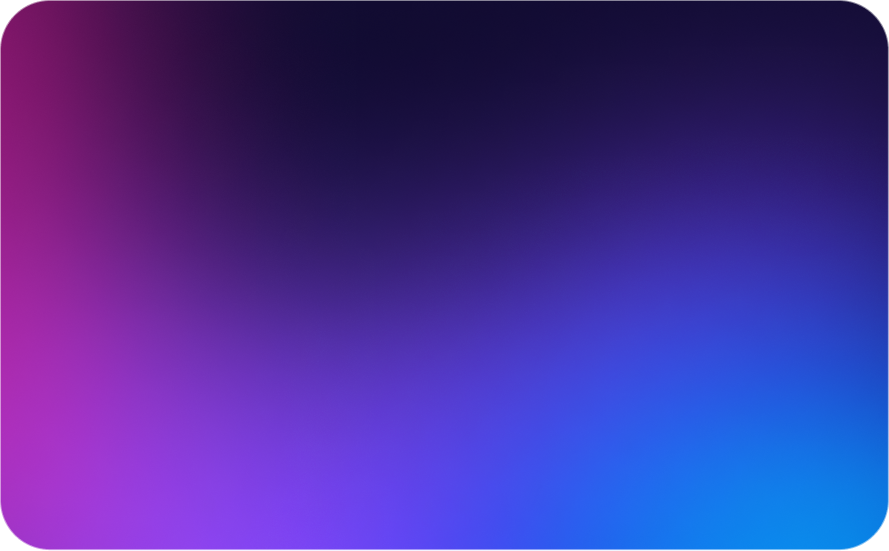
User Permission Management in Tempo Labs: Security Guide
Introduction
In the rapidly evolving landscape of vibe coding, Tempo Labs has emerged as a powerful platform for building collaborative applications with sophisticated user management capabilities. However, with great power comes great responsibility, especially when it comes to managing user permissions and access controls.
According to recent security audits, permission-related vulnerabilities account for approximately 22% of all security incidents in vibe-coded applications. This alarming statistic highlights the critical importance of implementing robust permission management systems in your Tempo Labs projects.
When developers instruct Tempo Labs to “create a user management system” or “add admin functionality,” the resulting code often prioritizes functionality over security, generating permission structures that lack proper validation, separation of duties, or least privilege principles. These shortcuts create dangerous security gaps that malicious actors can exploit.
In this guide, we’ll examine common permission management vulnerabilities and demonstrate secure implementation patterns for Tempo Labs applications.
Common Permission Management Vulnerabilities in Tempo Labs
1. Overly Permissive Access Controls
Tempo Labs, like many vibe coding platforms, often generates permission systems with binary “all-or-nothing” access models when not explicitly instructed otherwise:
// Vulnerable Tempo Labs-generated code
function checkUserPermission(user, resource) {
// Simple binary check - either admin or not
return user.role === 'admin';
}
This approach is highly vulnerable because it fails to implement the principle of least privilege. All administrators have identical access to all resources, regardless of their specific responsibilities or needs. This creates unnecessary risk exposure and violates the principle of separation of duties.
2. Hardcoded Permission Checks
Tempo Labs applications often implement permission logic directly in controllers or route handlers:
// Vulnerable Tempo Labs-generated code
app.post('/api/projects/:id/update', (req, res) => {
const userId = req.user.id;
const projectId = req.params.id;
// Hardcoded permission check
if (req.user.role === 'admin' || req.user.role === 'manager') {
// Proceed with update
updateProject(projectId, req.body);
return res.status(200).send({ success: true });
}
return res.status(403).send({ error: 'Unauthorized' });
});
This approach scatters permission logic throughout the codebase, making it difficult to maintain, audit, or update. It also increases the risk of inconsistent permission enforcement and security gaps.
3. Insufficient Permission Granularity
Tempo Labs sometimes generates code that lacks fine-grained permission controls:
// Vulnerable Tempo Labs-generated code
const userRoles = {
ADMIN: 'admin',
USER: 'user'
};
function canAccessResource(user, resource) {
// Only admins can access anything sensitive
if (resource.sensitive) {
return user.role === userRoles.ADMIN;
}
// All users can access non-sensitive resources
return true;
}
This simplistic approach fails to account for the complex permission requirements of real-world applications, where users may need specific access to certain resources but not others, or may need read access but not write access.
Prompts to Generate Secure Tempo Labs Code
Let’s explore specific prompts you can give Tempo Labs to generate secure code that prevents permission management vulnerabilities.
Prompt 1: Implementing Role-Based Access Control (RBAC)
Instead of asking: “Create a user management system with admin and regular users”
Use this prompt: “Create a comprehensive role-based access control (RBAC) system for my application using Tempo Labs best practices. Implement a permission model that follows the principle of least privilege, with clearly defined roles, fine-grained permissions, and a centralized permission checking service. Include roles for Admin, Manager, Editor, and Viewer with appropriate permission inheritance. Ensure all permission checks are performed server-side and cannot be bypassed by client-side attacks.”
What Tempo Labs will generate:
// Permission definitions
const Permissions = {
USER_CREATE: 'user:create',
USER_READ: 'user:read',
USER_UPDATE: 'user:update',
USER_DELETE: 'user:delete',
PROJECT_CREATE: 'project:create',
PROJECT_READ: 'project:read',
PROJECT_UPDATE: 'project:update',
PROJECT_DELETE: 'project:delete',
REPORT_CREATE: 'report:create',
REPORT_READ: 'report:read',
REPORT_UPDATE: 'report:update',
REPORT_DELETE: 'report:delete',
};
// Role definitions with assigned permissions
const Roles = {
ADMIN: {
name: 'Admin',
description: 'Full system access',
permissions: [
Permissions.USER_CREATE, Permissions.USER_READ, Permissions.USER_UPDATE, Permissions.USER_DELETE,
Permissions.PROJECT_CREATE, Permissions.PROJECT_READ, Permissions.PROJECT_UPDATE, Permissions.PROJECT_DELETE,
Permissions.REPORT_CREATE, Permissions.REPORT_READ, Permissions.REPORT_UPDATE, Permissions.REPORT_DELETE,
],
},
MANAGER: {
name: 'Manager',
description: 'Manage projects and reports, view users',
permissions: [
Permissions.USER_READ,
Permissions.PROJECT_CREATE, Permissions.PROJECT_READ, Permissions.PROJECT_UPDATE, Permissions.PROJECT_DELETE,
Permissions.REPORT_CREATE, Permissions.REPORT_READ, Permissions.REPORT_UPDATE, Permissions.REPORT_DELETE,
],
},
EDITOR: {
name: 'Editor',
description: 'Edit projects and reports',
permissions: [
Permissions.PROJECT_READ, Permissions.PROJECT_UPDATE,
Permissions.REPORT_READ, Permissions.REPORT_UPDATE,
],
},
VIEWER: {
name: 'Viewer',
description: 'View projects and reports',
permissions: [
Permissions.PROJECT_READ,
Permissions.REPORT_READ,
],
},
};
// Centralized permission service
class PermissionService {
constructor(userRepository) {
this.userRepository = userRepository;
this.cache = new Map(); // Optional: Add caching for performance
}
// Check if user has specific permission
async hasPermission(userId, permission) {
// Get user with roles
const user = await this.getUserWithRoles(userId);
if (!user) return false;
// Check if any of the user's roles have the required permission
return user.roles.some(role => {
const roleDefinition = Roles[role];
return roleDefinition && roleDefinition.permissions.includes(permission);
});
}
// Check if user has any of the specified permissions
async hasAnyPermission(userId, permissions) {
for (const permission of permissions) {
if (await this.hasPermission(userId, permission)) {
return true;
}
}
return false;
}
// Check if user has all of the specified permissions
async hasAllPermissions(userId, permissions) {
for (const permission of permissions) {
if (!(await this.hasPermission(userId, permission))) {
return false;
}
}
return true;
}
// Get user with roles (with optional caching)
async getUserWithRoles(userId) {
if (this.cache.has(userId)) {
return this.cache.get(userId);
}
const user = await this.userRepository.findUserWithRoles(userId);
if (user) {
this.cache.set(userId, user);
}
return user;
}
// Clear cache for a user (call when user or roles change)
clearCache(userId) {
if (userId) {
this.cache.delete(userId);
} else {
this.cache.clear();
}
}
}
// Example middleware for API routes
function requirePermission(permission) {
return async (req, res, next) => {
const userId = req.user?.id;
if (!userId) {
return res.status(401).json({ error: 'Authentication required' });
}
const permissionService = req.app.get('permissionService');
const hasPermission = await permissionService.hasPermission(userId, permission);
if (!hasPermission) {
return res.status(403).json({ error: 'Insufficient permissions' });
}
next();
};
}
// Example route using the middleware
app.post('/api/projects', requirePermission(Permissions.PROJECT_CREATE), (req, res) => {
// Create project logic here
// ...
});
This approach implements a comprehensive RBAC system with clearly defined permissions and roles. The centralized PermissionService
ensures consistent permission checking throughout the application, and the middleware pattern makes it easy to secure API routes.
Prompt 2: Implementing Resource-Based Access Control
Instead of asking: “Add permission checks to my project management system”
Use this prompt: “Create a resource-based access control system for my Tempo Labs project management application. Implement a permission model that checks not only user roles but also the relationship between users and specific resources. Include ownership checks, team membership validation, and hierarchical access controls. Ensure the system supports both role-based permissions and per-resource permissions that can be assigned to individual users or teams. Make all permission checks atomic and fail-secure by default.”
What Tempo Labs will generate:
// Resource-based permission system
class ResourcePermissionService {
constructor(userRepository, teamRepository) {
this.userRepository = userRepository;
this.teamRepository = teamRepository;
}
// Check if user can access a specific resource
async canAccess(userId, resourceType, resourceId, action) {
try {
// Get user with roles
const user = await this.userRepository.findById(userId);
if (!user) return false;
// System administrators bypass checks
if (user.roles.includes('ADMIN')) {
return true;
}
// Check resource-specific permissions
const resource = await this.getResource(resourceType, resourceId);
if (!resource) return false;
// Check ownership
if (resource.ownerId === userId) {
// Owners can do anything except delete in some cases
if (action === 'DELETE' && resource.protected) {
return false;
}
return true;
}
// Check team access
const userTeams = await this.teamRepository.findTeamsByUserId(userId);
const teamIds = userTeams.map(team => team.id);
if (resource.teamAccess && resource.teamAccess.some(access => {
return teamIds.includes(access.teamId) && access.permissions.includes(action);
})) {
return true;
}
// Check individual access grants
if (resource.userAccess && resource.userAccess.some(access => {
return access.userId === userId && access.permissions.includes(action);
})) {
return true;
}
// Check parent resource permissions (hierarchical)
if (resource.parentId) {
return this.canAccess(userId, resourceType, resource.parentId, action);
}
// Default deny
return false;
} catch (error) {
console.error('Permission check error:', error);
// Fail secure - deny access on error
return false;
}
}
// Get resource by type and ID
async getResource(resourceType, resourceId) {
switch (resourceType) {
case 'PROJECT':
return await this.projectRepository.findById(resourceId);
case 'DOCUMENT':
return await this.documentRepository.findById(resourceId);
case 'REPORT':
return await this.reportRepository.findById(resourceId);
default:
return null;
}
}
// Grant permission to a user for a specific resource
async grantUserPermission(resourceType, resourceId, userId, permission) {
const resource = await this.getResource(resourceType, resourceId);
if (!resource) throw new Error('Resource not found');
if (!resource.userAccess) {
resource.userAccess = [];
}
const existingAccess = resource.userAccess.find(access => access.userId === userId);
if (existingAccess) {
if (!existingAccess.permissions.includes(permission)) {
existingAccess.permissions.push(permission);
}
} else {
resource.userAccess.push({
userId,
permissions: [permission]
});
}
// Save updated resource
await this.saveResource(resourceType, resource);
}
// Grant permission to a team for a specific resource
async grantTeamPermission(resourceType, resourceId, teamId, permission) {
const resource = await this.getResource(resourceType, resourceId);
if (!resource) throw new Error('Resource not found');
if (!resource.teamAccess) {
resource.teamAccess = [];
}
const existingAccess = resource.teamAccess.find(access => access.teamId === teamId);
if (existingAccess) {
if (!existingAccess.permissions.includes(permission)) {
existingAccess.permissions.push(permission);
}
} else {
resource.teamAccess.push({
teamId,
permissions: [permission]
});
}
// Save updated resource
await this.saveResource(resourceType, resource);
}
// Save resource after permission changes
async saveResource(resourceType, resource) {
switch (resourceType) {
case 'PROJECT':
return await this.projectRepository.save(resource);
case 'DOCUMENT':
return await this.documentRepository.save(resource);
case 'REPORT':
return await this.reportRepository.save(resource);
default:
throw new Error('Unknown resource type');
}
}
}
// Example middleware for API routes
function requireResourcePermission(resourceType, action) {
return async (req, res, next) => {
const userId = req.user?.id;
const resourceId = req.params.id;
if (!userId || !resourceId) {
return res.status(401).json({ error: 'Authentication required' });
}
const permissionService = req.app.get('resourcePermissionService');
try {
const hasPermission = await permissionService.canAccess(
userId, resourceType, resourceId, action
);
if (!hasPermission) {
return res.status(403).json({ error: 'Insufficient permissions' });
}
next();
} catch (error) {
console.error('Permission check error:', error);
return res.status(500).json({ error: 'Permission check failed' });
}
};
}
// Example route using the middleware
app.put('/api/projects/:id', requireResourcePermission('PROJECT', 'UPDATE'), (req, res) => {
// Update project logic here
// ...
});
This approach implements a sophisticated resource-based access control system that considers not only user roles but also the relationship between users and specific resources. It supports ownership, team-based access, and hierarchical permissions, providing fine-grained control over who can access what.
Prompt 3: Implementing Audit Logging for Permission Changes
Instead of asking: “Add logging to my user management system”
Use this prompt: “Create a comprehensive audit logging system for permission changes in my Tempo Labs application. Implement detailed logging that captures all permission-related events including role assignments, permission grants/revokes, access attempts, and authentication events. Ensure each log entry includes the actor, action, target, timestamp, and relevant context. Make the logging system tamper-resistant and ensure it cannot be disabled by regular administrators. Include a secure viewing interface with filtering capabilities that is only accessible to security administrators.”
What Tempo Labs will generate:
// Permission audit logging service
class PermissionAuditService {
constructor(auditLogRepository, encryptionService) {
this.auditLogRepository = auditLogRepository;
this.encryptionService = encryptionService;
}
// Log permission-related events
async logPermissionEvent(event) {
try {
// Validate required fields
if (!event.actorId || !event.action || !event.timestamp) {
throw new Error('Missing required audit fields');
}
// Create audit entry
const auditEntry = {
id: this.generateUniqueId(),
actorId: event.actorId,
actorIp: event.actorIp,
actorType: event.actorType || 'USER',
action: event.action,
resourceType: event.resourceType,
resourceId: event.resourceId,
targetUserId: event.targetUserId,
targetRoleId: event.targetRoleId,
targetPermission: event.targetPermission,
previousState: event.previousState,
newState: event.newState,
timestamp: event.timestamp || new Date(),
success: event.success !== undefined ? event.success : true,
reason: event.reason,
metadata: event.metadata,
};
// Sign the entry to detect tampering
auditEntry.signature = await this.signAuditEntry(auditEntry);
// Store the audit entry
await this.auditLogRepository.save(auditEntry);
return auditEntry.id;
} catch (error) {
// Log to a separate error log to ensure we capture failures
console.error('Failed to log permission event:', error);
// Try to log to a fallback mechanism
this.logToFallbackSystem({
error: error.message,
event: event,
timestamp: new Date()
});
// Re-throw for caller awareness
throw error;
}
}
// Generate a unique ID for audit entries
generateUniqueId() {
return `audit-${Date.now()}-${Math.random().toString(36).substr(2, 9)}`;
}
// Sign audit entry to detect tampering
async signAuditEntry(entry) {
// Create a copy without the signature field
const { signature, ...entryWithoutSignature } = entry;
// Convert to string and sign
const entryString = JSON.stringify(entryWithoutSignature);
return this.encryptionService.sign(entryString);
}
// Verify audit entry hasn't been tampered with
async verifyAuditEntry(entry) {
const { signature, ...entryWithoutSignature } = entry;
const entryString = JSON.stringify(entryWithoutSignature);
return this.encryptionService.verify(entryString, signature);
}
// Log to fallback system in case primary logging fails
logToFallbackSystem(errorData) {
// Implement fallback logging (file, separate DB, etc.)
// This ensures we don't lose critical security events
try {
// Example: Write to a local file with restricted permissions
const fs = require('fs');
fs.appendFileSync(
'/var/log/app/security_fallback.log',
JSON.stringify(errorData) + '\n',
{ mode: 0o600 } // Restrictive permissions
);
} catch (fallbackError) {
// Last resort - console
console.error('CRITICAL: Fallback logging failed:', fallbackError);
}
}
// Search audit logs with filtering
async searchAuditLogs(filters, pagination) {
// Verify caller has permission (should be checked before calling)
// Apply filters
const query = {};
if (filters.actorId) query.actorId = filters.actorId;
if (filters.action) query.action = filters.action;
if (filters.resourceType) query.resourceType = filters.resourceType;
if (filters.resourceId) query.resourceId = filters.resourceId;
if (filters.targetUserId) query.targetUserId = filters.targetUserId;
if (filters.success !== undefined) query.success = filters.success;
// Date range
if (filters.startDate || filters.endDate) {
query.timestamp = {};
if (filters.startDate) query.timestamp.$gte = new Date(filters.startDate);
if (filters.endDate) query.timestamp.$lte = new Date(filters.endDate);
}
// Pagination
const limit = pagination?.limit || 50;
const offset = pagination?.offset || 0;
// Get results
const results = await this.auditLogRepository.find(query, {
limit,
offset,
sort: { timestamp: -1 }
});
// Verify each entry hasn't been tampered with
const verifiedResults = [];
for (const entry of results) {
const isValid = await this.verifyAuditEntry(entry);
if (isValid) {
verifiedResults.push(entry);
} else {
// Log potential tampering
console.error(`Tampered audit entry detected: ${entry.id}`);
// Add with tampering flag
verifiedResults.push({
...entry,
_tampered: true
});
}
}
return verifiedResults;
}
}
// Example middleware to log permission-related actions
function logPermissionChange(action) {
return async (req, res, next) => {
const originalSend = res.send;
// Override send to capture response
res.send = function(body) {
res.locals.responseBody = body;
return originalSend.apply(res, arguments);
};
// Continue with request
next();
// After response is sent, log the action
try {
const auditService = req.app.get('permissionAuditService');
await auditService.logPermissionEvent({
actorId: req.user?.id,
actorIp: req.ip,
action: action,
resourceType: req.params.resourceType,
resourceId: req.params.id,
targetUserId: req.body.userId,
targetRoleId: req.body.roleId,
targetPermission: req.body.permission,
previousState: req.body.previousState,
newState: req.body.newState,
success: res.statusCode >= 200 && res.statusCode < 300,
reason: res.statusCode >= 400 ? res.locals.responseBody : undefined,
metadata: {
method: req.method,
url: req.originalUrl,
userAgent: req.headers['user-agent']
}
});
} catch (error) {
console.error('Failed to log permission change:', error);
// Don't block the response for logging errors
}
};
}
// Example route using the middleware
app.post(
'/api/users/:id/roles',
requirePermission(Permissions.USER_UPDATE),
logPermissionChange('ASSIGN_ROLE'),
async (req, res) => {
// Assign role logic here
// ...
}
);
This approach implements a comprehensive audit logging system specifically for permission-related events. It includes tamper detection through cryptographic signatures, fallback mechanisms to ensure critical security events are never lost, and a secure search interface for reviewing logs.
Best Practices for Permission Management in Tempo Labs
To ensure your Tempo Labs applications maintain robust permission security, follow these best practices:
-
Implement the Principle of Least Privilege: Grant users only the permissions they absolutely need to perform their functions. Start with minimal permissions and add more as needed, rather than starting with broad access and restricting later.
-
Centralize Permission Logic: Create a dedicated permission service that handles all permission checks, rather than scattering permission logic throughout your codebase.
-
Use Role-Based Access Control (RBAC): Define clear roles with specific permissions, and assign users to these roles rather than assigning permissions directly to users.
-
Implement Resource-Based Access Control: Consider not just who the user is, but what they’re trying to access. Permission checks should include the relationship between the user and the specific resource.
-
Audit Permission Changes: Log all permission-related events, including role assignments, permission grants, and access attempts. These logs are crucial for security incident investigations.
-
Regularly Review Permissions: Implement a process to periodically review user permissions and remove unnecessary access rights.
-
Fail Secure: When permission checks encounter errors or edge cases, default to denying access rather than granting it.
-
Implement Multi-Factor Authentication: For sensitive operations that modify permissions, require additional verification beyond standard authentication.
-
Test Permission Boundaries: Regularly test your permission system by attempting to access resources with insufficient permissions to ensure your controls are working correctly.
-
Keep Permission Systems Updated: Regularly update your permission management code to address new security vulnerabilities and incorporate improved security practices.
Best Practices
-
Access Control Design:
- Implement role-based access control (RBAC)
- Follow principle of least privilege
- Use fine-grained permissions
- Implement proper role hierarchy
-
Permission Implementation:
- Centralize permission logic
- Validate permissions server-side
- Cache permission checks appropriately
- Log permission changes
-
Security Measures:
- Implement proper authentication first
- Validate all user input
- Use secure session management
- Monitor suspicious activity
-
Maintenance:
- Regular security audits
- Update permission schemes
- Monitor access patterns
- Document permission changes
Conclusion
Implementing secure user permission management in Tempo Labs applications requires careful attention to multiple security aspects. By following the best practices outlined in this guide and implementing proper security measures, you can create a robust permission system that effectively protects your application’s resources.
Remember that security is an ongoing process. Regularly review and update your permission management implementation to address new threats and vulnerabilities. Stay informed about the latest security recommendations and adjust your implementation accordingly.