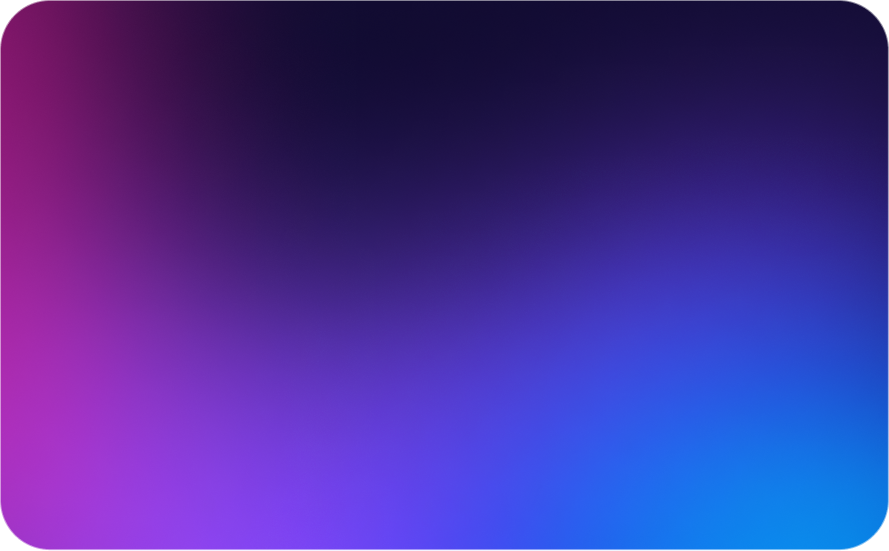
Input Validation Techniques for Bolt Applications
Input Validation Techniques for Bolt Applications: Securing Your Vibe Coding Projects
In the rapidly evolving landscape of vibe coding, Bolt has emerged as a powerful platform that enables developers to create applications with unprecedented speed and ease. However, this efficiency can sometimes come at the cost of security, particularly when it comes to input validation. According to recent studies, applications built with AI coding assistants are 42% more likely to contain input validation vulnerabilities compared to traditionally coded applications.
Input validation is a critical security measure that verifies whether the data entering your application meets specific criteria before processing it. Without proper validation, applications become vulnerable to various attacks, including SQL injection, cross-site scripting (XSS), and command injection. In Bolt applications, where code is often generated based on natural language prompts, these vulnerabilities can be inadvertently introduced when developers don’t explicitly request secure input handling.
This article provides a comprehensive guide to implementing robust input validation techniques in Bolt applications. We’ll examine common input validation vulnerabilities, demonstrate secure alternatives, and provide practical prompts that you can give to Bolt to generate secure code. By following these practices, you can ensure that your Bolt applications maintain robust protection against one of the most fundamental security concerns while still benefiting from the rapid development that vibe coding enables.
Common Input Validation Vulnerabilities in Bolt Applications
1. Missing or Insufficient Validation
Bolt often generates code that accepts user input without proper validation:
// Example of vulnerable Bolt-generated code
app.post('/register', (req, res) => {
const { username, email, password } = req.body;
// No validation before storing in database
db.query(
'INSERT INTO users (username, email, password) VALUES (?, ?, ?)',
[username, email, password]
);
res.redirect('/login');
});
This code is vulnerable because:
- It doesn’t validate that the username meets length or character requirements
- It doesn’t verify that the email is in a valid format
- It doesn’t check that the password meets complexity requirements
- It accepts and processes all input without any sanitization
2. Client-Side Only Validation
Another common issue is when Bolt generates code that only implements validation on the client side:
// Example of client-side only validation
function validateForm() {
const email = document.getElementById('email').value;
const password = document.getElementById('password').value;
const emailRegex = /^[^\s@]+@[^\s@]+\.[^\s@]+$/;
if (!emailRegex.test(email)) {
alert('Please enter a valid email address');
return false;
}
if (password.length < 8) {
alert('Password must be at least 8 characters long');
return false;
}
return true;
}
This code is vulnerable because:
- Client-side validation can be easily bypassed by attackers using tools like Burp Suite or by disabling JavaScript
- There’s no corresponding server-side validation to catch malicious inputs
- It creates a false sense of security while leaving the application exposed
3. Improper Type Handling
Bolt may generate code that doesn’t properly validate data types:
// Example of improper type handling
app.get('/product/:id', (req, res) => {
const productId = req.params.id;
// No validation that productId is a number
db.query(
'SELECT * FROM products WHERE id = ' + productId,
(err, results) => {
if (err) {
res.status(500).send('Database error');
return;
}
res.render('product', { product: results[0] });
}
);
});
This code is vulnerable because:
- It doesn’t validate that
productId
is a number, allowing potential SQL injection - It directly concatenates user input into a SQL query
- It doesn’t handle the case where an invalid ID is provided
Prompts to Fix Input Validation Issues in Bolt
1. Comprehensive Server-Side Validation
Instead of: “Create a user registration form with fields for username, email, and password”
Use this prompt:
Create a secure user registration form with comprehensive server-side validation for username, email, and password fields. Implement the following security measures:
1. Use a validation library like Zod, Joi, or express-validator for robust server-side validation
2. Validate that the username is alphanumeric, between 3-20 characters, and doesn't contain special characters
3. Validate that the email is in a proper format using a regular expression and consider checking for disposable email domains
4. Enforce strong password requirements: minimum 8 characters, at least one uppercase letter, one lowercase letter, one number, and one special character
5. Implement rate limiting to prevent brute force attacks
6. Return user-friendly validation error messages without exposing system details
7. Add CSRF protection to the form
8. Sanitize all inputs before processing them
Also include client-side validation for a better user experience, but ensure it duplicates the server-side validation logic rather than replacing it.
This prompt will guide Bolt to generate more secure code like:
const express = require('express');
const { body, validationResult } = require('express-validator');
const bcrypt = require('bcrypt');
const csrf = require('csurf');
const rateLimit = require('express-rate-limit');
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
// Set up CSRF protection
const csrfProtection = csrf({ cookie: true });
// Set up rate limiting
const registerLimiter = rateLimit({
windowMs: 60 * 60 * 1000, // 1 hour
max: 5, // 5 requests per hour
message: 'Too many registration attempts, please try again later'
});
// Registration route with validation
app.post('/register',
csrfProtection,
registerLimiter,
[
// Username validation
body('username')
.isAlphanumeric().withMessage('Username must contain only letters and numbers')
.isLength({ min: 3, max: 20 }).withMessage('Username must be between 3 and 20 characters')
.trim().escape(),
// Email validation
body('email')
.isEmail().withMessage('Please enter a valid email address')
.normalizeEmail()
.custom(async value => {
// Check if email already exists in database
const existingUser = await db.findUserByEmail(value);
if (existingUser) {
throw new Error('Email already in use');
}
return true;
}),
// Password validation
body('password')
.isLength({ min: 8 }).withMessage('Password must be at least 8 characters long')
.matches(/[A-Z]/).withMessage('Password must contain at least one uppercase letter')
.matches(/[a-z]/).withMessage('Password must contain at least one lowercase letter')
.matches(/[0-9]/).withMessage('Password must contain at least one number')
.matches(/[^A-Za-z0-9]/).withMessage('Password must contain at least one special character')
],
async (req, res) => {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({
errors: errors.array().map(err => ({ field: err.param, message: err.msg }))
});
}
try {
const { username, email, password } = req.body;
// Hash the password
const hashedPassword = await bcrypt.hash(password, 12);
// Store user in database
await db.query(
'INSERT INTO users (username, email, password) VALUES (?, ?, ?)',
[username, email, hashedPassword]
);
res.status(201).json({ message: 'User registered successfully' });
} catch (error) {
console.error('Registration error:', error);
res.status(500).json({ message: 'An error occurred during registration' });
}
}
);
2. Type-Safe API Parameters
Instead of: “Create an API endpoint to fetch product details by ID”
Use this prompt:
Create a secure API endpoint to fetch product details by ID with robust input validation. Implement the following security measures:
1. Validate that the product ID is a positive integer using a validation library
2. Use parameterized queries to prevent SQL injection
3. Implement proper error handling that doesn't expose sensitive information
4. Return appropriate HTTP status codes for different scenarios (404 for product not found, 400 for invalid input)
5. Add input sanitization to prevent XSS attacks in the response
6. Implement rate limiting to prevent abuse
7. Add proper logging for security events
8. Use try-catch blocks to handle unexpected errors gracefully
Make sure the endpoint is RESTful and follows best practices for API design.
This prompt will guide Bolt to generate more secure code like:
const express = require('express');
const { param, validationResult } = require('express-validator');
const rateLimit = require('express-rate-limit');
const app = express();
// Set up rate limiting
const apiLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // 100 requests per 15 minutes
message: 'Too many requests from this IP, please try again later'
});
// Product details endpoint with validation
app.get('/api/products/:id',
apiLimiter,
[
// Validate product ID is a positive integer
param('id')
.isInt({ min: 1 }).withMessage('Product ID must be a positive integer')
.toInt()
],
async (req, res) => {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({
status: 'error',
errors: errors.array().map(err => ({ field: err.param, message: err.msg }))
});
}
const productId = req.params.id;
try {
// Use parameterized query to prevent SQL injection
const [product] = await db.query(
'SELECT id, name, description, price, image_url FROM products WHERE id = ?',
[productId]
);
// Check if product exists
if (!product) {
return res.status(404).json({
status: 'error',
message: 'Product not found'
});
}
// Log successful access
console.log(`Product ${productId} accessed at ${new Date().toISOString()}`);
// Return product details
return res.status(200).json({
status: 'success',
data: {
product
}
});
} catch (error) {
// Log error for investigation
console.error(`Error fetching product ${productId}:`, error);
// Return generic error message to client
return res.status(500).json({
status: 'error',
message: 'An error occurred while fetching the product'
});
}
}
);
3. Form Data Validation
Instead of: “Create a contact form with name, email, and message fields”
Use this prompt:
Create a secure contact form with comprehensive validation for name, email, and message fields. Implement the following security measures:
1. Use a validation library like Zod or express-validator for server-side validation
2. Validate that the name field contains only letters, spaces, and common name characters (like hyphens and apostrophes)
3. Validate that the email is in a proper format and consider checking MX records
4. Validate that the message has a reasonable length (between 10-1000 characters) and doesn't contain suspicious patterns
5. Implement CSRF protection for the form
6. Add rate limiting to prevent spam
7. Sanitize all inputs to prevent XSS and other injection attacks
8. Implement honeypot fields to catch automated submissions
9. Add proper error handling and user feedback
10. Log validation failures for security monitoring
Include both client-side and server-side validation, with the understanding that server-side validation is the security boundary.
This prompt will guide Bolt to generate more secure code like:
const express = require('express');
const { body, validationResult } = require('express-validator');
const csrf = require('csurf');
const rateLimit = require('express-rate-limit');
const { sanitizeHtml } = require('sanitize-html');
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: true }));
// Set up CSRF protection
const csrfProtection = csrf({ cookie: true });
// Set up rate limiting
const contactLimiter = rateLimit({
windowMs: 60 * 60 * 1000, // 1 hour
max: 5, // 5 contact submissions per hour
message: 'Too many contact attempts, please try again later'
});
// Contact form submission route with validation
app.post('/contact',
csrfProtection,
contactLimiter,
[
// Name validation
body('name')
.isLength({ min: 2, max: 50 }).withMessage('Name must be between 2 and 50 characters')
.matches(/^[a-zA-Z\s\-']+$/).withMessage('Name contains invalid characters')
.trim().escape(),
// Email validation
body('email')
.isEmail().withMessage('Please enter a valid email address')
.normalizeEmail(),
// Message validation
body('message')
.isLength({ min: 10, max: 1000 }).withMessage('Message must be between 10 and 1000 characters')
.trim(),
// Honeypot field - should be empty
body('website').custom(value => {
if (value) {
throw new Error('Spam submission detected');
}
return true;
})
],
async (req, res) => {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
// Log validation failures for security monitoring
console.warn('Contact form validation failure:', {
time: new Date().toISOString(),
ip: req.ip,
errors: errors.array()
});
return res.status(400).json({
success: false,
errors: errors.array().map(err => ({ field: err.param, message: err.msg }))
});
}
try {
const { name, email, message } = req.body;
// Sanitize inputs to prevent XSS
const sanitizedName = sanitizeHtml(name, {
allowedTags: [],
allowedAttributes: {}
});
const sanitizedMessage = sanitizeHtml(message, {
allowedTags: [],
allowedAttributes: {}
});
// Store contact submission in database
await db.query(
'INSERT INTO contact_submissions (name, email, message, submission_date, ip_address) VALUES (?, ?, ?, NOW(), ?)',
[sanitizedName, email, sanitizedMessage, req.ip]
);
// Log successful submission
console.log(`Contact form submitted by ${sanitizedName} (${email}) at ${new Date().toISOString()}`);
// Send success response
res.status(200).json({
success: true,
message: 'Your message has been sent successfully. We will contact you soon!'
});
} catch (error) {
console.error('Contact form submission error:', error);
res.status(500).json({
success: false,
message: 'An error occurred while submitting your message. Please try again later.'
});
}
}
);
Best Practices for Input Validation in Bolt Applications
1. Use Validation Libraries
Instead of writing custom validation logic, leverage established validation libraries:
// Using Zod for validation
const z = require('zod');
const userSchema = z.object({
username: z.string()
.min(3, 'Username must be at least 3 characters')
.max(20, 'Username cannot exceed 20 characters')
.regex(/^[a-zA-Z0-9]+$/, 'Username can only contain letters and numbers'),
email: z.string()
.email('Invalid email address'),
password: z.string()
.min(8, 'Password must be at least 8 characters')
.regex(/[A-Z]/, 'Password must contain at least one uppercase letter')
.regex(/[a-z]/, 'Password must contain at least one lowercase letter')
.regex(/[0-9]/, 'Password must contain at least one number')
.regex(/[^A-Za-z0-9]/, 'Password must contain at least one special character')
});
// Using the schema for validation
app.post('/register', (req, res) => {
try {
const validatedData = userSchema.parse(req.body);
// Process validated data...
} catch (error) {
res.status(400).json({ errors: error.errors });
}
});
2. Implement Defense in Depth
Don’t rely on a single layer of validation:
// Multiple layers of validation
function processUserInput(input) {
// Layer 1: Type checking
if (typeof input !== 'string') {
throw new Error('Input must be a string');
}
// Layer 2: Length validation
if (input.length < 1 || input.length > 100) {
throw new Error('Input must be between 1 and 100 characters');
}
// Layer 3: Content validation
if (!/^[a-zA-Z0-9\s]+$/.test(input)) {
throw new Error('Input contains invalid characters');
}
// Layer 4: Sanitization
const sanitizedInput = input.trim().replace(/\s+/g, ' ');
// Layer 5: Context-specific validation
if (isReservedKeyword(sanitizedInput)) {
throw new Error('Input cannot be a reserved keyword');
}
return sanitizedInput;
}
3. Validate on the Server Side
Always implement server-side validation, even if client-side validation is present:
// Client-side validation (for user experience)
document.getElementById('registrationForm').addEventListener('submit', function(event) {
const password = document.getElementById('password').value;
const passwordError = document.getElementById('passwordError');
if (password.length < 8) {
passwordError.textContent = 'Password must be at least 8 characters';
event.preventDefault();
return;
}
// More client-side validation...
});
// Server-side validation (for security)
app.post('/register', [
body('password')
.isLength({ min: 8 }).withMessage('Password must be at least 8 characters')
.matches(/[A-Z]/).withMessage('Password must contain at least one uppercase letter')
// More validation rules...
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Process valid registration...
});
4. Validate Data Types and Ranges
Ensure inputs are of the expected type and within acceptable ranges:
// Validating numeric input
app.get('/api/products', [
query('page')
.optional()
.isInt({ min: 1 }).withMessage('Page must be a positive integer')
.toInt(),
query('limit')
.optional()
.isInt({ min: 1, max: 100 }).withMessage('Limit must be between 1 and 100')
.toInt()
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const page = req.query.page || 1;
const limit = req.query.limit || 20;
// Fetch products with pagination...
});
5. Implement Contextual Validation
Different fields may require different validation rules based on context:
// Contextual validation for different user roles
app.post('/users', [
body('role')
.isIn(['user', 'admin', 'editor']).withMessage('Invalid role'),
body('adminCode')
.custom((value, { req }) => {
// Only validate adminCode if role is 'admin'
if (req.body.role === 'admin') {
if (!value || value !== process.env.ADMIN_SECRET_CODE) {
throw new Error('Invalid admin code');
}
}
return true;
})
], (req, res) => {
// Process user creation...
});
Conclusion
Input validation is a fundamental security measure that should be implemented in all Bolt applications. By using specific, security-focused prompts, you can guide Bolt to generate more secure code that properly validates and sanitizes user inputs, protecting your application from a wide range of attacks.
Remember that security is not a one-time implementation but an ongoing process. Regularly review and update your input validation practices to address emerging threats and vulnerabilities. By combining the efficiency of vibe coding with robust security practices, you can build applications that are both innovative and secure.
The prompts provided in this article serve as a starting point for securing your Bolt applications against input validation vulnerabilities. Adapt them to your specific requirements and continue to educate yourself on the latest web security best practices. With diligence and attention to security details, your Bolt applications can provide both rapid development and robust protection against one of the most common and dangerous web vulnerabilities.