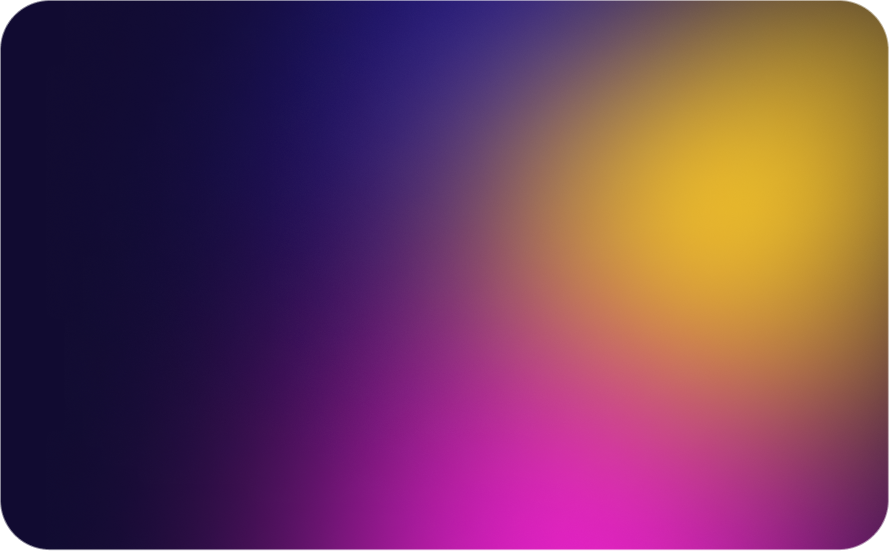
Secure Deployment Practices for Base44: A Comprehensive Guide
In the rapidly evolving landscape of vibe coding, Base44 has emerged as a powerful platform for developing applications quickly and efficiently. However, as with any development approach that prioritizes speed, security can sometimes take a backseat. This blog post explores essential secure deployment practices for Base44 applications, providing specific prompts to help you generate secure deployment configurations and processes.
Understanding the Deployment Security Challenges in Base44
Base44’s streamlined approach to application development makes it particularly attractive for rapid prototyping and MVP creation. However, this speed can introduce security vulnerabilities during the deployment phase if proper precautions aren’t taken.
Recent studies have shown that developers using AI assistants wrote less secure code in 80% of tasks compared to those coding manually, while being 3.5 times more likely to overestimate their code’s security. This risk is even more pronounced in vibe coding environments like Base44, where the focus is often on quick iteration rather than security hardening.
Common deployment security issues in Base44 applications include:
- Rushed End-to-End Deployment: Transitioning quickly from idea to production can bypass essential stages like testing and security review
- Insecure Default Configurations: Using default settings without proper security hardening
- Exposed Secrets and Credentials: Hardcoded API keys and credentials in deployment files
- Insufficient Access Controls: Lack of proper permission boundaries and role-based access
- Inadequate Monitoring and Logging: Missing visibility into application behavior post-deployment
Implementing Secure Deployment in Base44 Applications
Let’s explore how to implement robust deployment security in Base44 applications using specific prompts that will generate secure configurations and processes.
Prompt 1: Creating a Secure CI/CD Pipeline for Base44
Use this prompt to generate a secure continuous integration and deployment pipeline for your Base44 application:
Create a secure CI/CD pipeline configuration for my Base44 application that:
1. Implements security scanning at each stage of the pipeline
2. Includes dependency vulnerability scanning
3. Performs static code analysis to detect security issues
4. Implements infrastructure-as-code security validation
5. Ensures secrets are properly managed and not exposed
6. Includes post-deployment security verification
7. Provides clear documentation for the security measures implemented
This prompt will generate code similar to:
# secure-cicd-pipeline.yml for Base44 Application
name: Secure Base44 CI/CD Pipeline
on:
push:
branches: [ main, develop ]
pull_request:
branches: [ main, develop ]
jobs:
security-scan:
name: Security Scanning
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Setup Base44 environment
uses: base44-actions/setup@v2
with:
version: '1.5.0'
- name: Install dependencies
run: base44 install
- name: Dependency vulnerability scan
uses: snyk/actions/node@master
with:
args: --severity-threshold=medium
env:
SNYK_TOKEN: ${{ secrets.SNYK_TOKEN }}
- name: Static code analysis
uses: github/codeql-action/analyze@v2
with:
languages: javascript, typescript
- name: Secret scanning
uses: gitleaks/gitleaks-action@v2
build-and-test:
name: Build and Test
needs: security-scan
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Setup Base44 environment
uses: base44-actions/setup@v2
with:
version: '1.5.0'
- name: Build application
run: base44 build
- name: Run tests with security focus
run: base44 test --security
- name: Run integration tests
run: base44 test:integration
infrastructure-validation:
name: Infrastructure Validation
needs: build-and-test
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Validate infrastructure code
uses: terraform-linters/tfsec-action@v1
with:
directory: ./infrastructure
- name: Validate Kubernetes manifests
uses: kubescape/github-action@main
with:
files: ./k8s/*.yaml
format: sarif
secure-deployment:
name: Secure Deployment
needs: [security-scan, build-and-test, infrastructure-validation]
if: github.ref == 'refs/heads/main' && github.event_name == 'push'
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v3
- name: Setup Base44 environment
uses: base44-actions/setup@v2
with:
version: '1.5.0'
- name: Load secrets from secure store
uses: hashicorp/vault-action@v2
with:
url: ${{ secrets.VAULT_URL }}
token: ${{ secrets.VAULT_TOKEN }}
secrets: |
secret/data/base44-app API_KEY | API_KEY
secret/data/base44-app DB_PASSWORD | DB_PASSWORD
- name: Deploy with secure configuration
run: base44 deploy --env production --secure
env:
API_KEY: ${{ env.API_KEY }}
DB_PASSWORD: ${{ env.DB_PASSWORD }}
- name: Post-deployment security verification
run: |
base44 security:verify --env production
base44 security:scan-endpoints --env production
- name: Enable monitoring and alerts
run: base44 monitoring:enable --alerts security
security-documentation:
name: Generate Security Documentation
needs: secure-deployment
runs-on: ubuntu-latest
steps:
- name: Generate deployment security report
run: base44 security:report > deployment-security-report.md
- name: Upload security documentation
uses: actions/upload-artifact@v3
with:
name: security-documentation
path: |
deployment-security-report.md
security-measures.md
Prompt 2: Implementing Infrastructure as Code with Security Best Practices
Use this prompt to generate secure infrastructure as code configurations for your Base44 application:
Create secure infrastructure as code (IaC) configurations for my Base44 application that:
1. Implements the principle of least privilege for all resources
2. Ensures proper network segmentation and security groups
3. Configures secure storage for application data
4. Implements proper encryption for data at rest and in transit
5. Sets up secure logging and monitoring
6. Includes disaster recovery and backup procedures
7. Follows cloud provider security best practices
This prompt will generate code similar to:
# main.tf - Secure Infrastructure for Base44 Application
provider "aws" {
region = var.aws_region
# Enable default encryption for all AWS services that support it
default_tags {
tags = {
Environment = var.environment
Application = "Base44App"
ManagedBy = "Terraform"
}
}
}
# Create a VPC with proper network segmentation
module "vpc" {
source = "terraform-aws-modules/vpc/aws"
version = "3.14.0"
name = "base44-${var.environment}-vpc"
cidr = "10.0.0.0/16"
azs = ["${var.aws_region}a", "${var.aws_region}b", "${var.aws_region}c"]
private_subnets = ["10.0.1.0/24", "10.0.2.0/24", "10.0.3.0/24"]
public_subnets = ["10.0.101.0/24", "10.0.102.0/24", "10.0.103.0/24"]
database_subnets = ["10.0.201.0/24", "10.0.202.0/24", "10.0.203.0/24"]
enable_nat_gateway = true
single_nat_gateway = var.environment != "production"
# Enable VPC flow logs for network monitoring
enable_flow_log = true
flow_log_destination_type = "cloud-watch-logs"
flow_log_destination_arn = aws_cloudwatch_log_group.vpc_flow_logs.arn
# Enable DNS support
enable_dns_hostnames = true
enable_dns_support = true
}
# Security group for application servers with minimal required access
resource "aws_security_group" "app_sg" {
name = "base44-${var.environment}-app-sg"
description = "Security group for Base44 application servers"
vpc_id = module.vpc.vpc_id
# Only allow incoming traffic on application port from load balancer
ingress {
from_port = var.app_port
to_port = var.app_port
protocol = "tcp"
security_groups = [aws_security_group.alb_sg.id]
description = "Allow incoming traffic from ALB only"
}
# Allow outgoing traffic for updates and dependencies
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
description = "Allow outgoing traffic"
}
lifecycle {
create_before_destroy = true
}
}
# Database with encryption and security best practices
module "database" {
source = "terraform-aws-modules/rds/aws"
version = "5.0.0"
identifier = "base44-${var.environment}-db"
engine = "postgres"
engine_version = "14.5"
family = "postgres14"
major_engine_version = "14"
instance_class = var.environment == "production" ? "db.r6g.large" : "db.t4g.medium"
allocated_storage = 20
max_allocated_storage = 100
# Security settings
db_name = "base44app"
username = "base44_dbuser"
port = 5432
# Use secrets manager for password
manage_master_user_password = true
# Enable encryption
storage_encrypted = true
# Multi-AZ for production
multi_az = var.environment == "production"
# Backup and maintenance settings
backup_retention_period = var.environment == "production" ? 30 : 7
backup_window = "03:00-06:00"
maintenance_window = "Mon:00:00-Mon:03:00"
# Enhanced monitoring
monitoring_interval = 60
monitoring_role_name = "base44-${var.environment}-db-monitoring-role"
create_monitoring_role = true
# Network and security
subnet_ids = module.vpc.database_subnets
vpc_security_group_ids = [aws_security_group.db_sg.id]
# Deletion protection for production
deletion_protection = var.environment == "production"
# Parameter group with secure defaults
parameters = [
{
name = "log_connections"
value = "1"
},
{
name = "log_disconnections"
value = "1"
},
{
name = "log_statement"
value = "ddl"
},
{
name = "log_min_duration_statement"
value = "1000"
}
]
}
# Web Application Firewall for API and web protection
resource "aws_wafv2_web_acl" "main" {
name = "base44-${var.environment}-waf"
description = "WAF for Base44 application"
scope = "REGIONAL"
default_action {
allow {}
}
# AWS managed rule sets
rule {
name = "AWS-AWSManagedRulesCommonRuleSet"
priority = 1
override_action {
none {}
}
statement {
managed_rule_group_statement {
name = "AWSManagedRulesCommonRuleSet"
vendor_name = "AWS"
}
}
visibility_config {
cloudwatch_metrics_enabled = true
metric_name = "AWS-AWSManagedRulesCommonRuleSet"
sampled_requests_enabled = true
}
}
# SQL injection protection
rule {
name = "AWS-AWSManagedRulesSQLiRuleSet"
priority = 2
override_action {
none {}
}
statement {
managed_rule_group_statement {
name = "AWSManagedRulesSQLiRuleSet"
vendor_name = "AWS"
}
}
visibility_config {
cloudwatch_metrics_enabled = true
metric_name = "AWS-AWSManagedRulesSQLiRuleSet"
sampled_requests_enabled = true
}
}
# Rate limiting to prevent DDoS
rule {
name = "RateLimitRule"
priority = 3
action {
block {}
}
statement {
rate_based_statement {
limit = 2000
aggregate_key_type = "IP"
}
}
visibility_config {
cloudwatch_metrics_enabled = true
metric_name = "RateLimitRule"
sampled_requests_enabled = true
}
}
visibility_config {
cloudwatch_metrics_enabled = true
metric_name = "base44-${var.environment}-waf"
sampled_requests_enabled = true
}
}
# CloudWatch Logs and Alarms for security monitoring
resource "aws_cloudwatch_log_group" "app_logs" {
name = "/base44/${var.environment}/application"
retention_in_days = var.environment == "production" ? 90 : 30
kms_key_id = aws_kms_key.log_encryption.arn
}
resource "aws_cloudwatch_log_group" "vpc_flow_logs" {
name = "/base44/${var.environment}/vpc-flow-logs"
retention_in_days = var.environment == "production" ? 90 : 30
kms_key_id = aws_kms_key.log_encryption.arn
}
# KMS key for encrypting logs
resource "aws_kms_key" "log_encryption" {
description = "KMS key for encrypting logs"
deletion_window_in_days = 30
enable_key_rotation = true
policy = jsonencode({
Version = "2012-10-17"
Statement = [
{
Effect = "Allow"
Principal = {
Service = "logs.${var.aws_region}.amazonaws.com"
}
Action = [
"kms:Encrypt*",
"kms:Decrypt*",
"kms:ReEncrypt*",
"kms:GenerateDataKey*",
"kms:Describe*"
]
Resource = "*"
}
]
})
}
# Security alarm for suspicious activity
resource "aws_cloudwatch_metric_alarm" "waf_blocked_requests" {
alarm_name = "base44-${var.environment}-waf-blocked-requests"
comparison_operator = "GreaterThanThreshold"
evaluation_periods = 1
metric_name = "BlockedRequests"
namespace = "AWS/WAFV2"
period = 300
statistic = "Sum"
threshold = var.environment == "production" ? 100 : 50
alarm_description = "This alarm monitors for high rates of blocked requests by WAF"
dimensions = {
WebACL = aws_wafv2_web_acl.main.name
Region = var.aws_region
}
alarm_actions = [aws_sns_topic.security_alerts.arn]
ok_actions = [aws_sns_topic.security_alerts.arn]
}
# SNS topic for security alerts
resource "aws_sns_topic" "security_alerts" {
name = "base44-${var.environment}-security-alerts"
kms_master_key_id = aws_kms_key.sns_encryption.id
}
# KMS key for SNS encryption
resource "aws_kms_key" "sns_encryption" {
description = "KMS key for SNS encryption"
deletion_window_in_days = 30
enable_key_rotation = true
}
# S3 bucket for secure application assets with encryption
resource "aws_s3_bucket" "app_assets" {
bucket = "base44-${var.environment}-app-assets-${random_string.bucket_suffix.result}"
# Prevent public access
block_public_acls = true
block_public_policy = true
ignore_public_acls = true
restrict_public_buckets = true
}
resource "aws_s3_bucket_server_side_encryption_configuration" "app_assets" {
bucket = aws_s3_bucket.app_assets.id
rule {
apply_server_side_encryption_by_default {
sse_algorithm = "AES256"
}
}
}
# Random string for unique bucket names
resource "random_string" "bucket_suffix" {
length = 8
special = false
upper = false
}
# Output important information
output "vpc_id" {
value = module.vpc.vpc_id
}
output "database_endpoint" {
value = module.database.db_instance_endpoint
sensitive = true
}
output "assets_bucket_name" {
value = aws_s3_bucket.app_assets.bucket
}
Prompt 3: Creating a Secure Kubernetes Deployment for Base44
Use this prompt to generate secure Kubernetes deployment configurations for your Base44 application:
Create secure Kubernetes deployment configurations for my Base44 application that:
1. Implements pod security policies and network policies
2. Configures resource limits and requests to prevent DoS
3. Sets up proper RBAC for service accounts
4. Implements secure secret management
5. Configures secure ingress with TLS
6. Sets up proper health checks and readiness probes
7. Includes security context configurations for containers
This prompt will generate code similar to:
# base44-app-namespace.yaml
apiVersion: v1
kind: Namespace
metadata:
name: base44-app
labels:
name: base44-app
environment: production
---
# base44-app-network-policy.yaml
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: base44-app-network-policy
namespace: base44-app
spec:
podSelector:
matchLabels:
app: base44-app
policyTypes:
- Ingress
- Egress
ingress:
- from:
- namespaceSelector:
matchLabels:
name: ingress-nginx
ports:
- protocol: TCP
port: 8080
egress:
- to:
- namespaceSelector:
matchLabels:
name: kube-system
ports:
- protocol: TCP
port: 53
- protocol: UDP
port: 53
- to:
- podSelector:
matchLabels:
app: base44-db
ports:
- protocol: TCP
port: 5432
---
# base44-app-rbac.yaml
apiVersion: v1
kind: ServiceAccount
metadata:
name: base44-app-sa
namespace: base44-app
---
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
name: base44-app-role
namespace: base44-app
rules:
- apiGroups: [""]
resources: ["configmaps", "secrets"]
verbs: ["get", "list"]
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "list"]
---
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: base44-app-rolebinding
namespace: base44-app
subjects:
- kind: ServiceAccount
name: base44-app-sa
namespace: base44-app
roleRef:
kind: Role
name: base44-app-role
apiGroup: rbac.authorization.k8s.io
---
# base44-app-deployment.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: base44-app
namespace: base44-app
labels:
app: base44-app
spec:
replicas: 3
selector:
matchLabels:
app: base44-app
strategy:
type: RollingUpdate
rollingUpdate:
maxSurge: 1
maxUnavailable: 0
template:
metadata:
labels:
app: base44-app
annotations:
seccomp.security.alpha.kubernetes.io/pod: runtime/default
spec:
serviceAccountName: base44-app-sa
securityContext:
runAsNonRoot: true
runAsUser: 1000
runAsGroup: 3000
fsGroup: 2000
seccompProfile:
type: RuntimeDefault
containers:
- name: base44-app
image: ${REGISTRY}/base44-app:${VERSION}
imagePullPolicy: Always
securityContext:
allowPrivilegeEscalation: false
readOnlyRootFilesystem: true
capabilities:
drop:
- ALL
ports:
- containerPort: 8080
resources:
limits:
cpu: "1"
memory: "512Mi"
requests:
cpu: "200m"
memory: "256Mi"
livenessProbe:
httpGet:
path: /health
port: 8080
initialDelaySeconds: 30
periodSeconds: 10
timeoutSeconds: 5
failureThreshold: 3
readinessProbe:
httpGet:
path: /ready
port: 8080
initialDelaySeconds: 5
periodSeconds: 5
timeoutSeconds: 3
failureThreshold: 3
env:
- name: NODE_ENV
value: "production"
- name: DB_HOST
valueFrom:
configMapKeyRef:
name: base44-app-config
key: db_host
- name: DB_USER
valueFrom:
secretKeyRef:
name: base44-app-secrets
key: db_user
- name: DB_PASSWORD
valueFrom:
secretKeyRef:
name: base44-app-secrets
key: db_password
volumeMounts:
- name: tmp
mountPath: /tmp
- name: config
mountPath: /app/config
readOnly: true
volumes:
- name: tmp
emptyDir: {}
- name: config
configMap:
name: base44-app-config
imagePullSecrets:
- name: registry-credentials
---
# base44-app-service.yaml
apiVersion: v1
kind: Service
metadata:
name: base44-app
namespace: base44-app
labels:
app: base44-app
spec:
selector:
app: base44-app
ports:
- port: 80
targetPort: 8080
protocol: TCP
type: ClusterIP
---
# base44-app-ingress.yaml
apiVersion: networking.k8s.io/v1
kind: Ingress
metadata:
name: base44-app-ingress
namespace: base44-app
annotations:
kubernetes.io/ingress.class: "nginx"
nginx.ingress.kubernetes.io/ssl-redirect: "true"
nginx.ingress.kubernetes.io/force-ssl-redirect: "true"
nginx.ingress.kubernetes.io/proxy-body-size: "10m"
nginx.ingress.kubernetes.io/proxy-buffer-size: "128k"
cert-manager.io/cluster-issuer: "letsencrypt-prod"
nginx.ingress.kubernetes.io/configuration-snippet: |
more_set_headers "X-Frame-Options: DENY";
more_set_headers "X-Content-Type-Options: nosniff";
more_set_headers "X-XSS-Protection: 1; mode=block";
more_set_headers "Referrer-Policy: strict-origin-when-cross-origin";
more_set_headers "Content-Security-Policy: default-src 'self'; script-src 'self'; img-src 'self'; style-src 'self'; font-src 'self'; connect-src 'self';";
spec:
tls:
- hosts:
- app.base44-example.com
secretName: base44-app-tls
rules:
- host: app.base44-example.com
http:
paths:
- path: /
pathType: Prefix
backend:
service:
name: base44-app
port:
number: 80
---
# base44-app-secrets.yaml (Apply this with sealed-secrets or external secrets operator)
apiVersion: v1
kind: Secret
metadata:
name: base44-app-secrets
namespace: base44-app
type: Opaque
data:
db_user: YmFzZTQ0X3VzZXI= # base44_user
db_password: <base64-encoded-password> # Replace with actual password
api_key: <base64-encoded-api-key> # Replace with actual API key
---
# base44-app-config.yaml
apiVersion: v1
kind: ConfigMap
metadata:
name: base44-app-config
namespace: base44-app
data:
db_host: "base44-db.base44-app.svc.cluster.local"
app_config.json: |
{
"logLevel": "info",
"rateLimiting": {
"enabled": true,
"maxRequestsPerMinute": 100
},
"security": {
"corsAllowedOrigins": ["https://app.base44-example.com"],
"enableCSRFProtection": true,
"sessionTimeout": 3600
}
}
---
# base44-app-hpa.yaml
apiVersion: autoscaling/v2
kind: HorizontalPodAutoscaler
metadata:
name: base44-app-hpa
namespace: base44-app
spec:
scaleTargetRef:
apiVersion: apps/v1
kind: Deployment
name: base44-app
minReplicas: 2
maxReplicas: 10
metrics:
- type: Resource
resource:
name: cpu
target:
type: Utilization
averageUtilization: 70
- type: Resource
resource:
name: memory
target:
type: Utilization
averageUtilization: 80
Best Practices for Secure Deployment in Base44
When deploying Base44 applications to production, follow these best practices to ensure security:
1. Implement a Secure CI/CD Pipeline
A secure CI/CD pipeline is the foundation of secure deployment. Your pipeline should:
- Scan code for security vulnerabilities at every stage
- Validate infrastructure as code configurations
- Ensure secrets are properly managed
- Implement proper access controls for deployment processes
- Include post-deployment security verification
2. Use Infrastructure as Code (IaC) with Security Guardrails
Infrastructure as Code allows you to define your infrastructure in a declarative way, making it easier to implement security best practices:
- Define all infrastructure components as code
- Implement the principle of least privilege
- Ensure proper network segmentation
- Configure encryption for data at rest and in transit
- Set up proper logging and monitoring
- Include disaster recovery procedures
3. Implement Container Security Best Practices
If you’re deploying Base44 applications in containers:
- Use minimal base images
- Scan container images for vulnerabilities
- Implement proper resource limits
- Run containers as non-root users
- Use read-only file systems where possible
- Implement proper network policies
4. Secure Your Secrets Management
Proper secrets management is critical for secure deployments:
- Never hardcode secrets in your code or configuration files
- Use a dedicated secrets management solution (HashiCorp Vault, AWS Secrets Manager, etc.)
- Rotate secrets regularly
- Implement the principle of least privilege for secret access
- Audit secret access
5. Implement Proper Monitoring and Logging
Visibility into your application’s behavior is essential for security:
- Implement comprehensive logging
- Set up alerts for suspicious activities
- Monitor for unusual traffic patterns
- Implement proper log retention policies
- Ensure logs are securely stored and cannot be tampered with
6. Implement Defense in Depth
Don’t rely on a single security control:
- Implement multiple layers of security
- Use Web Application Firewalls (WAF)
- Implement rate limiting
- Use proper authentication and authorization
- Implement network segmentation
7. Automate Security Testing
Integrate security testing into your deployment process:
- Implement automated vulnerability scanning
- Perform regular penetration testing
- Use static and dynamic application security testing
- Implement continuous security validation
Testing Your Secure Deployment
After implementing secure deployment practices, test your deployment to ensure it meets security requirements:
-
Vulnerability Scanning: Use tools like OWASP ZAP, Nessus, or Qualys to scan your deployed application for vulnerabilities.
-
Penetration Testing: Conduct regular penetration testing to identify security weaknesses.
-
Configuration Validation: Validate your infrastructure configurations against security benchmarks (CIS, NIST, etc.).
-
Secret Scanning: Verify that no secrets are exposed in your deployment.
-
Access Control Testing: Verify that proper access controls are in place.
-
Monitoring and Logging Validation: Ensure that monitoring and logging are properly configured and working.
Conclusion
Secure deployment practices are essential for Base44 applications, especially given the rapid development nature of vibe coding. By implementing the practices outlined in this blog post and using the provided prompts, you can ensure that your Base44 applications are deployed securely, protecting your data and users from potential threats.
Remember that security is an ongoing process, not a one-time activity. Regularly review and update your deployment practices to address new threats and vulnerabilities as they emerge. By prioritizing security in your Base44 deployments, you can enjoy the benefits of rapid development without compromising on security.