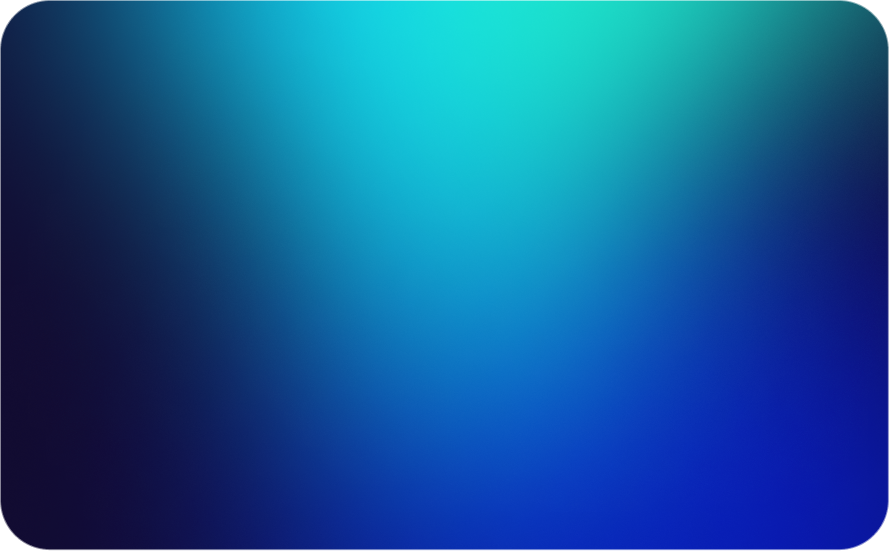
Secure File Storage Practices: Protecting Uploaded Files
Introduction
After implementing secure file upload validation, the next critical step is ensuring that stored files remain protected from unauthorized access and manipulation. Proper file storage security is essential for maintaining data confidentiality, integrity, and availability. This guide covers best practices for implementing secure file storage in your applications.
File System Permissions
Understanding File System Security
File system permissions are your first line of defense against unauthorized access:
- They control who can read, write, and execute files
- They prevent unauthorized modifications
- They protect sensitive data from exposure
- They isolate files between users
Implementing Secure Permissions
const fs = require('fs');
const path = require('path');
// Storage directories
const PRIVATE_STORAGE_DIR = path.join(__dirname, '../private-storage');
const TEMP_UPLOAD_DIR = path.join(__dirname, '../temp-uploads');
// Ensure directories exist with secure permissions
function initializeSecureStorage() {
// Create directories if they don't exist
[PRIVATE_STORAGE_DIR, TEMP_UPLOAD_DIR].forEach(dir => {
if (!fs.existsSync(dir)) {
// Create with restricted permissions (only owner can read/write)
fs.mkdirSync(dir, { recursive: true, mode: 0o700 });
} else {
// Update permissions on existing directory
fs.chmodSync(dir, 0o700);
}
});
}
// Create user-specific storage directory
async function createUserStorage(userId) {
const userDir = path.join(PRIVATE_STORAGE_DIR, userId.toString());
// Create directory with secure permissions
await fs.promises.mkdir(userDir, {
recursive: true,
mode: 0o700 // Only owner can read/write
});
return userDir;
}
// Set secure permissions on uploaded file
async function secureFile(filePath) {
try {
// Set file permissions to owner read/write only
await fs.promises.chmod(filePath, 0o600);
return true;
} catch (error) {
console.error('Failed to secure file:', error);
return false;
}
}
Permission Best Practices
-
Set restrictive base permissions:
- Use principle of least privilege
- Restrict directory listings
- Prevent execute permissions
- Isolate user files
-
Implement proper ownership:
- Run application with dedicated user
- Set appropriate group permissions
- Maintain permission hierarchy
- Regular permission audits
-
Handle permission errors:
- Log permission issues
- Monitor access attempts
- Alert on permission changes
- Regular security audits
Encryption at Rest
Importance of Data Encryption
Encryption protects files from:
- Unauthorized access
- Data breaches
- Physical storage theft
- Insider threats
Implementing File Encryption
const crypto = require('crypto');
const fs = require('fs');
const path = require('path');
class FileEncryption {
constructor(encryptionKey) {
this.algorithm = 'aes-256-gcm';
this.key = crypto.scryptSync(encryptionKey, 'salt', 32);
}
async encryptFile(sourcePath, destinationPath) {
try {
// Generate initialization vector
const iv = crypto.randomBytes(16);
// Create cipher
const cipher = crypto.createCipheriv(this.algorithm, this.key, iv);
// Create read and write streams
const readStream = fs.createReadStream(sourcePath);
const writeStream = fs.createWriteStream(destinationPath);
// Write IV at the beginning of the file
writeStream.write(iv);
// Encrypt file
readStream.pipe(cipher).pipe(writeStream);
return new Promise((resolve, reject) => {
writeStream.on('finish', () => {
resolve(true);
});
writeStream.on('error', (error) => {
reject(error);
});
});
} catch (error) {
throw new Error(`Encryption failed: ${error.message}`);
}
}
async decryptFile(sourcePath, destinationPath) {
try {
// Read the file
const data = await fs.promises.readFile(sourcePath);
// Extract IV from the beginning of the file
const iv = data.slice(0, 16);
const encryptedData = data.slice(16);
// Create decipher
const decipher = crypto.createDecipheriv(this.algorithm, this.key, iv);
// Decrypt file
const decrypted = Buffer.concat([
decipher.update(encryptedData),
decipher.final()
]);
// Write decrypted file
await fs.promises.writeFile(destinationPath, decrypted);
return true;
} catch (error) {
throw new Error(`Decryption failed: ${error.message}`);
}
}
}
// Example usage
const encryption = new FileEncryption(process.env.ENCRYPTION_KEY);
app.post('/upload', upload.single('file'), async (req, res) => {
try {
const file = req.file;
if (!file) {
return res.status(400).json({ error: 'No file uploaded' });
}
// Generate secure filename
const encryptedFilename = `${crypto.randomBytes(32).toString('hex')}.enc`;
const encryptedPath = path.join(PRIVATE_STORAGE_DIR, encryptedFilename);
// Encrypt file
await encryption.encryptFile(file.path, encryptedPath);
// Remove original file
await fs.promises.unlink(file.path);
// Store file metadata
const fileMetadata = {
id: crypto.randomBytes(16).toString('hex'),
originalName: file.originalname,
encryptedName: encryptedFilename,
mimeType: file.mimetype,
size: file.size
};
// Return file reference
res.json({ fileId: fileMetadata.id });
} catch (error) {
console.error('Upload error:', error);
res.status(500).json({ error: 'File upload failed' });
}
});
Encryption Best Practices
-
Key management:
- Secure key storage
- Regular key rotation
- Backup key management
- Access control to keys
-
Algorithm selection:
- Use strong algorithms
- Proper IV generation
- Secure mode of operation
- Regular security updates
-
Performance considerations:
- Streaming for large files
- Caching strategies
- Resource monitoring
- Optimization techniques
Secure Temporary Files
Managing Temporary Storage
Temporary files require special attention:
- They may contain sensitive data
- They can be targeted for attacks
- They need proper cleanup
- They require secure permissions
Implementing Secure Temporary Storage
const os = require('os');
const fs = require('fs');
const path = require('path');
const crypto = require('crypto');
class SecureTempStorage {
constructor() {
this.tempDir = path.join(os.tmpdir(), 'secure-uploads');
this.createTempDir();
}
createTempDir() {
if (!fs.existsSync(this.tempDir)) {
fs.mkdirSync(this.tempDir, { mode: 0o700 });
}
}
async createTempFile() {
const tempFilename = crypto.randomBytes(32).toString('hex');
const tempPath = path.join(this.tempDir, tempFilename);
// Create empty file with secure permissions
const fd = await fs.promises.open(tempPath, 'w', 0o600);
await fd.close();
return tempPath;
}
async cleanup(filePath) {
try {
if (fs.existsSync(filePath)) {
// Overwrite file with random data
const size = (await fs.promises.stat(filePath)).size;
const fd = await fs.promises.open(filePath, 'w');
const buffer = crypto.randomBytes(size);
await fd.write(buffer);
await fd.close();
// Delete file
await fs.promises.unlink(filePath);
}
} catch (error) {
console.error('Cleanup error:', error);
}
}
async cleanupAll() {
try {
const files = await fs.promises.readdir(this.tempDir);
await Promise.all(files.map(file =>
this.cleanup(path.join(this.tempDir, file))
));
} catch (error) {
console.error('Cleanup all error:', error);
}
}
}
// Example usage
const tempStorage = new SecureTempStorage();
app.post('/upload', upload.single('file'), async (req, res) => {
let tempPath = null;
try {
const file = req.file;
if (!file) {
return res.status(400).json({ error: 'No file uploaded' });
}
// Create temporary file
tempPath = await tempStorage.createTempFile();
// Process file...
// Cleanup temporary file
await tempStorage.cleanup(tempPath);
res.json({ message: 'File processed successfully' });
} catch (error) {
console.error('Upload error:', error);
// Ensure cleanup on error
if (tempPath) {
await tempStorage.cleanup(tempPath);
}
res.status(500).json({ error: 'File upload failed' });
}
});
// Cleanup on application shutdown
process.on('SIGINT', async () => {
await tempStorage.cleanupAll();
process.exit(0);
});
Temporary File Best Practices
-
Secure creation:
- Use random names
- Set proper permissions
- Isolate temporary storage
- Monitor usage
-
Proper cleanup:
- Immediate deletion
- Secure overwrite
- Error handling
- Scheduled cleanup
-
Resource management:
- Size limitations
- Quota enforcement
- Monitoring
- Automatic cleanup
Directory Traversal Prevention
Understanding Path Traversal
Directory traversal attacks can:
- Access unauthorized files
- Modify system files
- Expose sensitive data
- Compromise security
Implementing Path Protection
const path = require('path');
const fs = require('fs');
class SecureFileAccess {
constructor(baseDir) {
this.baseDir = path.resolve(baseDir);
}
isPathSafe(filePath) {
// Resolve full path
const fullPath = path.resolve(this.baseDir, filePath);
// Check if path is within base directory
return fullPath.startsWith(this.baseDir);
}
async readFile(filePath) {
if (!this.isPathSafe(filePath)) {
throw new Error('Invalid file path');
}
const fullPath = path.resolve(this.baseDir, filePath);
return fs.promises.readFile(fullPath);
}
async writeFile(filePath, data) {
if (!this.isPathSafe(filePath)) {
throw new Error('Invalid file path');
}
const fullPath = path.resolve(this.baseDir, filePath);
return fs.promises.writeFile(fullPath, data);
}
async deleteFile(filePath) {
if (!this.isPathSafe(filePath)) {
throw new Error('Invalid file path');
}
const fullPath = path.resolve(this.baseDir, filePath);
return fs.promises.unlink(fullPath);
}
}
// Example usage
const fileAccess = new SecureFileAccess(PRIVATE_STORAGE_DIR);
app.get('/files/:filename', async (req, res) => {
try {
const filename = req.params.filename;
// Attempt to read file
const data = await fileAccess.readFile(filename);
res.send(data);
} catch (error) {
if (error.message === 'Invalid file path') {
res.status(400).json({ error: 'Invalid file path' });
} else {
res.status(500).json({ error: 'Failed to read file' });
}
}
});
Path Protection Best Practices
-
Input validation:
- Normalize paths
- Validate characters
- Check boundaries
- Whitelist approach
-
Access control:
- Base directory restriction
- User isolation
- Permission checks
- Audit logging
-
Error handling:
- Secure error messages
- Proper logging
- Access monitoring
- Incident response
Conclusion
Implementing secure file storage is crucial for protecting uploaded files and maintaining application security. By properly implementing file system permissions, encryption at rest, secure temporary file handling, and directory traversal prevention, you create a comprehensive security strategy for stored files.
Key takeaways:
- Use proper file system permissions
- Implement encryption for sensitive files
- Handle temporary files securely
- Prevent directory traversal attacks
- Monitor and audit file access