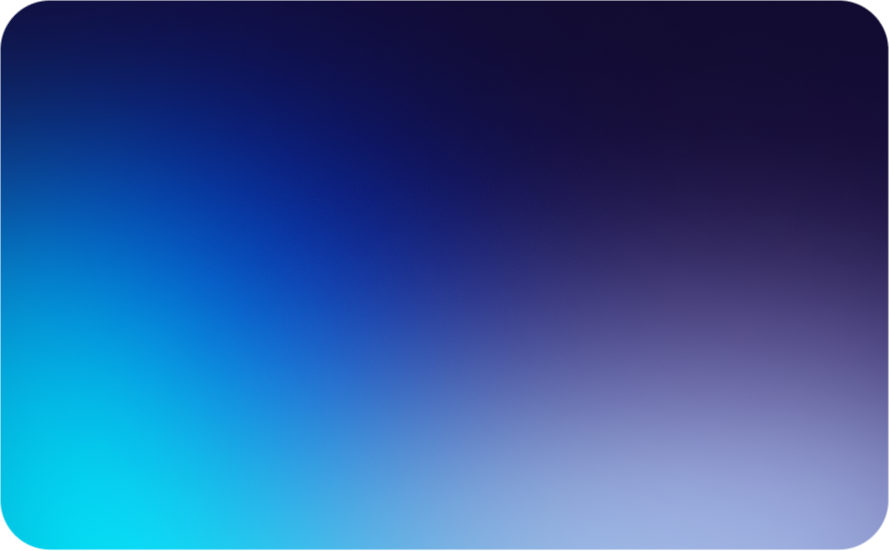
Secure Third-Party Integrations with Base44: A Comprehensive Guide
In the rapidly evolving landscape of vibe coding, Base44 has emerged as a powerful tool for developers looking to build applications quickly and efficiently. However, as with any AI-assisted development platform, security concerns arise—particularly when integrating with third-party services. These integrations, while essential for modern applications, can introduce significant vulnerabilities if not implemented securely.
When developers instruct Base44 to “add payment processing” or “integrate with the OpenAI API,” the resulting code often prioritizes functionality over security. This approach can lead to exposed API keys, insecure data transmission, and vulnerable authentication mechanisms. In this blog post, we’ll explore common security issues in Base44 third-party integrations and provide specific prompts you can use to generate more secure code.
Common Security Vulnerabilities in Base44 Third-Party Integrations
1. Hardcoded API Keys and Credentials
One of the most prevalent and dangerous practices in Base44-generated code is hardcoding API keys directly in application files:
// Vulnerable Base44-generated code
const stripe = require('stripe')('sk_live_51HG8h4JKleHkPwaxc7e9JKleHkPwaxc7e9');
app.post('/create-payment', async (req, res) => {
try {
const paymentIntent = await stripe.paymentIntents.create({
amount: req.body.amount,
currency: 'usd',
});
res.status(200).json({ clientSecret: paymentIntent.client_secret });
} catch (error) {
res.status(500).json({ error: error.message });
}
});
This approach is problematic because:
- API keys are exposed in source code repositories
- Keys cannot be rotated without code changes
- Different environments (development, staging, production) share the same keys
- Compromised keys give attackers direct access to services and data
2. Insufficient Validation of Third-Party Data
Base44 often generates code that trusts third-party responses without proper validation:
// Vulnerable Base44-generated code
app.get('/weather', async (req, res) => {
try {
const location = req.query.location;
const response = await axios.get(`https://weather-api.example.com/data?location=${location}`);
// No validation of the response data
res.status(200).json(response.data);
} catch (error) {
res.status(500).json({ error: 'Failed to fetch weather data' });
}
});
This approach is risky because:
- Malformed or malicious responses can propagate through your application
- Data type inconsistencies can cause application errors
- Missing validation enables potential injection attacks
- Sensitive information might be unintentionally exposed
3. Inadequate Error Handling and Logging
Base44 typically generates basic error handling that can either reveal too much information or fail to capture important details:
// Vulnerable Base44-generated code
app.post('/process-webhook', async (req, res) => {
try {
const event = req.body;
// Process the webhook event
await handleWebhookEvent(event);
res.status(200).send('Webhook processed');
} catch (error) {
console.error('Webhook error:', error);
res.status(500).json({ error: error.toString() });
}
});
This approach is problematic because:
- Detailed error messages can expose implementation details to attackers
- Insufficient logging makes security incidents harder to detect and investigate
- Generic error responses don’t provide actionable information for legitimate users
- No distinction between different types of errors (validation, authentication, server)
Prompts for Implementing Secure Third-Party Integrations in Base44
Now that we’ve identified common vulnerabilities, let’s look at specific prompts you can give Base44 to generate more secure code for third-party integrations.
Prompt 1: Implementing Secure API Key Management
Create a Node.js Express application that securely integrates with the Stripe API using environment variables for credentials. Implement:
1. A secure configuration module that loads API keys from environment variables
2. Proper error handling that doesn't expose sensitive information
3. Separate configurations for development and production environments
4. A validation mechanism to ensure required API keys are present before the application starts
Use best practices for API key management and ensure no credentials are hardcoded in the source code.
This prompt will generate code similar to:
// config.js - Secure configuration module
require('dotenv').config();
const config = {
stripe: {
secretKey: process.env.STRIPE_SECRET_KEY,
webhookSecret: process.env.STRIPE_WEBHOOK_SECRET,
publishableKey: process.env.STRIPE_PUBLISHABLE_KEY,
},
environment: process.env.NODE_ENV || 'development',
isProduction: (process.env.NODE_ENV || 'development') === 'production',
};
// Validate required environment variables
const requiredEnvVars = [
'STRIPE_SECRET_KEY',
'STRIPE_WEBHOOK_SECRET',
];
const missingEnvVars = requiredEnvVars.filter(envVar => !process.env[envVar]);
if (missingEnvVars.length > 0) {
throw new Error(`Missing required environment variables: ${missingEnvVars.join(', ')}`);
}
module.exports = config;
// app.js - Main application file
const express = require('express');
const config = require('./config');
const stripe = require('stripe')(config.stripe.secretKey);
const app = express();
app.use(express.json());
app.post('/create-payment', async (req, res) => {
try {
// Validate request data
if (!req.body.amount || typeof req.body.amount !== 'number') {
return res.status(400).json({ error: 'Valid amount is required' });
}
const paymentIntent = await stripe.paymentIntents.create({
amount: req.body.amount,
currency: 'usd',
});
res.status(200).json({ clientSecret: paymentIntent.client_secret });
} catch (error) {
// Log the detailed error internally
console.error('Payment creation error:', error);
// Return a sanitized error to the client
res.status(500).json({
error: 'Unable to process payment request',
requestId: req.id // Assuming you have request ID middleware
});
}
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running in ${config.environment} mode on port ${PORT}`);
});
Prompt 2: Implementing Secure Webhook Processing
Create a Node.js application that securely handles webhooks from a third-party service (like GitHub, Stripe, or SendGrid). Implement:
1. Signature verification to validate webhook authenticity
2. Request body parsing that preserves the raw body for signature verification
3. Idempotency handling to prevent duplicate processing
4. Comprehensive logging for security auditing
5. Proper error handling with appropriate status codes
Ensure the implementation follows security best practices and is resistant to replay attacks.
This prompt will generate code similar to:
const express = require('express');
const crypto = require('crypto');
const config = require('./config');
const app = express();
// Custom middleware to preserve raw body for signature verification
app.use(express.json({
verify: (req, res, buf) => {
req.rawBody = buf;
}
}));
// Webhook processing endpoint
app.post('/webhooks/stripe', async (req, res) => {
try {
// 1. Verify webhook signature
const signature = req.headers['stripe-signature'];
if (!signature) {
console.warn('Missing Stripe signature', {
ip: req.ip,
path: req.path,
timestamp: new Date().toISOString()
});
return res.status(400).json({ error: 'Missing signature header' });
}
let event;
try {
const webhookSecret = config.stripe.webhookSecret;
const payload = req.rawBody;
// Verify the event using the webhook secret and signature
const signatureTimestamp = signature.split(',')[0].split('=')[1];
const signatureValue = signature.split(',')[1].split('=')[1];
// Recreate the expected signature
const expectedSignature = crypto
.createHmac('sha256', webhookSecret)
.update(`${signatureTimestamp}.${payload.toString()}`)
.digest('hex');
// Compare signatures using constant-time comparison
const isSignatureValid = crypto.timingSafeEqual(
Buffer.from(expectedSignature),
Buffer.from(signatureValue)
);
if (!isSignatureValid) {
throw new Error('Invalid signature');
}
// Parse the event
event = JSON.parse(payload);
} catch (err) {
console.error('Webhook signature verification failed', {
error: err.message,
ip: req.ip,
path: req.path,
timestamp: new Date().toISOString()
});
return res.status(400).json({ error: 'Invalid webhook signature' });
}
// 2. Check for duplicate events (idempotency)
const eventId = event.id;
const isProcessed = await checkIfEventProcessed(eventId);
if (isProcessed) {
// Already processed this event, return success to prevent retries
console.info('Duplicate webhook event received', { eventId });
return res.status(200).json({ status: 'Already processed' });
}
// 3. Process the webhook event based on type
switch (event.type) {
case 'payment_intent.succeeded':
await handlePaymentSuccess(event.data.object);
break;
case 'payment_intent.payment_failed':
await handlePaymentFailure(event.data.object);
break;
// Handle other event types
default:
console.info('Unhandled webhook event type', { type: event.type, eventId });
}
// 4. Mark event as processed
await markEventAsProcessed(eventId);
// 5. Return success
res.status(200).json({ received: true });
} catch (error) {
// Log the error with context but without sensitive details
console.error('Webhook processing error', {
error: error.message,
stack: config.isProduction ? undefined : error.stack,
path: req.path,
timestamp: new Date().toISOString()
});
// Return appropriate status code based on the error
if (error.type === 'StripeSignatureVerificationError') {
return res.status(400).json({ error: 'Webhook signature verification failed' });
}
res.status(500).json({ error: 'Webhook processing failed' });
}
});
// Helper functions
async function checkIfEventProcessed(eventId) {
// Implementation to check if event has been processed
// This would typically use a database
return false; // Placeholder
}
async function markEventAsProcessed(eventId) {
// Implementation to mark event as processed
// This would typically use a database
}
async function handlePaymentSuccess(paymentIntent) {
// Implementation to handle successful payment
console.info('Payment succeeded', { paymentIntentId: paymentIntent.id });
}
async function handlePaymentFailure(paymentIntent) {
// Implementation to handle failed payment
console.warn('Payment failed', {
paymentIntentId: paymentIntent.id,
failureCode: paymentIntent.last_payment_error?.code,
failureMessage: paymentIntent.last_payment_error?.message
});
}
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Webhook server running on port ${PORT}`);
});
Prompt 3: Implementing Secure OAuth Integration
Create a Node.js Express application that implements a secure OAuth 2.0 flow for integrating with a third-party service (like GitHub, Google, or Microsoft). Implement:
1. Secure storage of client ID and client secret using environment variables
2. PKCE (Proof Key for Code Exchange) for enhanced security
3. State parameter validation to prevent CSRF attacks
4. Secure token storage and refresh mechanisms
5. Proper scope handling and validation
Ensure the implementation follows OAuth 2.0 security best practices and handles errors appropriately.
This prompt will generate code similar to:
const express = require('express');
const axios = require('axios');
const crypto = require('crypto');
const session = require('express-session');
const { v4: uuidv4 } = require('uuid');
require('dotenv').config();
const app = express();
// Session configuration for storing OAuth state and PKCE values
app.use(session({
secret: process.env.SESSION_SECRET || crypto.randomBytes(32).toString('hex'),
resave: false,
saveUninitialized: false,
cookie: {
secure: process.env.NODE_ENV === 'production',
httpOnly: true,
maxAge: 15 * 60 * 1000 // 15 minutes
}
}));
// OAuth configuration
const oauthConfig = {
clientId: process.env.GITHUB_CLIENT_ID,
clientSecret: process.env.GITHUB_CLIENT_SECRET,
redirectUri: process.env.REDIRECT_URI || 'http://localhost:3000/oauth/callback',
authorizationEndpoint: 'https://github.com/login/oauth/authorize',
tokenEndpoint: 'https://github.com/login/oauth/access_token',
scope: 'user:email repo',
// Add other necessary endpoints
};
// Validate required environment variables
if (!oauthConfig.clientId || !oauthConfig.clientSecret) {
console.error('Missing required environment variables: GITHUB_CLIENT_ID and/or GITHUB_CLIENT_SECRET');
process.exit(1);
}
// Generate a random string for PKCE and state
function generateRandomString(length = 48) {
return crypto.randomBytes(length).toString('hex');
}
// Create a code challenge from the code verifier (for PKCE)
function generateCodeChallenge(codeVerifier) {
const base64URLEncode = str =>
str.toString('base64')
.replace(/\+/g, '-')
.replace(/\//g, '_')
.replace(/=/g, '');
const sha256 = str =>
crypto.createHash('sha256').update(str).digest();
return base64URLEncode(sha256(codeVerifier));
}
// Initiate OAuth flow
app.get('/auth/github', (req, res) => {
// Generate and store PKCE code verifier
const codeVerifier = generateRandomString();
const codeChallenge = generateCodeChallenge(codeVerifier);
// Generate and store state parameter to prevent CSRF
const state = uuidv4();
// Store in session
req.session.oauthState = state;
req.session.codeVerifier = codeVerifier;
// Build authorization URL
const authorizationUrl = new URL(oauthConfig.authorizationEndpoint);
authorizationUrl.searchParams.append('client_id', oauthConfig.clientId);
authorizationUrl.searchParams.append('redirect_uri', oauthConfig.redirectUri);
authorizationUrl.searchParams.append('state', state);
authorizationUrl.searchParams.append('scope', oauthConfig.scope);
authorizationUrl.searchParams.append('response_type', 'code');
authorizationUrl.searchParams.append('code_challenge', codeChallenge);
authorizationUrl.searchParams.append('code_challenge_method', 'S256');
// Redirect user to authorization URL
res.redirect(authorizationUrl.toString());
});
// Handle OAuth callback
app.get('/oauth/callback', async (req, res) => {
try {
const { code, state } = req.query;
// Validate state parameter to prevent CSRF attacks
if (!state || state !== req.session.oauthState) {
console.warn('OAuth state mismatch', {
expectedState: req.session.oauthState,
receivedState: state,
ip: req.ip,
timestamp: new Date().toISOString()
});
return res.status(400).send('Invalid state parameter. Possible CSRF attack.');
}
// Validate authorization code is present
if (!code) {
return res.status(400).send('Missing authorization code');
}
// Exchange authorization code for tokens
const tokenResponse = await axios.post(
oauthConfig.tokenEndpoint,
{
client_id: oauthConfig.clientId,
client_secret: oauthConfig.clientSecret,
code,
redirect_uri: oauthConfig.redirectUri,
code_verifier: req.session.codeVerifier,
grant_type: 'authorization_code'
},
{
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
}
}
);
// Extract tokens
const { access_token, refresh_token, expires_in } = tokenResponse.data;
// Store tokens securely (in a real app, you'd use a database)
// Never store tokens in client-side JavaScript or cookies without proper protection
req.session.tokens = {
accessToken: access_token,
refreshToken: refresh_token,
expiresAt: Date.now() + (expires_in * 1000)
};
// Clear PKCE and state values as they're no longer needed
delete req.session.oauthState;
delete req.session.codeVerifier;
// Redirect to protected area or show success message
res.redirect('/dashboard');
} catch (error) {
console.error('OAuth callback error', {
error: error.message,
stack: process.env.NODE_ENV === 'production' ? undefined : error.stack,
timestamp: new Date().toISOString()
});
res.status(500).send('Authentication failed. Please try again.');
}
});
// Protected route example
app.get('/dashboard', async (req, res) => {
// Check if user is authenticated
if (!req.session.tokens || !req.session.tokens.accessToken) {
return res.redirect('/auth/github');
}
// Check if token is expired and refresh if necessary
if (req.session.tokens.expiresAt < Date.now() && req.session.tokens.refreshToken) {
try {
await refreshAccessToken(req);
} catch (error) {
// If refresh fails, redirect to login
return res.redirect('/auth/github');
}
}
// Use the access token to fetch user data
try {
const userResponse = await axios.get('https://api.github.com/user', {
headers: {
'Authorization': `Bearer ${req.session.tokens.accessToken}`,
'Accept': 'application/json'
}
});
res.send(`
<h1>Dashboard</h1>
<p>Welcome, ${userResponse.data.name || userResponse.data.login}!</p>
<img src="${userResponse.data.avatar_url}" width="100" alt="Profile picture">
<p>GitHub profile: <a href="${userResponse.data.html_url}" target="_blank">${userResponse.data.html_url}</a></p>
<a href="/logout">Logout</a>
`);
} catch (error) {
console.error('API request error', {
error: error.message,
timestamp: new Date().toISOString()
});
res.status(500).send('Error fetching user data');
}
});
// Helper function to refresh access token
async function refreshAccessToken(req) {
const tokenResponse = await axios.post(
oauthConfig.tokenEndpoint,
{
client_id: oauthConfig.clientId,
client_secret: oauthConfig.clientSecret,
refresh_token: req.session.tokens.refreshToken,
grant_type: 'refresh_token'
},
{
headers: {
'Accept': 'application/json',
'Content-Type': 'application/json'
}
}
);
const { access_token, refresh_token, expires_in } = tokenResponse.data;
// Update tokens in session
req.session.tokens = {
accessToken: access_token,
refreshToken: refresh_token || req.session.tokens.refreshToken,
expiresAt: Date.now() + (expires_in * 1000)
};
}
// Logout route
app.get('/logout', (req, res) => {
req.session.destroy();
res.redirect('/');
});
// Home page
app.get('/', (req, res) => {
res.send(`
<h1>OAuth 2.0 Demo</h1>
<a href="/auth/github">Login with GitHub</a>
`);
});
// Start the server
const PORT = process.env.PORT || 3000;
app.listen(PORT, () => {
console.log(`Server running on port ${PORT}`);
});
Best Practices for Secure Third-Party Integrations in Base44
When using Base44 to generate code for third-party integrations, keep these best practices in mind:
1. Secure Credential Management
- Use Environment Variables: Always store API keys, secrets, and credentials in environment variables, never in code.
- Implement Secret Rotation: Design your application to make credential rotation easy without code changes.
- Use Secret Management Services: For production applications, consider using dedicated secret management services like AWS Secrets Manager, HashiCorp Vault, or cloud-native solutions.
- Validate Configuration: Check that all required credentials are available before your application starts.
2. Secure Communication
- Always Use HTTPS: Ensure all API calls to third-party services use HTTPS.
- Validate Certificates: Implement proper certificate validation and avoid disabling certificate checks.
- Implement Request Signing: When available, use request signing mechanisms provided by the third-party service.
- Use Secure Webhook Endpoints: Validate webhook signatures and implement proper authentication for incoming webhooks.
3. Data Validation and Sanitization
- Validate All Inputs: Validate and sanitize all data received from third-party services before processing.
- Implement Schema Validation: Use JSON Schema or similar tools to validate the structure of API responses.
- Handle Data Type Inconsistencies: Be prepared for changes in API responses and handle them gracefully.
- Limit Data Exposure: Only request and store the minimum data needed from third-party services.
4. Error Handling and Logging
- Implement Proper Error Handling: Catch and handle all exceptions from third-party API calls.
- Use Structured Logging: Log errors with context but without sensitive information.
- Implement Retry Mechanisms: Use exponential backoff for retrying failed API calls.
- Monitor Integration Health: Set up monitoring and alerting for third-party integration failures.
5. Rate Limiting and Throttling
- Respect API Limits: Implement rate limiting to respect the third-party service’s constraints.
- Handle Rate Limit Errors: Properly handle 429 Too Many Requests responses.
- Implement Queuing: For high-volume operations, use a queue to manage API calls.
- Monitor Usage: Track API usage to avoid unexpected charges and service disruptions.
Conclusion
Secure third-party integrations are critical for maintaining the overall security posture of your Base44-generated applications. By using the prompts provided in this blog post, you can guide Base44 to generate more secure code that follows industry best practices for credential management, secure communication, data validation, and error handling.
Remember that while Base44 can help you implement secure integrations, it’s still your responsibility to understand the security implications of the third-party services you’re using and to regularly audit and update your integrations as security requirements evolve.
By taking a security-first approach to third-party integrations in your Base44 applications, you can enjoy the productivity benefits of vibe coding while maintaining a strong security posture that protects your users’ data and your organization’s reputation.