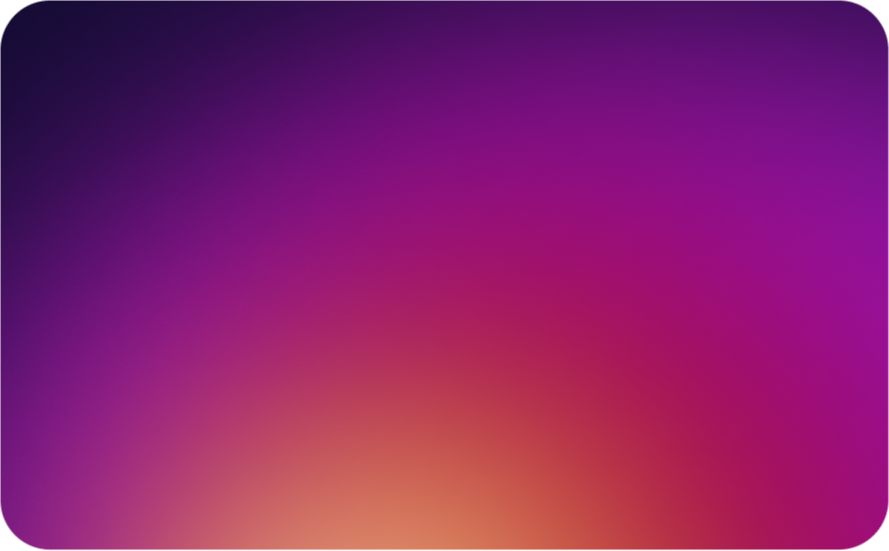
OAuth Implementation Security for Lovable Applications
OAuth Implementation Security for Lovable Applications
In the rapidly evolving landscape of vibe coding, Lovable has emerged as a powerful platform that enables developers to create sophisticated applications with minimal effort. However, as applications built with Lovable often handle sensitive user data and integrate with third-party services, implementing secure authentication and authorization becomes critical. OAuth 2.0 is the industry standard for authorization, but implementing it securely in Lovable applications requires careful consideration of best practices and potential vulnerabilities.
This article explores the essential security considerations for implementing OAuth 2.0 in Lovable applications. We’ll examine common vulnerabilities, provide practical prompts to generate secure OAuth implementations, and outline best practices to ensure your Lovable-built applications maintain robust security while leveraging the power of OAuth 2.0.
Understanding OAuth 2.0 Security Challenges in Lovable
Lovable’s vibe coding approach simplifies application development, but this simplification can sometimes lead to security oversights when implementing OAuth 2.0. The platform’s focus on rapid development might inadvertently encourage developers to use default configurations or simplified implementations that don’t adhere to security best practices.
Common OAuth implementation vulnerabilities in Lovable applications include:
1. Insecure Redirect URI Handling
Lovable’s default OAuth implementation might not properly validate redirect URIs, potentially allowing attackers to exploit open redirectors:
// Vulnerable Lovable-generated OAuth implementation
app.get('/oauth/callback', (req, res) => {
const code = req.query.code;
const redirectUri = req.query.redirect_uri || '/dashboard';
// Vulnerability: No validation of redirect_uri
exchangeCodeForToken(code)
.then(tokens => {
// Store tokens in session
req.session.accessToken = tokens.access_token;
req.session.refreshToken = tokens.refresh_token;
// Redirect to potentially malicious URI
res.redirect(redirectUri);
})
.catch(error => {
res.redirect('/login?error=' + error.message);
});
});
This code is vulnerable because it accepts a redirect URI from a query parameter without validation, potentially allowing attackers to redirect users to malicious sites after authentication.
2. Improper Token Storage
Lovable applications might store OAuth tokens insecurely by default:
// Vulnerable token storage in Lovable application
function storeTokens(tokens) {
// Vulnerability: Storing tokens in localStorage
localStorage.setItem('access_token', tokens.access_token);
localStorage.setItem('refresh_token', tokens.refresh_token);
localStorage.setItem('id_token', tokens.id_token);
}
Storing tokens in localStorage makes them vulnerable to cross-site scripting (XSS) attacks, as any JavaScript running on the page can access them.
3. Missing PKCE Implementation
Lovable might generate OAuth code that doesn’t implement PKCE (Proof Key for Code Exchange), which is essential for securing the authorization code flow, especially for single-page applications:
// Vulnerable OAuth initialization without PKCE
const oauthConfig = {
client_id: 'your-client-id',
redirect_uri: 'https://your-app.com/callback',
response_type: 'code',
scope: 'openid profile email'
};
// Vulnerability: No PKCE implementation
function initiateLogin() {
const authUrl = `https://auth-server.com/authorize?${new URLSearchParams(oauthConfig)}`;
window.location.href = authUrl;
}
Without PKCE, the authorization code flow is vulnerable to code interception attacks, particularly for public clients like browser-based applications.
Prompts to Fix OAuth Security Issues in Lovable
1. Secure Redirect URI Handling
Instead of: “Create an OAuth callback handler for my Lovable application”
Use this prompt:
Create a secure OAuth callback handler for my Lovable application with the following security measures:
1. Implement strict redirect URI validation that:
- Uses an allowlist of pre-configured redirect URIs
- Performs exact string matching (not pattern matching)
- Rejects any redirect URIs not in the allowlist
- Never accepts redirect URIs from query parameters
2. Implement proper CSRF protection using:
- A state parameter with a cryptographically secure random value
- Server-side validation of the state parameter
- Secure binding of the state to the user's session
3. Include comprehensive error handling that:
- Doesn't expose sensitive information in error messages
- Logs security events for monitoring
- Provides user-friendly error messages
The implementation should follow OAuth 2.0 security best practices and be resistant to common OAuth redirect attacks.
This prompt will guide Lovable to generate more secure code like:
// Server-side implementation
const crypto = require('crypto');
const { URL } = require('url');
// Predefined allowlist of redirect URIs
const ALLOWED_REDIRECT_URIS = [
'https://your-app.com/dashboard',
'https://your-app.com/profile',
'https://your-app.com/home'
];
// Express middleware for OAuth callback
app.get('/oauth/callback', async (req, res) => {
try {
// 1. Validate required parameters
const { code, state } = req.query;
if (!code || !state) {
throw new Error('Missing required parameters');
}
// 2. Validate state parameter to prevent CSRF
const savedState = req.session.oauthState;
const savedStateExpiry = req.session.oauthStateExpiry;
if (!savedState || savedState !== state) {
throw new Error('Invalid state parameter');
}
// Check if state is expired (15 minutes max lifetime)
if (!savedStateExpiry || savedStateExpiry < Date.now()) {
throw new Error('State parameter expired');
}
// Clear state from session to prevent replay
delete req.session.oauthState;
delete req.session.oauthStateExpiry;
// 3. Exchange code for tokens
const tokenResponse = await exchangeCodeForToken(
code,
process.env.OAUTH_CLIENT_ID,
process.env.OAUTH_CLIENT_SECRET,
process.env.OAUTH_REDIRECT_URI
);
// 4. Store tokens securely in server-side session
req.session.accessToken = tokenResponse.access_token;
if (tokenResponse.refresh_token) {
req.session.refreshToken = tokenResponse.refresh_token;
}
// 5. Validate and use redirect URI from session (not from query params)
const redirectUri = req.session.postAuthRedirect || '/dashboard';
delete req.session.postAuthRedirect;
if (!ALLOWED_REDIRECT_URIS.includes(redirectUri)) {
// Default to a safe URI if the stored one isn't allowed
return res.redirect('/dashboard');
}
// 6. Redirect to the validated URI
return res.redirect(redirectUri);
} catch (error) {
// Log error for monitoring but don't expose details to user
console.error('OAuth callback error:', error);
// Redirect to login with generic error
return res.redirect('/login?error=authentication_failed');
}
});
// Function to initiate OAuth flow
app.get('/login', (req, res) => {
// Generate cryptographically secure random state
const state = crypto.randomBytes(32).toString('hex');
// Store state in session with expiry (15 minutes)
req.session.oauthState = state;
req.session.oauthStateExpiry = Date.now() + (15 * 60 * 1000);
// Store post-authentication redirect (if provided and valid)
const { redirect } = req.query;
if (redirect && ALLOWED_REDIRECT_URIS.includes(redirect)) {
req.session.postAuthRedirect = redirect;
}
// Construct authorization URL with state parameter
const authUrl = new URL('https://auth-server.com/authorize');
authUrl.searchParams.append('client_id', process.env.OAUTH_CLIENT_ID);
authUrl.searchParams.append('redirect_uri', process.env.OAUTH_REDIRECT_URI);
authUrl.searchParams.append('response_type', 'code');
authUrl.searchParams.append('scope', 'openid profile email');
authUrl.searchParams.append('state', state);
// Redirect to authorization server
res.redirect(authUrl.toString());
});
// Function to exchange code for tokens
async function exchangeCodeForToken(code, clientId, clientSecret, redirectUri) {
// Implementation of token exchange
// ...
}
2. Secure Token Storage and Management
Instead of: “Add OAuth token storage to my Lovable SPA”
Use this prompt:
Create a secure OAuth token management system for my Lovable single-page application with the following security measures:
1. Implement secure token storage that:
- Never stores tokens in localStorage or sessionStorage
- Uses HttpOnly cookies for refresh tokens
- Keeps access tokens in memory only during the session
- Implements proper token renewal before expiration
2. Add token security features including:
- Access token validation (signature, expiration, audience)
- Automatic token refresh mechanism
- Secure logout that properly invalidates tokens
- Protection against token leakage
3. Include proper error handling and monitoring for:
- Failed token validations
- Refresh failures
- Potential security incidents
The implementation should follow OAuth 2.0 security best practices and be resistant to XSS and other common token theft attacks.
This prompt will guide Lovable to generate more secure code like:
// Token management service for a Lovable SPA
class TokenService {
constructor() {
// Store access token in memory only (not in localStorage)
this.accessToken = null;
this.tokenExpiry = null;
this.refreshing = null;
// Set up automatic token refresh
this.setupTokenRefresh();
// Listen for tab/window close to clear memory
window.addEventListener('beforeunload', () => {
this.clearTokens();
});
}
// Store tokens securely after login
setTokens(tokenResponse) {
// Store access token in memory only
this.accessToken = tokenResponse.access_token;
// Calculate token expiry time (with 5-minute buffer)
const expiresIn = tokenResponse.expires_in || 3600;
this.tokenExpiry = Date.now() + (expiresIn * 1000) - (5 * 60 * 1000);
// Refresh token is handled by the backend and stored in HttpOnly cookie
// We don't store it in the frontend at all
// Setup automatic refresh based on expiry
this.setupTokenRefresh();
// Notify app that authentication state changed
this.notifyAuthStateChange(true);
}
// Get the current access token, refreshing if needed
async getAccessToken() {
// If no token exists, user is not logged in
if (!this.accessToken) {
return null;
}
// If token is expired or close to expiry, refresh it
if (this.tokenExpiry <= Date.now()) {
try {
await this.refreshAccessToken();
} catch (error) {
console.error('Token refresh failed:', error);
// If refresh fails, user needs to log in again
this.logout();
return null;
}
}
return this.accessToken;
}
// Refresh the access token using the refresh token in HttpOnly cookie
async refreshAccessToken() {
// Prevent multiple simultaneous refresh requests
if (this.refreshing) {
return this.refreshing;
}
try {
this.refreshing = fetch('/api/auth/refresh', {
method: 'POST',
credentials: 'include', // Include cookies
headers: {
'Content-Type': 'application/json'
}
}).then(async (response) => {
if (!response.ok) {
throw new Error('Token refresh failed');
}
const tokenResponse = await response.json();
this.setTokens(tokenResponse);
return this.accessToken;
});
return await this.refreshing;
} catch (error) {
console.error('Error refreshing token:', error);
this.clearTokens();
throw error;
} finally {
this.refreshing = null;
}
}
// Set up automatic token refresh
setupTokenRefresh() {
// Clear any existing refresh timer
if (this.refreshTimer) {
clearTimeout(this.refreshTimer);
}
// If we have a valid token and expiry time
if (this.accessToken && this.tokenExpiry) {
// Calculate time until refresh (with 5-minute buffer)
const refreshTime = this.tokenExpiry - Date.now();
if (refreshTime > 0) {
// Set timer to refresh token before it expires
this.refreshTimer = setTimeout(() => {
this.refreshAccessToken().catch(error => {
console.error('Automatic token refresh failed:', error);
// If automatic refresh fails, user needs to log in again
this.logout();
});
}, refreshTime);
} else {
// Token is already expired, refresh immediately
this.refreshAccessToken().catch(error => {
console.error('Token refresh failed:', error);
this.logout();
});
}
}
}
// Securely log out
async logout() {
try {
// Call backend to invalidate the refresh token and clear the cookie
await fetch('/api/auth/logout', {
method: 'POST',
credentials: 'include'
});
} catch (error) {
console.error('Error during logout:', error);
} finally {
// Clear tokens from memory regardless of server response
this.clearTokens();
}
}
// Clear all token data from memory
clearTokens() {
this.accessToken = null;
this.tokenExpiry = null;
if (this.refreshTimer) {
clearTimeout(this.refreshTimer);
this.refreshTimer = null;
}
// Notify app that authentication state changed
this.notifyAuthStateChange(false);
}
// Check if user is authenticated
isAuthenticated() {
return !!this.accessToken && this.tokenExpiry > Date.now();
}
// Notify app of authentication state changes
notifyAuthStateChange(isAuthenticated) {
const event = new CustomEvent('authStateChanged', {
detail: { isAuthenticated }
});
window.dispatchEvent(event);
}
}
// Backend API for token refresh (Node.js/Express)
app.post('/api/auth/refresh', async (req, res) => {
try {
// Get refresh token from HttpOnly cookie
const refreshToken = req.cookies.refresh_token;
if (!refreshToken) {
return res.status(401).json({ error: 'No refresh token' });
}
// Exchange refresh token for new access token
const tokenResponse = await refreshAccessToken(refreshToken);
// Set new refresh token as HttpOnly cookie
res.cookie('refresh_token', tokenResponse.refresh_token, {
httpOnly: true,
secure: process.env.NODE_ENV === 'production',
sameSite: 'strict',
maxAge: 30 * 24 * 60 * 60 * 1000 // 30 days
});
// Return new access token to client
return res.json({
access_token: tokenResponse.access_token,
expires_in: tokenResponse.expires_in
});
} catch (error) {
console.error('Refresh token error:', error);
// Clear the invalid refresh token
res.clearCookie('refresh_token');
return res.status(401).json({ error: 'Invalid refresh token' });
}
});
// Logout endpoint to invalidate refresh token
app.post('/api/auth/logout', (req, res) => {
// Clear refresh token cookie
res.clearCookie('refresh_token');
// Optionally, invalidate the refresh token on the authorization server
// ...
return res.json({ success: true });
});
3. Implementing PKCE for Single-Page Applications
Instead of: “Add OAuth login to my Lovable SPA”
Use this prompt:
Create a secure OAuth implementation for my Lovable single-page application that uses the Authorization Code flow with PKCE (Proof Key for Code Exchange) with the following security measures:
1. Implement PKCE with:
- Cryptographically secure code_verifier generation
- SHA-256 code_challenge method
- Secure storage of code_verifier during authorization
- Proper code exchange with verification
2. Add additional security features:
- State parameter for CSRF protection
- Proper scope limitations
- Audience restriction for tokens
- Secure token handling after authentication
3. Include comprehensive error handling for:
- Authorization failures
- Token exchange errors
- Validation failures
The implementation should follow OAuth 2.0 security best practices and be suitable for a public client (browser-based SPA) according to current IETF recommendations.
This prompt will guide Lovable to generate more secure code like:
// OAuth service with PKCE for Lovable SPA
class OAuthService {
constructor(config) {
this.config = {
authorizationEndpoint: config.authorizationEndpoint,
tokenEndpoint: config.tokenEndpoint,
clientId: config.clientId,
redirectUri: config.redirectUri,
scope: config.scope || 'openid profile email',
audience: config.audience,
autoRefresh: config.autoRefresh !== false
};
// Initialize token service for secure token management
this.tokenService = new TokenService();
}
// Generate cryptographically secure random string
generateRandomString(length = 64) {
const array = new Uint8Array(length);
window.crypto.getRandomValues(array);
return Array.from(array, byte => byte.toString(16).padStart(2, '0')).join('');
}
// Generate code challenge from verifier using SHA-256
async generateCodeChallenge(codeVerifier) {
const encoder = new TextEncoder();
const data = encoder.encode(codeVerifier);
const digest = await window.crypto.subtle.digest('SHA-256', data);
return btoa(String.fromCharCode(...new Uint8Array(digest)))
.replace(/\+/g, '-')
.replace(/\//g, '_')
.replace(/=+$/, '');
}
// Initiate login with PKCE
async login() {
try {
// Generate and store PKCE code_verifier
const codeVerifier = this.generateRandomString(128);
sessionStorage.setItem('code_verifier', codeVerifier);
// Generate code_challenge from code_verifier
const codeChallenge = await this.generateCodeChallenge(codeVerifier);
// Generate and store state for CSRF protection
const state = this.generateRandomString(32);
sessionStorage.setItem('oauth_state', state);
// Store current time for state expiry check
sessionStorage.setItem('oauth_state_time', Date.now().toString());
// Build authorization URL with PKCE and state parameters
const authUrl = new URL(this.config.authorizationEndpoint);
authUrl.searchParams.append('client_id', this.config.clientId);
authUrl.searchParams.append('redirect_uri', this.config.redirectUri);
authUrl.searchParams.append('response_type', 'code');
authUrl.searchParams.append('scope', this.config.scope);
authUrl.searchParams.append('code_challenge', codeChallenge);
authUrl.searchParams.append('code_challenge_method', 'S256');
authUrl.searchParams.append('state', state);
// Add audience if specified
if (this.config.audience) {
authUrl.searchParams.append('audience', this.config.audience);
}
// Redirect to authorization server
window.location.href = authUrl.toString();
} catch (error) {
console.error('Login initialization error:', error);
throw new Error('Failed to initialize login process');
}
}
// Handle authorization callback
async handleCallback() {
try {
// Parse URL parameters
const urlParams = new URLSearchParams(window.location.search);
const code = urlParams.get('code');
const state = urlParams.get('state');
const error = urlParams.get('error');
// Handle authorization errors
if (error) {
throw new Error(`Authorization error: ${error}`);
}
// Verify required parameters
if (!code || !state) {
throw new Error('Missing required parameters');
}
// Retrieve stored state and code_verifier
const storedState = sessionStorage.getItem('oauth_state');
const stateTime = parseInt(sessionStorage.getItem('oauth_state_time') || '0', 10);
const codeVerifier = sessionStorage.getItem('code_verifier');
// Clear sensitive data from sessionStorage
sessionStorage.removeItem('oauth_state');
sessionStorage.removeItem('oauth_state_time');
sessionStorage.removeItem('code_verifier');
// Validate state parameter to prevent CSRF
if (!storedState || storedState !== state) {
throw new Error('Invalid state parameter');
}
// Check if state is expired (15 minutes max lifetime)
const stateAge = Date.now() - stateTime;
if (stateAge > 15 * 60 * 1000) {
throw new Error('State parameter expired');
}
// Verify code_verifier exists
if (!codeVerifier) {
throw new Error('Missing code_verifier');
}
// Exchange code for tokens using PKCE
const tokenResponse = await this.exchangeCodeForTokens(code, codeVerifier);
// Store tokens securely
this.tokenService.setTokens(tokenResponse);
// Clean up URL to remove code and state
const cleanUrl = window.location.pathname;
window.history.replaceState({}, document.title, cleanUrl);
return tokenResponse;
} catch (error) {
console.error('Authorization callback error:', error);
throw error;
}
}
// Exchange authorization code for tokens
async exchangeCodeForTokens(code, codeVerifier) {
try {
const tokenRequest = {
grant_type: 'authorization_code',
client_id: this.config.clientId,
code_verifier: codeVerifier,
code: code,
redirect_uri: this.config.redirectUri
};
const response = await fetch(this.config.tokenEndpoint, {
method: 'POST',
headers: {
'Content-Type': 'application/x-www-form-urlencoded'
},
body: new URLSearchParams(tokenRequest)
});
if (!response.ok) {
const errorData = await response.json().catch(() => ({}));
throw new Error(errorData.error_description || 'Token exchange failed');
}
return await response.json();
} catch (error) {
console.error('Token exchange error:', error);
throw error;
}
}
// Check if user is authenticated
isAuthenticated() {
return this.tokenService.isAuthenticated();
}
// Get access token for API calls
async getAccessToken() {
return this.tokenService.getAccessToken();
}
// Logout user
async logout() {
await this.tokenService.logout();
// Optionally redirect to authorization server logout endpoint
if (this.config.logoutEndpoint) {
window.location.href = this.config.logoutEndpoint;
}
}
}
// Usage in a Lovable SPA
document.addEventListener('DOMContentLoaded', async () => {
const oauthService = new OAuthService({
authorizationEndpoint: 'https://auth-server.com/authorize',
tokenEndpoint: 'https://auth-server.com/oauth/token',
clientId: 'YOUR_CLIENT_ID',
redirectUri: 'https://your-app.com/callback',
scope: 'openid profile email api:access',
audience: 'https://api.your-service.com'
});
// Check if this is a callback from the authorization server
if (window.location.search.includes('code=')) {
try {
await oauthService.handleCallback();
updateUI(true);
} catch (error) {
console.error('Authentication error:', error);
showError('Authentication failed. Please try again.');
updateUI(false);
}
} else {
// Check if user is already authenticated
updateUI(oauthService.isAuthenticated());
}
// Set up login button
document.getElementById('loginButton').addEventListener('click', () => {
oauthService.login().catch(error => {
console.error('Login error:', error);
showError('Failed to initialize login. Please try again.');
});
});
// Set up logout button
document.getElementById('logoutButton').addEventListener('click', () => {
oauthService.logout().catch(error => {
console.error('Logout error:', error);
});
updateUI(false);
});
// Function to update UI based on authentication state
function updateUI(isAuthenticated) {
document.getElementById('loginButton').style.display = isAuthenticated ? 'none' : 'block';
document.getElementById('logoutButton').style.display = isAuthenticated ? 'block' : 'none';
document.getElementById('protectedContent').style.display = isAuthenticated ? 'block' : 'none';
}
// Function to show error messages
function showError(message) {
const errorElement = document.getElementById('errorMessage');
errorElement.textContent = message;
errorElement.style.display = 'block';
// Hide error after 5 seconds
setTimeout(() => {
errorElement.style.display = 'none';
}, 5000);
}
});
Best Practices for OAuth Implementation in Lovable
1. Use Authorization Code Flow with PKCE
Always use the Authorization Code flow with PKCE for Lovable single-page applications. The Implicit flow is deprecated due to security concerns:
// Example of proper PKCE implementation in Lovable
async function generateCodeVerifierAndChallenge() {
// Generate code verifier
const codeVerifier = generateRandomString(128);
// Generate code challenge using SHA-256
const encoder = new TextEncoder();
const data = encoder.encode(codeVerifier);
const digest = await window.crypto.subtle.digest('SHA-256', data);
const codeChallenge = btoa(String.fromCharCode(...new Uint8Array(digest)))
.replace(/\+/g, '-')
.replace(/\//g, '_')
.replace(/=+$/, '');
return { codeVerifier, codeChallenge };
}
2. Implement Proper Token Storage
Store tokens securely in Lovable applications:
// Example of secure token storage in Lovable
class SecureTokenStorage {
// Store access token in memory only
#accessToken = null;
#tokenExpiry = null;
setAccessToken(token, expiresIn) {
this.#accessToken = token;
this.#tokenExpiry = Date.now() + (expiresIn * 1000);
}
getAccessToken() {
if (this.#tokenExpiry && this.#tokenExpiry < Date.now()) {
// Token expired
this.clearTokens();
return null;
}
return this.#accessToken;
}
clearTokens() {
this.#accessToken = null;
this.#tokenExpiry = null;
}
}
3. Validate Tokens Properly
Always validate tokens on both client and server:
// Example of token validation in Lovable
async function validateAccessToken(token) {
try {
// For JWT tokens
const tokenParts = token.split('.');
if (tokenParts.length !== 3) {
return false;
}
// Decode payload
const payload = JSON.parse(atob(tokenParts[1]));
// Check expiration
if (!payload.exp || payload.exp * 1000 < Date.now()) {
return false;
}
// Check audience
if (!payload.aud || payload.aud !== 'your-api-audience') {
return false;
}
// In production, also verify signature with jwks-rsa or similar
return true;
} catch (error) {
console.error('Token validation error:', error);
return false;
}
}
4. Implement Proper Error Handling
Handle OAuth errors gracefully in Lovable applications:
// Example of OAuth error handling in Lovable
function handleOAuthError(error) {
// Log error for monitoring
console.error('OAuth error:', error);
// Map error types to user-friendly messages
const errorMessages = {
'invalid_request': 'The OAuth request was invalid. Please try again.',
'unauthorized_client': 'This application is not authorized to use this authentication method.',
'access_denied': 'You denied access to the application.',
'unsupported_response_type': 'The authentication server does not support this type of request.',
'invalid_scope': 'The requested scope is invalid or unknown.',
'server_error': 'The authentication server encountered an error. Please try again later.',
'temporarily_unavailable': 'The authentication service is temporarily unavailable. Please try again later.'
};
// Get user-friendly message or use generic fallback
const userMessage = errorMessages[error] || 'Authentication failed. Please try again.';
// Display error to user
showErrorNotification(userMessage);
// Redirect to appropriate page based on error
if (error === 'access_denied') {
navigateTo('/login');
} else {
navigateTo('/error');
}
}
5. Implement Secure Logout
Ensure proper logout in Lovable applications:
// Example of secure logout in Lovable
async function secureLogout() {
try {
// 1. Clear tokens from memory
tokenService.clearTokens();
// 2. Clear any session cookies via backend
await fetch('/api/auth/logout', {
method: 'POST',
credentials: 'include'
});
// 3. Optionally redirect to IdP logout endpoint
const logoutUrl = new URL('https://auth-server.com/logout');
logoutUrl.searchParams.append('client_id', 'your-client-id');
logoutUrl.searchParams.append('returnTo', window.location.origin);
window.location.href = logoutUrl.toString();
} catch (error) {
console.error('Logout error:', error);
// Still clear local tokens even if server logout fails
tokenService.clearTokens();
window.location.href = '/';
}
}
Conclusion
Implementing OAuth 2.0 securely in Lovable applications requires careful attention to best practices and potential vulnerabilities. By using the prompts provided in this article, you can guide Lovable to generate more secure OAuth implementations that protect your users and their data.
Remember that security is an ongoing process, not a one-time implementation. Regularly review your OAuth implementation for security updates and best practices, especially as the OAuth standards and Lovable platform evolve.
By combining the efficiency of vibe coding with robust security practices, you can build Lovable applications that are both innovative and secure, providing your users with a seamless and safe authentication experience.