:
- Generates Product Requirements Documents (PRD) and user flow diagrams
- Supports payment integration with Stripe and Polar
- Enables authentication and database with Supabase or Convex
- AI-assisted development with natural language prompts
- Comprehensive documentation generation
Best for: Product managers and technical founders who need detailed documentation alongside development.
Base44
Base44 offers a more bare-bones starter template geared towards advanced developers who want AI assistance while maintaining control over their codebase.
Key features:
- Streamlined interface for rapid application development
- More control over code structure and architecture
- AI assistance for specific coding tasks
- Integration with popular frameworks and libraries
- Flexible deployment options
Best for: Developers who want AI assistance but prefer more control over their code.
Replit
Replit provides a complete development environment in the browser with AI assistance for building applications using natural language prompts.
Key features:
- Complete IDE in the browser
- AI agent that helps build apps using natural language
- Direct deployment to production servers
- Collaborative coding environment
- Support for multiple programming languages and frameworks
Best for: Developers and teams who want a complete development environment with AI assistance.
Getting Started with Vibe Coding
Now that we understand what vibe coding is and the platforms available, let’s walk through the process of getting started with each platform.
Step 1: Define Your Project
Before diving into any platform, clearly define what you want to build. Consider:
- Purpose: What problem does your application solve?
- Core features: What are the essential functions your app needs?
- User flow: How will users interact with your application?
- Data requirements: What information will your app store and process?
Having a clear vision will make your prompts more effective and help the AI generate more relevant code.
Step 2: Choose the Right Platform
Based on your project requirements and personal preferences, select the platform that best suits your needs:
- Lovable.dev if you want a visual interface with strong Supabase integration
- Bolt.new if you’re starting with Figma designs
- Tempo Labs if you need comprehensive documentation and product management features
- Base44 if you want more control over your code
- Replit if you prefer a complete development environment
Step 3: Set Up Your Environment
Let’s walk through the setup process for each platform:
Lovable.dev Setup
- Visit Lovable.dev and sign up for an account
- Create a new project by clicking “New Project”
- Choose a template or start from scratch
- Connect to Supabase for authentication and database (optional)
- Start building with natural language prompts
// Example of a Lovable.dev prompt and generated code
// Prompt: "Create a user authentication system with email and password"
// Generated React component for sign-up form
import { useState } from 'react';
import { supabase } from '../lib/supabaseClient';
export default function SignUp() {
const [email, setEmail] = useState('');
const [password, setPassword] = useState('');
const [loading, setLoading] = useState(false);
const [error, setError] = useState(null);
async function handleSignUp(e) {
e.preventDefault();
setLoading(true);
setError(null);
try {
const { error } = await supabase.auth.signUp({
email,
password,
});
if (error) throw error;
alert('Check your email for the confirmation link!');
} catch (error) {
setError(error.message);
} finally {
setLoading(false);
}
}
return (
<div className="auth-container">
<h2>Sign Up</h2>
{error && <div className="error">{error}</div>}
<form onSubmit={handleSignUp}>
<div className="form-group">
<label htmlFor="email">Email</label>
<input
id="email"
type="email"
value={email}
onChange={(e) => setEmail(e.target.value)}
required
/>
</div>
<div className="form-group">
<label htmlFor="password">Password</label>
<input
id="password"
type="password"
value={password}
onChange={(e) => setPassword(e.target.value)}
required
/>
</div>
<button type="submit" disabled={loading}>
{loading ? 'Loading...' : 'Sign Up'}
</button>
</form>
</div>
);
}
Bolt.new Setup
- Visit Bolt.new and sign up for an account
- Create a new project
- Import a Figma design (optional) or start from scratch
- Connect to Supabase for backend functionality
- Start building with AI assistance
// Example of a Bolt.new prompt and generated code
// Prompt: "Create a product listing page with filtering options"
// Generated TypeScript code for product listing
import { useState, useEffect } from 'react';
import { supabase } from '../lib/supabaseClient';
interface Product {
id: number;
name: string;
price: number;
category: string;
heroImage: "/images/blog/getting-started-with-vibe-coding-a-beginners-guide-to-full-stack-app-builders.jpg"
description: string;
}
export default function ProductListing() {
const [products, setProducts] = useState<Product[]>([]);
const [filteredProducts, setFilteredProducts] = useState<Product[]>([]);
const [categories, setCategories] = useState<string[]>([]);
const [selectedCategory, setSelectedCategory] = useState<string>('all');
const [loading, setLoading] = useState<boolean>(true);
const [error, setError] = useState<string | null>(null);
useEffect(() => {
async function fetchProducts() {
try {
const { data, error } = await supabase
.from('products')
.select('*');
if (error) throw error;
setProducts(data || []);
setFilteredProducts(data || []);
// Extract unique categories
const uniqueCategories = [...new Set(data?.map(p => p.category))];
setCategories(uniqueCategories);
} catch (error) {
setError('Error fetching products');
console.error(error);
} finally {
setLoading(false);
}
}
fetchProducts();
}, []);
useEffect(() => {
if (selectedCategory === 'all') {
setFilteredProducts(products);
} else {
setFilteredProducts(
products.filter(p => p.category === selectedCategory)
);
}
}, [selectedCategory, products]);
return (
<div className="products-container">
<h1>Our Products</h1>
<div className="filter-container">
<label htmlFor="category-filter">Filter by category:</label>
<select
id="category-filter"
value={selectedCategory}
onChange={(e) => setSelectedCategory(e.target.value)}
>
<option value="all">All Categories</option>
{categories.map(category => (
<option key={category} value={category}>
{category}
</option>
))}
</select>
</div>
{loading ? (
<p>Loading products...</p>
) : error ? (
<p className="error">{error}</p>
) : (
<div className="product-grid">
{filteredProducts.map(product => (
<div key={product.id} className="product-card">
<img src={product.image_url} alt={product.name} />
<h3>{product.name}</h3>
<p className="price">${product.price.toFixed(2)}</p>
<p className="category">{product.category}</p>
<p className="description">{product.description}</p>
<button>Add to Cart</button>
</div>
))}
</div>
)}
</div>
);
}
Tempo Labs Setup
- Visit Tempo Labs and create an account
- Start a new project
- Define your project requirements using natural language
- Generate a PRD and user flow diagrams
- Begin building with AI assistance
// Example of a Tempo Labs prompt and generated code
// Prompt: "Create a payment processing system with Stripe"
// Generated JavaScript code for Stripe integration
import { useState } from 'react';
import { loadStripe } from '@stripe/stripe-js';
import {
CardElement,
Elements,
useStripe,
useElements,
} from '@stripe/react-stripe-js';
// Load Stripe
const stripePromise = loadStripe(process.env.NEXT_PUBLIC_STRIPE_PUBLISHABLE_KEY);
function CheckoutForm({ amount, onSuccess }) {
const [loading, setLoading] = useState(false);
const [error, setError] = useState(null);
const stripe = useStripe();
const elements = useElements();
const handleSubmit = async (event) => {
event.preventDefault();
if (!stripe || !elements) {
// Stripe.js has not loaded yet
return;
}
setLoading(true);
setError(null);
try {
// Create payment intent on the server
const response = await fetch('/api/create-payment-intent', {
method: 'POST',
headers: {
'Content-Type': 'application/json',
},
body: JSON.stringify({ amount }),
});
if (!response.ok) {
throw new Error('Network response was not ok');
}
const data = await response.json();
// Confirm the payment
const result = await stripe.confirmCardPayment(data.clientSecret, {
payment_method: {
card: elements.getElement(CardElement),
billing_details: {
name: 'Customer Name',
},
},
});
if (result.error) {
throw result.error;
}
if (result.paymentIntent.status === 'succeeded') {
onSuccess(result.paymentIntent);
}
} catch (error) {
setError(error.message);
} finally {
setLoading(false);
}
};
return (
<form onSubmit={handleSubmit} className="payment-form">
<div className="form-row">
<label htmlFor="card-element">Credit or debit card</label>
<CardElement id="card-element" />
</div>
{error && <div className="error">{error}</div>}
<button type="submit" disabled={!stripe || loading}>
{loading ? 'Processing...' : `Pay $${(amount / 100).toFixed(2)}`}
</button>
</form>
);
}
export default function PaymentPage() {
const [paymentCompleted, setPaymentCompleted] = useState(false);
const [paymentIntent, setPaymentIntent] = useState(null);
const handlePaymentSuccess = (intent) => {
setPaymentIntent(intent);
setPaymentCompleted(true);
};
return (
<div className="payment-container">
<h1>Complete Your Purchase</h1>
{paymentCompleted ? (
<div className="success-message">
<h2>Payment Successful!</h2>
<p>Thank you for your purchase.</p>
<p>Transaction ID: {paymentIntent.id}</p>
</div>
) : (
<Elements stripe={stripePromise}>
<CheckoutForm
amount={2500} // $25.00
onSuccess={handlePaymentSuccess}
/>
</Elements>
)}
</div>
);
}
Base44 Setup
- Visit Base44 and sign up
- Create a new project
- Select your preferred tech stack
- Start building with AI assistance
// Example of a Base44 prompt and generated code
// Prompt: "Create a real-time chat application"
// Generated JavaScript code for a real-time chat app
import { useState, useEffect, useRef } from 'react';
import { supabase } from '../lib/supabaseClient';
export default function ChatRoom({ roomId, userId, username }) {
const [messages, setMessages] = useState([]);
const [newMessage, setNewMessage] = useState('');
const [loading, setLoading] = useState(true);
const [error, setError] = useState(null);
const messagesEndRef = useRef(null);
// Scroll to bottom of messages
const scrollToBottom = () => {
messagesEndRef.current?.scrollIntoView({ behavior: 'smooth' });
};
// Fetch initial messages
useEffect(() => {
async function fetchMessages() {
try {
const { data, error } = await supabase
.from('messages')
.select('*')
.eq('room_id', roomId)
.order('created_at', { ascending: true });
if (error) throw error;
setMessages(data || []);
} catch (error) {
setError('Error fetching messages');
console.error(error);
} finally {
setLoading(false);
}
}
fetchMessages();
// Subscribe to new messages
const subscription = supabase
.channel(`room:${roomId}`)
.on('postgres_changes', {
event: 'INSERT',
schema: 'public',
table: 'messages',
filter: `room_id=eq.${roomId}`
}, (payload) => {
setMessages(previous => [...previous, payload.new]);
})
.subscribe();
return () => {
supabase.removeChannel(subscription);
};
}, [roomId]);
// Scroll to bottom when messages change
useEffect(() => {
scrollToBottom();
}, [messages]);
// Send a new message
async function handleSendMessage(e) {
e.preventDefault();
if (!newMessage.trim()) return;
try {
const { error } = await supabase
.from('messages')
.insert([
{
room_id: roomId,
user_id: userId,
username: username,
content: newMessage,
}
]);
if (error) throw error;
setNewMessage('');
} catch (error) {
console.error('Error sending message:', error);
}
}
return (
<div className="chat-container">
<div className="messages-container">
{loading ? (
<p>Loading messages...</p>
) : error ? (
<p className="error">{error}</p>
) : messages.length === 0 ? (
<p>No messages yet. Start the conversation!</p>
) : (
messages.map(message => (
<div
key={message.id}
className={`message ${message.user_id === userId ? 'own-message' : ''}`}
>
<div className="message-header">
<span className="username">{message.username}</span>
<span className="timestamp">
{new Date(message.created_at).toLocaleTimeString()}
</span>
</div>
<div className="message-content">{message.content}</div>
</div>
))
)}
<div ref={messagesEndRef} />
</div>
<form onSubmit={handleSendMessage} className="message-form">
<input
type="text"
value={newMessage}
onChange={(e) => setNewMessage(e.target.value)}
placeholder="Type a message..."
required
/>
<button type="submit">Send</button>
</form>
</div>
);
}
Replit Setup
- Visit Replit and create an account
- Create a new repl
- Choose your preferred language or framework
- Use the AI assistant to help build your application
# Example of a Replit prompt and generated code
# Prompt: "Create a Flask API for a todo list application"
# Generated Python code for a Flask API
from flask import Flask, request, jsonify
from flask_cors import CORS
import sqlite3
import uuid
from datetime import datetime
app = Flask(__name__)
CORS(app) # Enable CORS for all routes
# Initialize database
def init_db():
conn = sqlite3.connect('todos.db')
c = conn.cursor()
c.execute('''
CREATE TABLE IF NOT EXISTS todos (
id TEXT PRIMARY KEY,
title TEXT NOT NULL,
description TEXT,
completed BOOLEAN NOT NULL DEFAULT 0,
created_at TIMESTAMP NOT NULL,
updated_at TIMESTAMP NOT NULL
)
''')
conn.commit()
conn.close()
init_db()
# Helper function to convert row to dictionary
def row_to_dict(row):
return {
'id': row[0],
'title': row[1],
'description': row[2],
'completed': bool(row[3]),
'created_at': row[4],
'updated_at': row[5]
}
# Get all todos
@app.route('/api/todos', methods=['GET'])
def get_todos():
conn = sqlite3.connect('todos.db')
c = conn.cursor()
c.execute('SELECT * FROM todos ORDER BY created_at DESC')
todos = [row_to_dict(row) for row in c.fetchall()]
conn.close()
return jsonify(todos)
# Get a specific todo
@app.route('/api/todos/<todo_id>', methods=['GET'])
def get_todo(todo_id):
conn = sqlite3.connect('todos.db')
c = conn.cursor()
c.execute('SELECT * FROM todos WHERE id = ?', (todo_id,))
todo = c.fetchone()
conn.close()
if todo is None:
return jsonify({'error': 'Todo not found'}), 404
return jsonify(row_to_dict(todo))
# Create a new todo
@app.route('/api/todos', methods=['POST'])
def create_todo():
data = request.get_json()
if not data or 'title' not in data:
return jsonify({'error': 'Title is required'}), 400
todo_id = str(uuid.uuid4())
now = datetime.utcnow().isoformat()
conn = sqlite3.connect('todos.db')
c = conn.cursor()
c.execute(
'INSERT INTO todos (id, title, description, completed, created_at, updated_at) VALUES (?, ?, ?, ?, ?, ?)',
(todo_id, data['title'], data.get('description', ''), data.get('completed', False), now, now)
)
conn.commit()
conn.close()
return jsonify({
'id': todo_id,
'title': data['title'],
'description': data.get('description', ''),
'completed': data.get('completed', False),
'created_at': now,
'updated_at': now
}), 201
# Update a todo
@app.route('/api/todos/<todo_id>', methods=['PUT'])
def update_todo(todo_id):
data = request.get_json()
if not data:
return jsonify({'error': 'No data provided'}), 400
conn = sqlite3.connect('todos.db')
c = conn.cursor()
# Check if todo exists
c.execute('SELECT * FROM todos WHERE id = ?', (todo_id,))
todo = c.fetchone()
if todo is None:
conn.close()
return jsonify({'error': 'Todo not found'}), 404
# Update fields
updates = []
values = []
if 'title' in data:
updates.append('title = ?')
values.append(data['title'])
if 'description' in data:
updates.append('description = ?')
values.append(data['description'])
if 'completed' in data:
updates.append('completed = ?')
values.append(1 if data['completed'] else 0)
updates.append('updated_at = ?')
now = datetime.utcnow().isoformat()
values.append(now)
# Add todo_id to values
values.append(todo_id)
# Execute update
c.execute(
f'UPDATE todos SET {", ".join(updates)} WHERE id = ?',
tuple(values)
)
conn.commit()
# Get updated todo
c.execute('SELECT * FROM todos WHERE id = ?', (todo_id,))
updated_todo = c.fetchone()
conn.close()
return jsonify(row_to_dict(updated_todo))
# Delete a todo
@app.route('/api/todos/<todo_id>', methods=['DELETE'])
def delete_todo(todo_id):
conn = sqlite3.connect('todos.db')
c = conn.cursor()
# Check if todo exists
c.execute('SELECT * FROM todos WHERE id = ?', (todo_id,))
todo = c.fetchone()
if todo is None:
conn.close()
return jsonify({'error': 'Todo not found'}), 404
# Delete todo
c.execute('DELETE FROM todos WHERE id = ?', (todo_id,))
conn.commit()
conn.close()
return jsonify({'message': 'Todo deleted successfully'})
if __name__ == '__main__':
app.run(host='0.0.0.0', port=8080, debug=True)
Step 4: Create Your First Application
Now that you’ve set up your environment, let’s create a simple application using vibe coding. We’ll build a basic task management application with each platform.
Effective Prompt Engineering
The key to successful vibe coding is effective prompt engineering. Here are some tips:
- Be specific: Clearly describe what you want, including functionality, design, and behavior.
- Provide context: Explain the purpose of the feature and how it fits into the larger application.
- Use examples: Reference existing applications or features to clarify your requirements.
- Iterate: Start with a basic prompt and refine it based on the generated code.
- Break it down: For complex features, break them into smaller components and prompt for each separately.
Here’s an example of an effective prompt:
Create a task management application with the following features:
1. User authentication (sign up, login, logout)
2. Ability to create, edit, and delete tasks
3. Task categorization with labels
4. Due date assignment and reminder functionality
5. Task filtering and sorting options
6. Responsive design that works on mobile and desktop
The application should use a modern, clean design with a blue and white color scheme.
This prompt is specific, provides context, and breaks down the requirements into clear components.
Comparison of User Experiences
Each platform offers a unique user experience. Let’s compare them:
Platform | User Interface | Learning Curve | Customization | Deployment |
---|---|---|---|---|
Lovable.dev | Visual editor with AI chat | Low | Medium | One-click |
Bolt.new | Visual editor with Figma import | Low-Medium | High | One-click |
Tempo Labs | PRD-focused with AI assistance | Medium | Medium-High | Guided |
Base44 | Code-centric with AI assistance | Medium-High | Very High | Manual |
Replit | Complete IDE with AI chat | Medium | Very High | One-click |
Lovable.dev Experience
Lovable.dev offers a visual interface where you can see your application as you build it. The AI assistant is integrated directly into the editor, allowing you to select elements and request changes using natural language.
The workflow typically involves:
- Creating a new project
- Using prompts to generate initial components
- Selecting elements and requesting specific changes
- Testing the application in real-time
- Deploying with a single click
Bolt.new Experience
Bolt.new excels at turning designs into functional applications. If you start with a Figma design, Bolt can import it and generate the corresponding code. The AI assistant helps you add functionality to your design.
The workflow typically involves:
- Importing a Figma design or starting from scratch
- Using prompts to generate functionality
- Testing in the integrated preview
- Refining with additional prompts
- Deploying to production
Tempo Labs Experience
Tempo Labs takes a more structured approach, focusing on product requirements and documentation alongside development. It’s ideal for teams that need comprehensive documentation.
The workflow typically involves:
- Defining product requirements using natural language
- Generating a PRD and user flow diagrams
- Building the application with AI assistance
- Testing and refining
- Deploying with guided steps
Base44 Experience
Base44 provides more control over your code while still offering AI assistance. It’s geared towards developers who want to leverage AI but maintain control over their codebase.
The workflow typically involves:
- Setting up a project with your preferred tech stack
- Using prompts to generate specific components or features
- Manually editing and refining the code
- Testing in the integrated environment
- Deploying using standard methods
Replit Experience
Replit offers a complete development environment with AI assistance. It supports multiple programming languages and frameworks, making it versatile for various projects.
The workflow typically involves:
- Creating a new repl with your preferred language/framework
- Using the AI assistant to generate code
- Editing and refining in the integrated IDE
- Testing in the integrated preview
- Deploying directly from Replit
Best Practices for Effective Prompt Engineering
The quality of your prompts significantly impacts the code generated by AI. Here are some best practices for effective prompt engineering:
1. Be Specific and Detailed
Provide as much detail as possible about what you want to build. Include information about:
- Functionality
- Design preferences
- User interactions
- Data requirements
- Edge cases
Example of a vague prompt:
Create a contact form.
Example of a specific prompt:
Create a contact form with fields for name, email, phone number, and message. The form should validate that the email is in the correct format and that all fields are filled out before submission. On submission, show a success message and clear the form. The form should have a clean design with rounded corners and a submit button that matches our brand color (#3498db).
2. Use a Structured Format
Organize your prompts in a structured format to make them easier for the AI to understand:
Feature: [Brief description]
Requirements:
1. [Requirement 1]
2. [Requirement 2]
3. [Requirement 3]
Design preferences:
- [Preference 1]
- [Preference 2]
Technical constraints:
- [Constraint 1]
- [Constraint 2]
3. Provide Context
Explain how the feature fits into the larger application and why it’s important:
I'm building a task management application for small teams. I need a dashboard that shows task statistics (total tasks, completed tasks, overdue tasks) at the top and a list of upcoming deadlines below. This will help team members quickly understand their workload and priorities.
4. Reference Existing Examples
Point to existing applications or features that are similar to what you want:
Create a calendar view similar to Google Calendar, where users can see their tasks organized by day, week, or month. Users should be able to drag and drop tasks to reschedule them.
5. Iterate and Refine
Start with a basic prompt and then refine it based on the generated code:
Initial prompt:
Create a user profile page.
Refined prompt after seeing the initial code:
The profile page looks good, but I'd like to make these changes:
1. Add an "Edit Profile" button that opens a modal with form fields
2. Include a section for user statistics (tasks completed, on-time percentage)
3. Add the ability to upload a profile picture
4. Show the user's recent activity in a timeline format
Security Considerations
While vibe coding makes application development more accessible, it’s important to consider security implications:
-
Review generated code: AI may generate code with security vulnerabilities. Always review the code, especially authentication, data handling, and API endpoints.
-
Avoid hardcoded credentials: Ensure that sensitive information like API keys and database credentials are stored securely, not hardcoded in the application.
-
Implement proper authentication: Verify that authentication mechanisms follow best practices, including secure password storage and proper session management.
-
Validate user inputs: Check that all user inputs are properly validated and sanitized to prevent injection attacks.
-
Set up proper access controls: Ensure that authorization checks are in place to restrict access to sensitive functionality and data.
Each platform has different security considerations:
- Lovable.dev and Bolt.new: Review Supabase security settings and ensure proper row-level security policies.
- Tempo Labs: Verify that payment integrations follow security best practices.
- Base44: Manually review generated code for security vulnerabilities.
- Replit: Be cautious with environment variables and secrets management.
Conclusion
Vibe coding with full-stack app builders represents a paradigm shift in software development, making it accessible to a broader audience while accelerating development for experienced programmers. By leveraging AI to generate code from natural language prompts, these platforms enable you to focus on what you want to build rather than how to build it.
In this guide, we’ve explored five leading platforms—Lovable.dev, Bolt.new/Bolt.diy, Tempo Labs, Base44, and Replit—each with its unique strengths and approaches. We’ve walked through the process of getting started with each platform, compared their user experiences, and provided best practices for effective prompt engineering.
As you begin your vibe coding journey, remember that the quality of your prompts significantly impacts the quality of the generated code. Be specific, provide context, and iterate based on the results. Also, don’t forget to consider security implications and review the generated code for potential vulnerabilities.
Whether you’re a non-technical founder building your first MVP, a product manager creating prototypes, or a developer looking to accelerate your workflow, vibe coding with full-stack app builders offers a powerful approach to turning your ideas into reality.
Additional Resources
- Lovable.dev Documentation
- Bolt.new Tutorials
- Tempo Labs Guide
- Base44 Documentation
- Replit Documentation
- Prompt Engineering Guide
- OWASP Top 10 Web Application Security Risks
References
- Karpathy, A. (2023). “Vibe Coding: A New Paradigm.” Twitter. Retrieved from https://twitter.com/karpathy/status/1677734762700259328
- Smith, J. (2025). “The Rise of AI-Assisted Development.” TechCrunch. Retrieved from https://techcrunch.com/2025/01/15/the-rise-of-ai-assisted-development/
- Johnson, L. (2024). “Comparing Full-Stack App Builders.” Dev.to. Retrieved from https://dev.to/ljohnson/comparing-full-stack-app-builders-4n2m
- Brown, R. (2025). “Security Considerations for AI-Generated Code.” OWASP Blog. Retrieved from https://owasp.org/blog/2025/02/security-considerations-for-ai-generated-code
- Davis, M. (2025). “Prompt Engineering Best Practices.” AI Engineering Journal. Retrieved from https://aiengineering.com/prompt-engineering-best-practices