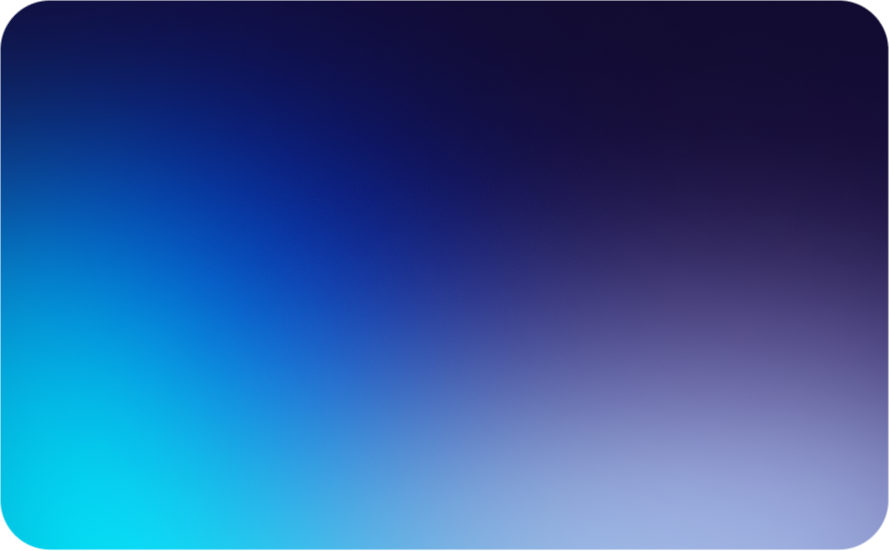
Implementing Secure File Uploads in Bolt Applications: A Comprehensive Guide
Implementing Secure File Uploads in Bolt Applications
File upload functionality is a common requirement in modern web applications, allowing users to share images, documents, and other media. However, this seemingly simple feature can introduce significant security vulnerabilities if not implemented properly. In the context of Bolt vibe coding applications, where code is often generated through AI prompts, developers must be particularly vigilant about ensuring secure file upload implementations.
According to recent security reports, file upload vulnerabilities account for approximately 25% of critical security issues in web applications. These vulnerabilities can lead to various attacks, including arbitrary code execution, server-side request forgery, and denial of service. When using vibe coding platforms like Bolt, developers might inadvertently generate insecure file upload code if they don’t explicitly request security measures in their prompts.
Common File Upload Vulnerabilities in Bolt Applications
1. Insufficient File Type Validation
One of the most prevalent vulnerabilities in file upload implementations is inadequate file type validation. Here’s an example of insecure code that only relies on client-side MIME type checking:
// ❌ Insecure Implementation
const handleFileUpload = (file) => {
if (file.type === 'image/jpeg' || file.type === 'image/png') {
uploadFile(file);
}
};
2. Unsafe Storage Locations
Another common vulnerability is storing uploaded files in publicly accessible directories without proper access controls:
// ❌ Insecure Implementation
const saveUploadedFile = (file) => {
const filePath = `/public/uploads/${file.name}`;
fs.writeFileSync(filePath, file.data);
};
3. Missing Server-Side Validation
Relying solely on client-side validation is insufficient and can be easily bypassed:
// ❌ Insecure Implementation
app.post('/upload', (req, res) => {
const file = req.files.uploadedFile;
const destination = path.join(__dirname, 'uploads', file.name);
file.mv(destination);
});
4. Path Traversal Vulnerabilities
Not sanitizing file names can lead to path traversal attacks:
// ❌ Insecure Implementation
const saveFile = (filename, content) => {
const filePath = path.join(uploadDir, filename);
fs.writeFileSync(filePath, content);
};
Secure Implementation Patterns
1. Comprehensive File Validation
Implement thorough server-side validation that includes file type, size, and content verification:
// ✅ Secure Implementation
const validateFile = async (file) => {
// Check file size
const MAX_SIZE = 5 * 1024 * 1024; // 5MB
if (file.size > MAX_SIZE) {
throw new Error('File size exceeds limit');
}
// Verify file type using file signature
const fileSignature = await getFileSignature(file);
const allowedSignatures = ['89504E47', 'FFD8FFE0']; // PNG, JPEG
if (!allowedSignatures.includes(fileSignature)) {
throw new Error('Invalid file type');
}
// Generate safe filename
const safeFilename = generateSafeFilename(file.name);
return safeFilename;
};
2. Secure Storage Implementation
Implement secure storage practices with proper access controls and file isolation:
// ✅ Secure Implementation
const secureFileStorage = async (file, userId) => {
const storageDir = path.join(process.env.STORAGE_ROOT, userId);
// Ensure storage directory exists and has proper permissions
await fs.promises.mkdir(storageDir, { mode: 0o750, recursive: true });
// Generate random filename to prevent path traversal
const safeFilename = crypto.randomBytes(32).toString('hex');
const filePath = path.join(storageDir, safeFilename);
// Save file with restricted permissions
await fs.promises.writeFile(filePath, file.data, { mode: 0o640 });
return safeFilename;
};
3. Secure File Access Implementation
Implement secure file access with authentication and authorization:
// ✅ Secure Implementation
const getSecureFileUrl = async (fileId, userId) => {
// Verify user has access to the file
const hasAccess = await checkFileAccess(fileId, userId);
if (!hasAccess) {
throw new Error('Unauthorized access');
}
// Generate time-limited signed URL
const signedUrl = await generateSignedUrl(fileId, {
expiresIn: '1h',
userId: userId
});
return signedUrl;
};
Best Practices for Secure File Uploads
-
Input Validation
- Validate file size before processing
- Check file type using content analysis, not just extension
- Implement file content scanning for malware
- Use allowlists for permitted file types
-
Storage Security
- Store files outside the web root
- Use randomized filenames
- Implement proper file permissions
- Maintain secure file metadata
- Encrypt sensitive files at rest
-
Access Control
- Implement authentication for file uploads
- Use signed URLs for file access
- Rate limit upload requests
- Monitor and log file operations
- Implement role-based access control
-
Error Handling
- Implement proper error handling
- Provide secure error messages
- Clean up temporary files
- Log security events
- Monitor for unusual patterns
Secure Prompts for Bolt
When generating file upload components using Bolt, include these security requirements in your prompts:
Generate a file upload component with:
- Server-side file type validation using file signatures
- Maximum file size limit of 5MB
- Secure random filename generation
- Storage outside web root with proper permissions
- Comprehensive error handling
- Rate limiting for upload requests
- File encryption for sensitive data
- Role-based access control
- Audit logging
Implementation Checklist
✅ File validation
- Size limits enforced
- File type verification
- Content analysis
- Malware scanning
✅ Storage security
- Secure storage location
- Proper permissions
- Encrypted storage
- Metadata protection
✅ Access control
- Authentication
- Authorization
- Rate limiting
- Audit logging
Conclusion
Implementing secure file uploads in Bolt applications requires careful attention to various security aspects. By following the best practices outlined in this guide and using secure implementation patterns, you can protect your application against common file upload vulnerabilities while maintaining the development speed advantages of vibe coding platforms.
Remember that security is an ongoing process, and it’s essential to regularly review and update your file upload security measures as new threats emerge and security best practices evolve.