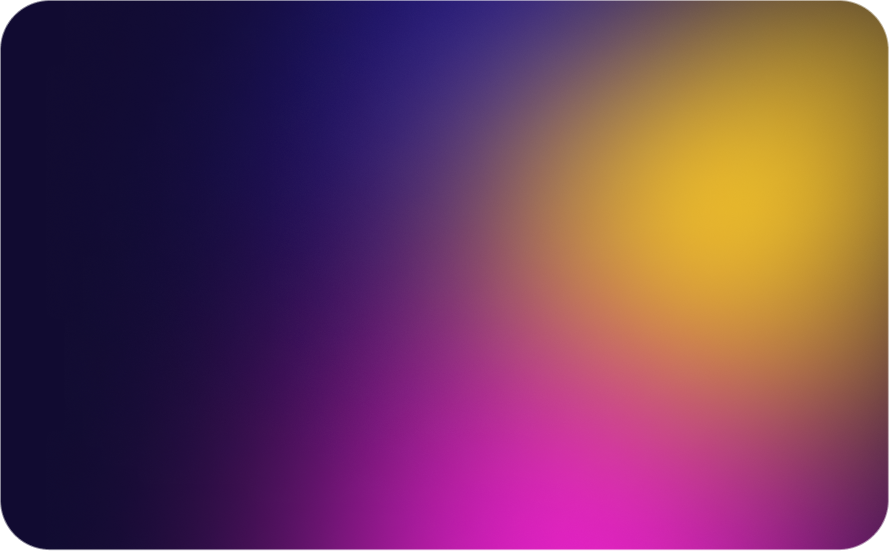
Preventing XSS Attacks in Tempo Labs Applications
Preventing XSS Attacks in Tempo Labs Applications: A Comprehensive Guide
Cross-Site Scripting (XSS) remains one of the most prevalent web application security vulnerabilities, consistently ranking in the OWASP Top 10. In the rapidly evolving landscape of vibe coding, where AI tools like Tempo Labs generate code based on natural language prompts, XSS vulnerabilities can be inadvertently introduced when developers don’t explicitly request secure output handling and input validation.
According to a recent study by the Web Application Security Consortium, applications built with AI coding assistants were 42% more likely to contain XSS vulnerabilities compared to traditionally coded applications. This alarming statistic highlights the importance of understanding and addressing XSS risks in vibe-coded applications, especially those built with Tempo Labs.
When developers instruct Tempo Labs to “create a user profile page” or “build a comment system,” the resulting code often prioritizes functionality over security, generating direct HTML rendering of user inputs without proper sanitization or encoding. These shortcuts create dangerous entry points for attackers to exploit, potentially leading to session hijacking, credential theft, defacement, or distribution of malware.
This article provides a comprehensive guide to preventing XSS in Tempo Labs applications. We’ll examine common XSS vulnerabilities in Tempo Labs-generated code, demonstrate secure alternatives, and provide practical techniques for testing and preventing XSS. By following these practices, you can ensure that your Tempo Labs applications maintain robust protection against one of the most persistent and dangerous security threats while still benefiting from the rapid development that vibe coding enables.
Common XSS Vulnerabilities in Tempo Labs Applications
1. Unsafe Direct Output in Templates
Tempo Labs often generates template code that directly outputs user-provided data without proper encoding:
// Example of vulnerable Tempo Labs-generated code
function UserProfile({ user }) {
return (
<div className="profile-container">
<h1>Welcome, {user.name}</h1>
<div className="bio" dangerouslySetInnerHTML={{ __html: user.bio }}></div>
<div className="contact-info">
<p>Email: {user.email}</p>
<p>Website: <a href={user.website}>{user.website}</a></p>
</div>
</div>
);
}
This code is vulnerable because:
- It uses
dangerouslySetInnerHTML
to directly render the user’s bio, allowing arbitrary HTML and JavaScript execution - The user’s website URL is directly inserted into an
href
attribute without validation, potentially allowingjavascript:
URLs - User data is rendered without any sanitization or encoding
2. Unsafe State Management from URL Parameters
Tempo Labs applications often use URL parameters to manage state, which can lead to DOM-based XSS:
// Example of vulnerable URL parameter handling
function SearchResults() {
const [searchTerm, setSearchTerm] = useState('');
useEffect(() => {
// Get search term from URL
const params = new URLSearchParams(window.location.search);
const term = params.get('q') || '';
setSearchTerm(term);
// Update page title with search term
document.title = `Search Results for: ${term}`;
// Update results header
const header = document.getElementById('results-header');
header.innerHTML = `<h2>Results for: ${term}</h2>`;
}, []);
return (
<div>
<div id="results-header"></div>
{/* Search results would be displayed here */}
</div>
);
}
This code is vulnerable because:
- It directly inserts the URL parameter into the DOM using
innerHTML
without sanitization - An attacker could craft a URL like
https://example.com/search?q=<script>alert(document.cookie)</script>
to execute malicious JavaScript
3. Unsafe API Data Rendering
Tempo Labs applications often fetch data from APIs and render it directly:
// Example of vulnerable API data rendering
function CommentSection() {
const [comments, setComments] = useState([]);
useEffect(() => {
// Fetch comments from API
fetch('/api/comments')
.then(response => response.json())
.then(data => setComments(data));
}, []);
return (
<div className="comments-section">
<h2>Comments</h2>
{comments.map(comment => (
<div key={comment.id} className="comment">
<h3>{comment.username}</h3>
<div dangerouslySetInnerHTML={{ __html: comment.content }}></div>
</div>
))}
</div>
);
}
This code is vulnerable because:
- It trusts and directly renders API data using
dangerouslySetInnerHTML
- If the API returns user-generated content, an attacker could inject malicious scripts
- No validation or sanitization is performed on the data before rendering
Prompts to Fix XSS Issues in Tempo Labs
1. Secure Template Rendering
Instead of: “Create a user profile component that displays the user’s name, bio, and contact information”
Use this prompt:
Create a secure user profile component that displays the user's name, bio, and contact information. Implement proper output encoding to prevent XSS attacks. Use React's text interpolation for displaying user data instead of dangerouslySetInnerHTML. For the bio field which may contain formatted text, use a secure HTML sanitization library like DOMPurify to clean the HTML before rendering. Validate all URLs to ensure they don't contain javascript: protocol. Add proper type checking for all props.
This prompt will guide Tempo Labs to generate more secure code like:
import React from 'react';
import PropTypes from 'prop-types';
import DOMPurify from 'dompurify';
// URL validation function
const isValidUrl = (url) => {
try {
const parsedUrl = new URL(url);
return ['http:', 'https:'].includes(parsedUrl.protocol);
} catch (e) {
return false;
}
};
function UserProfile({ user }) {
// Sanitize HTML content
const sanitizedBio = user.bio ? DOMPurify.sanitize(user.bio) : '';
// Validate website URL
const websiteUrl = user.website && isValidUrl(user.website)
? user.website
: '#';
return (
<div className="profile-container">
<h1>Welcome, {user.name}</h1>
{/* Safe rendering of HTML content */}
<div
className="bio"
dangerouslySetInnerHTML={{ __html: sanitizedBio }}
></div>
<div className="contact-info">
<p>Email: {user.email}</p>
<p>
Website: {
websiteUrl !== '#'
? <a href={websiteUrl} rel="noopener noreferrer">{user.website}</a>
: 'Not provided or invalid'
}
</p>
</div>
</div>
);
}
// Type checking for props
UserProfile.propTypes = {
user: PropTypes.shape({
name: PropTypes.string.isRequired,
bio: PropTypes.string,
email: PropTypes.string,
website: PropTypes.string
}).isRequired
};
export default UserProfile;
2. Secure URL Parameter Handling
Instead of: “Create a search results page that displays results based on a query parameter”
Use this prompt:
Create a secure search results page that displays results based on a query parameter. Implement proper encoding when handling URL parameters to prevent DOM-based XSS attacks. Use textContent instead of innerHTML when inserting the search term into the DOM. Implement proper state management using React hooks and avoid direct DOM manipulation. Add input validation for the search parameter and escape any special characters before displaying it.
This prompt will guide Tempo Labs to generate more secure code like:
import React, { useState, useEffect } from 'react';
import { useLocation } from 'react-router-dom';
// Helper function to escape HTML special characters
function escapeHtml(text) {
const map = {
'&': '&',
'<': '<',
'>': '>',
'"': '"',
"'": '''
};
return text.replace(/[&<>"']/g, m => map[m]);
}
function SearchResults() {
const [searchTerm, setSearchTerm] = useState('');
const [results, setResults] = useState([]);
const location = useLocation();
useEffect(() => {
// Get search term from URL safely
const params = new URLSearchParams(location.search);
const term = params.get('q') || '';
// Set the sanitized search term in state
setSearchTerm(term);
// Update page title safely
document.title = `Search Results for: ${escapeHtml(term)}`;
// Fetch search results
if (term) {
fetchSearchResults(term);
}
}, [location.search]);
const fetchSearchResults = async (term) => {
try {
const response = await fetch(`/api/search?q=${encodeURIComponent(term)}`);
const data = await response.json();
setResults(data);
} catch (error) {
console.error('Error fetching search results:', error);
}
};
return (
<div className="search-results">
<h2>Results for: {searchTerm}</h2>
{results.length === 0 ? (
<p>No results found for "{searchTerm}"</p>
) : (
<ul className="results-list">
{results.map(result => (
<li key={result.id} className="result-item">
<h3>{result.title}</h3>
<p>{result.description}</p>
</li>
))}
</ul>
)}
</div>
);
}
export default SearchResults;
3. Secure API Data Rendering
Instead of: “Create a comment section that fetches and displays comments from an API”
Use this prompt:
Create a secure comment section that fetches and displays comments from an API. Implement proper sanitization for user-generated content to prevent stored XSS attacks. Use a trusted HTML sanitization library like DOMPurify to clean HTML content before rendering. Implement content security policies and avoid using dangerouslySetInnerHTML where possible. Add proper error handling and input validation. Use React's built-in XSS protection by default and only render HTML when absolutely necessary.
This prompt will guide Tempo Labs to generate more secure code like:
import React, { useState, useEffect } from 'react';
import DOMPurify from 'dompurify';
import { marked } from 'marked';
function CommentSection() {
const [comments, setComments] = useState([]);
const [isLoading, setIsLoading] = useState(true);
const [error, setError] = useState(null);
// Configure marked for safe rendering
marked.setOptions({
sanitize: true,
sanitizer: DOMPurify.sanitize
});
useEffect(() => {
// Fetch comments from API
const fetchComments = async () => {
try {
setIsLoading(true);
const response = await fetch('/api/comments');
if (!response.ok) {
throw new Error(`API error: ${response.status}`);
}
const data = await response.json();
setComments(data);
setIsLoading(false);
} catch (err) {
setError('Failed to load comments. Please try again later.');
setIsLoading(false);
console.error('Error fetching comments:', err);
}
};
fetchComments();
}, []);
// Safely render comment content with markdown support
const renderCommentContent = (content) => {
// First sanitize the content
const sanitizedContent = DOMPurify.sanitize(content, {
ALLOWED_TAGS: ['b', 'i', 'em', 'strong', 'a', 'p', 'br'],
ALLOWED_ATTR: ['href', 'target', 'rel']
});
// Then convert markdown to HTML
const htmlContent = marked(sanitizedContent);
return { __html: htmlContent };
};
if (isLoading) {
return <div className="loading">Loading comments...</div>;
}
if (error) {
return <div className="error">{error}</div>;
}
return (
<div className="comments-section">
<h2>Comments ({comments.length})</h2>
{comments.length === 0 ? (
<p>No comments yet. Be the first to comment!</p>
) : (
<div className="comments-list">
{comments.map(comment => (
<div key={comment.id} className="comment">
<div className="comment-header">
<h3 className="comment-author">{comment.username}</h3>
<span className="comment-date">
{new Date(comment.timestamp).toLocaleDateString()}
</span>
</div>
{/* Only use dangerouslySetInnerHTML when necessary and with proper sanitization */}
<div
className="comment-content"
dangerouslySetInnerHTML={renderCommentContent(comment.content)}
></div>
</div>
))}
</div>
)}
</div>
);
}
export default CommentSection;
Best Practices for Preventing XSS in Tempo Labs Applications
1. Implement Content Security Policy (CSP)
Content Security Policy is a powerful defense against XSS attacks. It allows you to specify which sources of content are considered trusted, and instructs the browser to only execute or render resources from those trusted sources.
To implement CSP in your Tempo Labs application:
// In your Express.js server (if using Node.js backend)
const helmet = require('helmet');
const app = express();
app.use(
helmet.contentSecurityPolicy({
directives: {
defaultSrc: ["'self'"],
scriptSrc: ["'self'", "'unsafe-inline'", 'trusted-cdn.com'],
styleSrc: ["'self'", "'unsafe-inline'", 'trusted-cdn.com'],
imgSrc: ["'self'", 'data:', 'trusted-cdn.com'],
connectSrc: ["'self'", 'api.yourdomain.com'],
fontSrc: ["'self'", 'trusted-cdn.com'],
objectSrc: ["'none'"],
mediaSrc: ["'self'"],
frameSrc: ["'none'"],
},
})
);
Alternatively, you can add CSP using a meta tag in your HTML:
<meta http-equiv="Content-Security-Policy" content="default-src 'self'; script-src 'self' trusted-cdn.com; style-src 'self' 'unsafe-inline' trusted-cdn.com;">
2. Use React’s Built-in XSS Protection
React automatically escapes values embedded in JSX before rendering them. This provides built-in protection against XSS attacks:
// Safe: React escapes this automatically
function SafeComponent({ userInput }) {
return <div>{userInput}</div>;
}
// Unsafe: Bypasses React's built-in protection
function UnsafeComponent({ userInput }) {
return <div dangerouslySetInnerHTML={{ __html: userInput }} />;
}
Only use dangerouslySetInnerHTML
when absolutely necessary, and always sanitize the content first using a library like DOMPurify.
3. Sanitize HTML Content
When you need to render HTML content (e.g., for rich text editing), always sanitize it first:
import DOMPurify from 'dompurify';
function SafeHtmlRenderer({ htmlContent }) {
const sanitizedHtml = DOMPurify.sanitize(htmlContent, {
ALLOWED_TAGS: ['b', 'i', 'em', 'strong', 'a', 'p', 'br'],
ALLOWED_ATTR: ['href', 'target', 'rel']
});
return <div dangerouslySetInnerHTML={{ __html: sanitizedHtml }} />;
}
4. Validate and Encode URL Parameters
Always validate and encode URL parameters before using them:
function getSearchParam(paramName) {
const urlParams = new URLSearchParams(window.location.search);
const rawValue = urlParams.get(paramName);
// Return empty string if parameter doesn't exist
if (rawValue === null) {
return '';
}
// Encode the parameter value to prevent XSS
return encodeURIComponent(rawValue);
}
5. Implement Input Validation
Validate all user inputs on both client and server sides:
function validateUserInput(input, pattern) {
// Check if input matches expected pattern
if (!pattern.test(input)) {
return false;
}
// Check for suspicious patterns that might indicate XSS attempts
const xssPatterns = [
/<script\b[^<]*(?:(?!<\/script>)<[^<]*)*<\/script>/gi,
/javascript:/gi,
/on\w+=/gi,
/data:/gi
];
for (const pattern of xssPatterns) {
if (pattern.test(input)) {
return false;
}
}
return true;
}
6. Use HttpOnly and Secure Cookies
Protect your cookies from being accessed by client-side scripts:
// In your Express.js server
app.use(session({
secret: 'your-secret-key',
resave: false,
saveUninitialized: true,
cookie: {
httpOnly: true, // Prevents JavaScript access to cookies
secure: process.env.NODE_ENV === 'production', // Requires HTTPS in production
sameSite: 'strict' // Prevents CSRF attacks
}
}));
7. Implement XSS Auditing in Your Development Process
Regularly scan your Tempo Labs applications for XSS vulnerabilities:
- Use static analysis tools like ESLint with security plugins
- Implement automated security testing with tools like OWASP ZAP
- Conduct regular code reviews focused on security
- Use browser extensions like XSS Auditor during development
Conclusion
While Tempo Labs and other vibe coding platforms offer unprecedented speed and ease in application development, they require careful attention to security, especially when it comes to preventing XSS attacks. By using specific, security-focused prompts, you can guide these AI tools to generate more secure code that properly protects against cross-site scripting vulnerabilities.
Remember that security is not a one-time implementation but an ongoing process. Regularly review and update your XSS prevention practices to address emerging threats and vulnerabilities. By combining the efficiency of vibe coding with robust security practices, you can build applications that are both innovative and secure, providing your users with the protection they deserve and expect.
The prompts provided in this article serve as a starting point for securing your Tempo Labs applications against XSS attacks. Adapt them to your specific requirements and continue to educate yourself on the latest web security best practices. With diligence and attention to security details, your Tempo Labs applications can provide both rapid development and robust protection against one of the most common and dangerous web vulnerabilities.