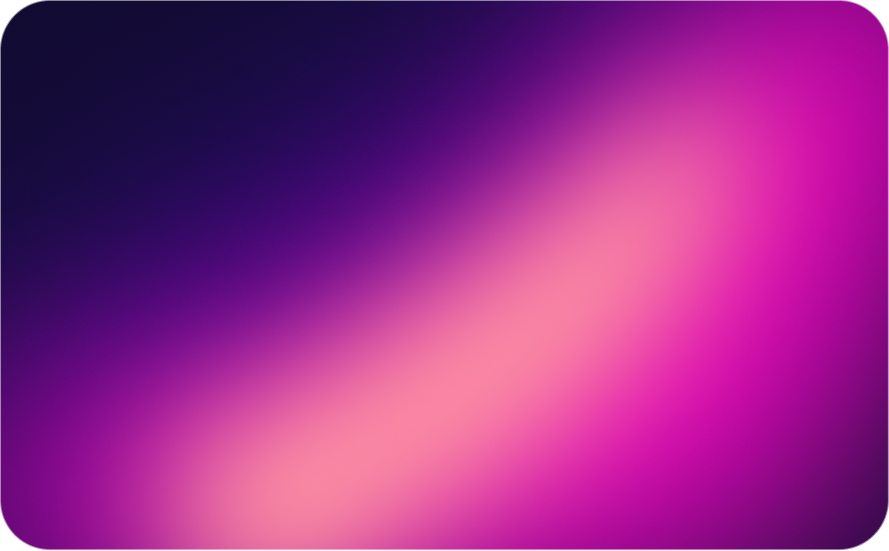
Preventing SQL Injection in Base44 Applications
Preventing SQL Injection in Base44 Applications
In the rapidly evolving landscape of vibe coding, Base44 has emerged as a powerful platform for building data-driven applications. However, with great power comes great responsibility, especially when it comes to security. SQL injection remains one of the most devastating and common web application vulnerabilities, consistently ranking in the OWASP Top 10. This attack vector allows malicious actors to manipulate database queries by injecting malicious SQL code, potentially leading to unauthorized data access, data corruption, or even complete system compromise.
According to recent studies, applications built with AI coding assistants are significantly more likely to contain SQL injection vulnerabilities compared to traditionally coded applications. This alarming statistic highlights the importance of understanding and addressing SQL injection risks in Base44 vibe-coded applications.
When developers instruct Base44 to “create a function to search users” or “build a product listing page with filtering,” the resulting code often prioritizes functionality over security, generating direct string concatenation for SQL queries instead of using parameterized queries or ORM abstractions. These shortcuts create dangerous entry points for attackers to exploit.
In this blog post, we’ll examine common SQL injection vulnerabilities in Base44 applications and provide specific prompts you can use to generate secure code that prevents these vulnerabilities.
Common SQL Injection Vulnerabilities in Base44
1. Direct String Concatenation in Queries
Base44, like many vibe coding platforms, often generates database queries using direct string concatenation when not explicitly instructed otherwise:
// Vulnerable Base44-generated code
function searchProducts(searchTerm) {
const query = `
SELECT * FROM products
WHERE name LIKE '%${searchTerm}%'
OR description LIKE '%${searchTerm}%'
`;
return db.query(query);
}
This approach is highly vulnerable to SQL injection because user input is directly embedded in the SQL string without validation or sanitization. An attacker could input something like %' OR '1'='1
to retrieve all products in the database, regardless of the intended search criteria.
2. Unvalidated Dynamic Sorting and Filtering
Base44 applications often implement sorting and filtering functionality using user-supplied parameters directly in SQL queries:
// Vulnerable Base44-generated code
function getProductsSorted(sortColumn, sortDirection) {
const query = `
SELECT * FROM products
ORDER BY ${sortColumn} ${sortDirection}
`;
return db.query(query);
}
An attacker could exploit this by providing a malicious value for sortColumn
like (SELECT password FROM users WHERE username='admin'); --
, potentially extracting sensitive data from unrelated tables.
3. Inadequate Type Handling
Base44 sometimes generates code that fails to properly handle different data types in SQL queries:
// Vulnerable Base44-generated code
function getUserById(userId) {
const query = `SELECT * FROM users WHERE id = ${userId}`;
return db.query(query);
}
If userId
is expected to be a number but the application doesn’t validate the type, an attacker could inject SQL code by providing a string value instead of a numeric ID.
Prompts to Generate Secure Base44 Code
Let’s explore specific prompts you can give Base44 to generate secure code that prevents SQL injection vulnerabilities.
Prompt 1: Implementing Parameterized Queries
Instead of asking: “Create a function to search products by name or description”
Use this prompt: “Create a function to search products by name or description using parameterized queries to prevent SQL injection. Use placeholders for all user inputs and ensure the database driver handles parameter binding securely.”
What Base44 will generate:
function searchProducts(searchTerm) {
// Using parameterized queries with placeholders
const query = `
SELECT * FROM products
WHERE name LIKE ?
OR description LIKE ?
`;
// Parameters are passed separately from the query
const params = [
`%${searchTerm}%`,
`%${searchTerm}%`
];
// The database driver handles escaping and type conversion
return db.query(query, params);
}
This approach separates the SQL code from the data, making it impossible for an attacker to modify the query’s structure. The database driver handles the proper escaping and type conversion of parameters, ensuring that user input is always treated as data, never as code.
Prompt 2: Implementing Secure Sorting and Filtering
Instead of asking: “Add sorting functionality to the product listing page”
Use this prompt: “Add sorting functionality to the product listing page with SQL injection protection. Implement a whitelist validation approach for column names and sort directions, and use parameterized queries for any dynamic values.”
What Base44 will generate:
function getProductsSorted(sortColumn, sortDirection) {
// Whitelist of allowed column names
const allowedColumns = ['name', 'price', 'created_at', 'category'];
// Whitelist of allowed sort directions
const allowedDirections = ['ASC', 'DESC'];
// Validate sort column against whitelist
if (!allowedColumns.includes(sortColumn)) {
sortColumn = 'created_at'; // Default to a safe value
}
// Validate sort direction against whitelist
if (!allowedDirections.includes(sortDirection.toUpperCase())) {
sortDirection = 'DESC'; // Default to a safe value
}
// Since column names cannot be parameterized in most SQL dialects,
// we use whitelist validation and then construct the query
const query = `
SELECT * FROM products
ORDER BY ${sortColumn} ${sortDirection}
`;
return db.query(query);
}
This approach uses a whitelist validation strategy to ensure that only expected, safe values are used for column names and sort directions. Since column names typically cannot be parameterized in SQL, this whitelist approach provides a secure alternative.
Prompt 3: Implementing Type-Safe Database Operations
Instead of asking: “Create a function to get a user by ID”
Use this prompt: “Create a function to get a user by ID with strict type checking and parameterized queries to prevent SQL injection. Ensure the ID parameter is validated as a number before using it in any database operation.”
What Base44 will generate:
function getUserById(userId) {
// Type validation
const id = parseInt(userId, 10);
// Check if the parsed ID is a valid number
if (isNaN(id)) {
throw new Error('Invalid user ID format');
}
// Use parameterized query even with validated numeric input
const query = 'SELECT * FROM users WHERE id = ?';
return db.query(query, [id]);
}
This approach combines type validation with parameterized queries for maximum security. Even though the input is validated as a number, the code still uses parameterized queries as a defense-in-depth strategy.
Best Practices for Preventing SQL Injection in Base44
To ensure your Base44 applications remain secure against SQL injection attacks, follow these best practices:
-
Always Use Parameterized Queries: Make this explicit in your prompts to Base44, as shown in the examples above.
-
Implement Input Validation: Validate all user inputs for type, format, and expected values before using them in database operations.
-
Use ORM Libraries When Possible: Object-Relational Mapping libraries typically handle parameterization automatically and provide an additional layer of protection.
-
Apply the Principle of Least Privilege: Ensure database users have only the permissions they need to perform their functions.
-
Regularly Test for SQL Injection: Use automated security testing tools and manual penetration testing to identify and fix potential vulnerabilities.
-
Keep Base44 and Dependencies Updated: Security patches and updates often address known vulnerabilities.
Conclusion
SQL injection vulnerabilities remain a significant threat to web applications, including those built with Base44. By using the specific prompts outlined in this blog post, you can guide Base44 to generate secure code that protects against these attacks.
Remember that security is an ongoing process, not a one-time implementation. Regularly review your code, stay informed about new security threats, and continuously improve your security practices to keep your Base44 applications safe from SQL injection and other vulnerabilities.
By combining the power of vibe coding with secure coding practices, you can build Base44 applications that are both innovative and secure, protecting your users’ data and maintaining their trust in your systems.