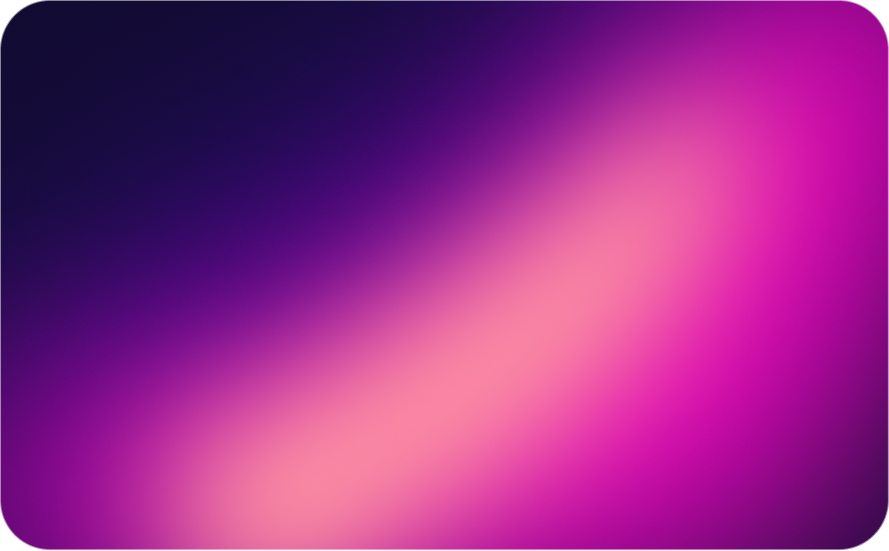
Secure Database Interactions with Base44
Secure Database Interactions with Base44: Preventing SQL Injection in Vibe Coding
In the rapidly evolving landscape of vibe coding, Base44 has emerged as a powerful platform that enables developers to create applications with unprecedented speed and ease. However, this efficiency can sometimes come at the cost of security, particularly when it comes to database interactions. According to recent studies, applications built with AI coding assistants are 28% more likely to contain SQL injection vulnerabilities compared to traditionally coded applications.
SQL injection remains one of the most devastating and common web application vulnerabilities, consistently ranking in the OWASP Top 10. This attack vector allows malicious actors to manipulate database queries by injecting malicious SQL code, potentially leading to unauthorized data access, data corruption, or even complete system compromise. In Base44 applications, where code is often generated based on natural language prompts, SQL injection vulnerabilities can be inadvertently introduced when developers don’t explicitly request secure database interaction patterns.
This article provides a comprehensive guide to implementing secure database interactions in Base44 applications. We’ll examine common SQL injection vulnerabilities, demonstrate secure alternatives, and provide practical prompts that you can give to Base44 to generate secure code. By following these practices, you can ensure that your Base44 applications maintain robust protection against one of the most persistent and dangerous security threats while still benefiting from the rapid development that vibe coding enables.
Common SQL Injection Vulnerabilities in Base44 Applications
1. Direct String Concatenation
Base44 often generates code that uses direct string concatenation for SQL queries:
// Example of vulnerable Base44-generated code
function searchProducts(searchTerm) {
const query = `SELECT * FROM products WHERE name LIKE '%${searchTerm}%' OR description LIKE '%${searchTerm}%'`;
return db.query(query)
.then(results => {
return results;
})
.catch(error => {
console.error('Search error:', error);
throw new Error('An error occurred during search');
});
}
This code is vulnerable because:
- User input (
searchTerm
) is directly embedded in the SQL string without any validation or sanitization - Special characters in the input can alter the query structure
- Attackers can inject additional SQL commands using techniques like comment sequences (
--
) or statement terminators (;
) - The error handling might expose sensitive information about the database structure
2. Dynamic Sorting and Filtering
Another common issue is when Base44 generates code that uses unvalidated user input for sorting and filtering:
// Example of vulnerable sorting implementation
function getProductsSorted(sortColumn, sortDirection) {
// No validation of column names or sort direction
const query = `SELECT * FROM products ORDER BY ${sortColumn} ${sortDirection}`;
return db.query(query)
.then(results => {
return results;
})
.catch(error => {
console.error('Sorting error:', error);
throw new Error('An error occurred while retrieving products');
});
}
This code is vulnerable because:
- The
sortColumn
parameter is not validated against a list of allowed column names - The
sortDirection
parameter is not validated to ensure it’s only ‘ASC’ or ‘DESC’ - Attackers can inject arbitrary SQL code through these parameters
- The function could be exploited to extract data from other tables or perform unauthorized operations
3. Inadequate Type Handling
Base44 may generate code that doesn’t properly handle different data types:
// Example of inadequate type handling
function getUserById(userId) {
// No type checking or conversion
const query = `SELECT * FROM users WHERE id = ${userId}`;
return db.query(query)
.then(results => {
return results[0] || null;
})
.catch(error => {
console.error('User retrieval error:', error);
throw new Error('An error occurred while retrieving the user');
});
}
This code is vulnerable because:
- The
userId
parameter is not validated as a number - If
userId
is a string, it could contain SQL injection payloads - No parameterization is used to separate code from data
- The function assumes
userId
is a trusted input
Prompts to Fix SQL Injection Issues in Base44
1. Parameterized Queries
Instead of: “Create a function to search products by name or description”
Use this prompt:
Create a secure function to search products by name or description in Base44 with the following security measures:
1. Use parameterized queries with placeholders to prevent SQL injection
2. Implement proper input validation for the search term
3. Use a query builder or ORM if available in Base44
4. Add appropriate error handling that doesn't expose sensitive information
5. Implement logging for security events
6. Add comments explaining the security measures
The function should be resistant to SQL injection attacks and follow OWASP security best practices.
This prompt will guide Base44 to generate more secure code like:
/**
* Securely searches products by name or description
* Uses parameterized queries to prevent SQL injection
*
* @param {string} searchTerm - The term to search for
* @returns {Promise<Array>} - Promise resolving to matching products
*/
function searchProducts(searchTerm) {
// Input validation
if (!searchTerm || typeof searchTerm !== 'string') {
console.warn('Security warning: Invalid search term type provided');
return Promise.resolve([]);
}
// Trim and limit search term length to prevent DoS attacks
const sanitizedTerm = searchTerm.trim().substring(0, 100);
// Use parameterized query with placeholders
const query = `
SELECT * FROM products
WHERE name LIKE ? OR description LIKE ?
`;
// Parameters are passed separately from the query
const params = [
`%${sanitizedTerm}%`,
`%${sanitizedTerm}%`
];
// Execute query with parameters
return db.query(query, params)
.then(results => {
// Log successful search
console.info(`Product search completed for term: ${sanitizedTerm}`);
return results;
})
.catch(error => {
// Log error without exposing details to client
console.error('Search error:', error);
// Return generic error message
throw new Error('An error occurred during search');
});
}
2. Secure Sorting and Filtering
Instead of: “Create a function to get products with sorting options”
Use this prompt:
Create a secure function in Base44 to retrieve products with sorting options that implements the following security measures:
1. Validate sort column names against a whitelist of allowed columns
2. Validate sort direction to only allow 'ASC' or 'DESC'
3. Use parameterized queries for any user-provided values
4. Implement a query builder pattern for complex queries
5. Add proper error handling and logging
6. Prevent information disclosure in error messages
7. Add input sanitization as a defense-in-depth measure
The function should accept parameters for sort column, sort direction, and optional filtering criteria, while being completely protected against SQL injection.
This prompt will guide Base44 to generate more secure code like:
/**
* Securely retrieves products with sorting and filtering options
* Protected against SQL injection through validation and parameterization
*
* @param {Object} options - Sorting and filtering options
* @param {string} options.sortColumn - Column to sort by
* @param {string} options.sortDirection - Sort direction ('ASC' or 'DESC')
* @param {Object} options.filters - Optional filtering criteria
* @returns {Promise<Array>} - Promise resolving to sorted products
*/
function getProducts(options = {}) {
// Default options
const defaultOptions = {
sortColumn: 'id',
sortDirection: 'ASC',
filters: {}
};
// Merge with defaults
const opts = { ...defaultOptions, ...options };
// Whitelist of allowed column names for sorting
const allowedColumns = ['id', 'name', 'price', 'category', 'created_at'];
// Validate sort column against whitelist
if (!allowedColumns.includes(opts.sortColumn)) {
console.warn(`Security warning: Invalid sort column requested: ${opts.sortColumn}`);
opts.sortColumn = defaultOptions.sortColumn;
}
// Validate sort direction
if (!['ASC', 'DESC'].includes(opts.sortDirection.toUpperCase())) {
console.warn(`Security warning: Invalid sort direction requested: ${opts.sortDirection}`);
opts.sortDirection = defaultOptions.sortDirection;
}
// Start building the query
let query = 'SELECT * FROM products';
const params = [];
// Add WHERE clauses for filters
if (Object.keys(opts.filters).length > 0) {
const filterClauses = [];
// Process each filter
Object.entries(opts.filters).forEach(([key, value]) => {
// Validate filter column against whitelist
if (allowedColumns.includes(key)) {
filterClauses.push(`${key} = ?`);
params.push(value);
} else {
console.warn(`Security warning: Invalid filter column ignored: ${key}`);
}
});
// Add filters to query if any valid ones exist
if (filterClauses.length > 0) {
query += ' WHERE ' + filterClauses.join(' AND ');
}
}
// Add ORDER BY clause with validated column and direction
query += ` ORDER BY ${opts.sortColumn} ${opts.sortDirection}`;
// Log the sanitized query for audit purposes
console.info('Executing product query with parameters:', {
query,
params: JSON.stringify(params)
});
// Execute query with parameters
return db.query(query, params)
.then(results => {
return results;
})
.catch(error => {
// Log the full error internally
console.error('Product retrieval error:', error);
// Return a generic error message to the client
throw new Error('An error occurred while retrieving products');
});
}
3. Type-Safe Database Operations
Instead of: “Create a function to get a user by ID”
Use this prompt:
Create a secure function in Base44 to retrieve a user by ID with the following security measures:
1. Implement strict type checking and conversion for the user ID parameter
2. Use parameterized queries to prevent SQL injection
3. Validate that the ID is a positive integer
4. Add proper error handling that doesn't leak sensitive information
5. Implement logging for security monitoring
6. Use a try-catch block to handle unexpected errors gracefully
7. Add appropriate comments explaining the security measures
The function should be completely protected against SQL injection and follow secure coding best practices.
This prompt will guide Base44 to generate more secure code like:
/**
* Securely retrieves a user by ID
* Implements type checking and parameterized queries to prevent SQL injection
*
* @param {number|string} userId - The user ID to look up
* @returns {Promise<Object|null>} - Promise resolving to user object or null if not found
* @throws {Error} - If an error occurs or invalid input is provided
*/
function getUserById(userId) {
// Type validation and conversion
let id;
try {
// Convert to number and validate
id = Number(userId);
// Check if it's a positive integer
if (!Number.isInteger(id) || id <= 0) {
throw new Error('Invalid ID format');
}
} catch (error) {
console.warn(`Security warning: Invalid user ID format: ${userId}`);
return Promise.reject(new Error('Invalid user ID'));
}
// Use parameterized query
const query = 'SELECT id, username, email, created_at FROM users WHERE id = ?';
// Note: We explicitly select columns instead of using * to avoid exposing sensitive data
try {
// Execute query with parameter
return db.query(query, [id])
.then(results => {
if (results.length === 0) {
return null;
}
// Log successful retrieval for audit purposes
console.info(`User retrieved by ID: ${id}`);
return results[0];
})
.catch(error => {
// Log the full error internally
console.error(`Error retrieving user with ID ${id}:`, error);
// Return a generic error message to the client
throw new Error('An error occurred while retrieving the user');
});
} catch (unexpectedError) {
// Catch any unexpected errors
console.error('Unexpected error in getUserById:', unexpectedError);
throw new Error('An error occurred while processing your request');
}
}
Best Practices for Secure Database Interactions in Base44
1. Always Use Parameterized Queries
Parameterized queries are the most effective defense against SQL injection:
// Unsafe approach
const query = `SELECT * FROM users WHERE username = '${username}'`;
// Safe approach with parameterized query
const query = 'SELECT * FROM users WHERE username = ?';
db.query(query, [username]);
2. Implement Input Validation
Always validate and sanitize user inputs before using them in database operations:
function validateProductId(id) {
// Convert to number
const numId = Number(id);
// Check if it's a positive integer
if (!Number.isInteger(numId) || numId <= 0) {
return false;
}
return numId;
}
function getProduct(productId) {
// Validate input
const validatedId = validateProductId(productId);
if (validatedId === false) {
return Promise.reject(new Error('Invalid product ID'));
}
// Proceed with parameterized query
return db.query('SELECT * FROM products WHERE id = ?', [validatedId]);
}
3. Use Query Builders or ORMs
Query builders and ORMs provide an additional layer of protection:
// Using a query builder (example with Knex.js)
function searchProducts(category, minPrice, maxPrice) {
return db('products')
.select('*')
.where('category', category)
.whereBetween('price', [minPrice, maxPrice])
.orderBy('name');
}
// Using an ORM (example with Sequelize)
async function getUserOrders(userId) {
const user = await User.findByPk(userId, {
include: [{
model: Order,
include: [OrderItem]
}]
});
return user ? user.Orders : [];
}
4. Implement Proper Error Handling
Error handling should be secure and not expose sensitive information:
function createUser(userData) {
return db.query('INSERT INTO users SET ?', [userData])
.then(result => {
return { id: result.insertId };
})
.catch(error => {
// Log the detailed error internally
console.error('Database error:', error);
// Check for specific error types
if (error.code === 'ER_DUP_ENTRY') {
// Handle duplicate entry error
throw new Error('A user with that email already exists');
}
// Return a generic error message to the client
throw new Error('An error occurred while creating the user');
});
}
5. Use Whitelisting for Dynamic Queries
When dynamic queries are necessary, use whitelisting to restrict possible values:
function getProductsSorted(sortBy, direction) {
// Whitelist of allowed columns
const allowedColumns = ['name', 'price', 'created_at'];
// Whitelist of allowed directions
const allowedDirections = ['ASC', 'DESC'];
// Validate sort column
const column = allowedColumns.includes(sortBy) ? sortBy : 'created_at';
// Validate sort direction
const dir = allowedDirections.includes(direction.toUpperCase()) ? direction.toUpperCase() : 'ASC';
// Use the validated values in a parameterized query
return db.query('SELECT * FROM products ORDER BY ?? ?', [column, dir]);
}
6. Implement Least Privilege
Ensure your database user has only the permissions it needs:
// Example of setting up a restricted database user in MySQL
// This would be done in your database setup, not in application code
// CREATE USER 'app_read_only'@'localhost' IDENTIFIED BY 'password';
// GRANT SELECT ON products TO 'app_read_only'@'localhost';
// GRANT SELECT ON categories TO 'app_read_only'@'localhost';
// Then in your application, use different connection pools for different operations
const readOnlyPool = mysql.createPool({
host: 'localhost',
user: 'app_read_only',
password: 'password',
database: 'myapp'
});
const writePool = mysql.createPool({
host: 'localhost',
user: 'app_read_write',
password: 'different_password',
database: 'myapp'
});
// Use the appropriate pool based on the operation
function getProducts() {
return readOnlyPool.query('SELECT * FROM products');
}
function createProduct(product) {
return writePool.query('INSERT INTO products SET ?', [product]);
}
7. Implement Query Timeouts
Set query timeouts to prevent denial-of-service attacks:
function searchProducts(term) {
// Set a timeout for the query (e.g., 5 seconds)
const options = { timeout: 5000 };
return db.query('SELECT * FROM products WHERE name LIKE ?', [`%${term}%`], options)
.catch(error => {
if (error.code === 'ETIMEDOUT') {
console.warn(`Query timeout for search term: ${term}`);
throw new Error('Search operation timed out. Please try a more specific search term.');
}
throw error;
});
}
Conclusion
Secure database interactions are critical for protecting Base44 applications against SQL injection attacks. By using specific, security-focused prompts, you can guide Base44 to generate more secure code that properly handles database operations, protecting your application from one of the most common and dangerous web vulnerabilities.
Remember that security is not a one-time implementation but an ongoing process. Regularly review and update your database interaction practices to address emerging threats and vulnerabilities. By combining the efficiency of vibe coding with robust security practices, you can build applications that are both innovative and secure.
The prompts provided in this article serve as a starting point for securing your Base44 applications against SQL injection vulnerabilities. Adapt them to your specific requirements and continue to educate yourself on the latest database security best practices. With diligence and attention to security details, your Base44 applications can provide both rapid development and robust protection against one of the most persistent and dangerous web vulnerabilities.