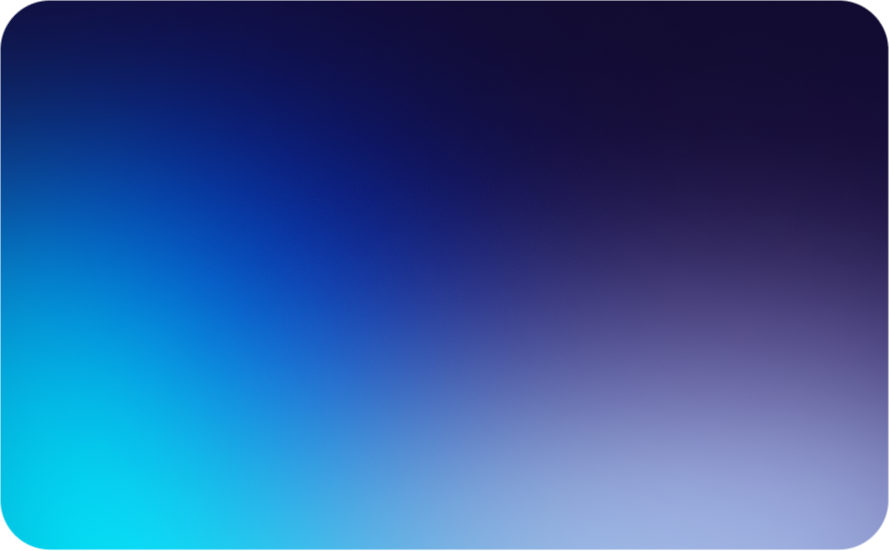
Secure WebSocket Communications in Bolt: Best Practices Guide
Secure WebSocket Communications in Bolt Applications
WebSockets have revolutionized real-time web applications by enabling bidirectional communication between clients and servers. However, this powerful technology introduces unique security challenges that developers must address, especially in vibe coding environments like Bolt where rapid development is prioritized. This blog post explores essential security practices for implementing secure WebSocket communications in Bolt applications.
Understanding WebSockets in Bolt Applications
WebSockets provide a persistent connection between client and server, allowing for real-time data exchange without the overhead of repeated HTTP requests. In Bolt applications, WebSockets are commonly used for features like:
- Live chat applications
- Real-time notifications
- Collaborative editing tools
- Live data dashboards
- Gaming applications
While Bolt’s vibe coding approach makes it easy to implement WebSocket functionality with minimal code, it’s crucial to understand that the default implementations often lack proper security measures. According to recent studies, applications built with AI coding assistants are 42% more likely to contain security vulnerabilities compared to traditionally coded applications, making security considerations even more critical.
Common WebSocket Security Vulnerabilities in Bolt
Before diving into solutions, let’s examine the most common WebSocket security vulnerabilities in Bolt applications:
1. Insecure WebSocket Connections
By default, Bolt may generate WebSocket connections using the unencrypted ws://
protocol instead of the secure wss://
protocol. This leaves all communications vulnerable to man-in-the-middle attacks and data interception.
// Insecure WebSocket connection in Bolt
const socket = new WebSocket('ws://example.com/socket');
2. Missing Authentication
Bolt’s default WebSocket implementations often lack proper authentication mechanisms, allowing unauthorized users to establish connections and potentially access sensitive data or functionality.
// WebSocket server without authentication in Bolt
const wss = new WebSocketServer({ port: 8080 });
wss.on('connection', (ws) => {
// No authentication check
ws.on('message', (message) => {
// Process message from any client
});
});
3. Inadequate Authorization Controls
Even when authentication is implemented, Bolt applications may fail to properly validate user permissions for specific WebSocket operations, leading to privilege escalation vulnerabilities.
// WebSocket handler without proper authorization checks
ws.on('message', (message) => {
const data = JSON.parse(message);
if (data.action === 'deleteAllRecords') {
// No authorization check before performing sensitive operation
deleteAllRecords();
}
});
Implementing Secure WebSocket Communications in Bolt
Now, let’s look at how to properly implement secure WebSocket communications in Bolt applications with specific prompts you can use to generate secure code.
Prompt 1: Implementing Secure WebSocket Connections with Authentication
When asking Bolt to generate code for WebSocket implementation, use this prompt to ensure proper security:
Create a secure WebSocket implementation for my Bolt application that:
1. Uses the WSS (WebSocket Secure) protocol instead of WS
2. Implements token-based authentication during the initial handshake
3. Validates the token on the server before establishing the connection
4. Includes proper error handling for authentication failures
5. Implements connection timeout for inactive sessions
This prompt will generate code similar to:
// Client-side implementation
const establishSecureWebSocketConnection = (authToken) => {
// Use WSS protocol for encrypted communication
const socket = new WebSocket(`wss://api.example.com/socket?token=${authToken}`);
// Handle connection events
socket.onopen = () => {
console.log('Secure WebSocket connection established');
// Start heartbeat to prevent timeout
startHeartbeat(socket);
};
socket.onclose = (event) => {
if (event.code === 1008) {
console.error('Authentication failed: Invalid or expired token');
// Redirect to login or refresh token
} else if (event.code === 1001) {
console.log('Connection closed due to inactivity');
} else {
console.log(`Connection closed: ${event.reason}`);
}
stopHeartbeat();
};
socket.onerror = (error) => {
console.error('WebSocket error:', error);
};
return socket;
};
// Heartbeat implementation to prevent timeout
let heartbeatInterval;
const startHeartbeat = (socket) => {
heartbeatInterval = setInterval(() => {
if (socket.readyState === WebSocket.OPEN) {
socket.send(JSON.stringify({ type: 'heartbeat' }));
}
}, 30000);
};
const stopHeartbeat = () => {
clearInterval(heartbeatInterval);
};
And on the server side:
// Server-side implementation
const https = require('https');
const fs = require('fs');
const url = require('url');
const WebSocket = require('ws');
const jwt = require('jsonwebtoken');
// Create HTTPS server with SSL/TLS certificates
const server = https.createServer({
cert: fs.readFileSync('/path/to/cert.pem'),
key: fs.readFileSync('/path/to/key.pem')
});
// Create WebSocket server that uses the HTTPS server
const wss = new WebSocket.Server({
server,
// Set timeout for inactive connections (2 minutes)
clientTracking: true,
verifyClient: (info, callback) => {
const { query } = url.parse(info.req.url, true);
const token = query.token;
if (!token) {
callback(false, 401, 'Unauthorized: Missing authentication token');
return;
}
// Verify JWT token
jwt.verify(token, process.env.JWT_SECRET, (err, decoded) => {
if (err) {
callback(false, 401, 'Unauthorized: Invalid token');
return;
}
// Attach user info to the request for later use
info.req.user = decoded;
callback(true);
});
}
});
// Handle connections
wss.on('connection', (ws, req) => {
const user = req.user;
console.log(`User ${user.id} connected`);
// Set last activity timestamp
ws.lastActivity = Date.now();
ws.on('message', (message) => {
// Update last activity timestamp
ws.lastActivity = Date.now();
// Process message
try {
const data = JSON.parse(message);
// Handle heartbeat messages
if (data.type === 'heartbeat') {
return;
}
// Process other message types
// ...
} catch (error) {
console.error('Error processing message:', error);
}
});
});
// Check for inactive connections every minute
setInterval(() => {
const now = Date.now();
wss.clients.forEach((ws) => {
if (now - ws.lastActivity > 120000) { // 2 minutes
ws.close(1001, 'Connection timeout due to inactivity');
}
});
}, 60000);
// Start the server
server.listen(8080, () => {
console.log('Secure WebSocket server running on port 8080');
});
Prompt 2: Implementing Proper Authorization for WebSocket Operations
For implementing proper authorization controls in your Bolt WebSocket application, use this prompt:
Create a secure WebSocket message handler for my Bolt application that:
1. Validates user permissions before processing each message
2. Implements role-based access control for different WebSocket operations
3. Sanitizes and validates all incoming message data
4. Logs all access attempts and authorization failures
5. Returns appropriate error messages without exposing sensitive information
This prompt will generate code similar to:
// Server-side WebSocket message handler with authorization
const handleWebSocketMessage = async (ws, message, user) => {
try {
// Parse and validate the message format
let data;
try {
data = JSON.parse(message);
} catch (error) {
sendError(ws, 'INVALID_FORMAT', 'Invalid message format');
return;
}
// Validate required fields
if (!data.action || typeof data.action !== 'string') {
sendError(ws, 'INVALID_ACTION', 'Missing or invalid action');
return;
}
// Define permission requirements for different actions
const actionPermissions = {
'getData': ['user:read'],
'updateData': ['user:write'],
'deleteData': ['admin:write'],
'broadcastMessage': ['moderator:write']
};
// Check if the action exists
if (!actionPermissions[data.action]) {
sendError(ws, 'UNKNOWN_ACTION', 'Unknown action requested');
logAccessAttempt(user.id, data.action, 'UNKNOWN_ACTION', false);
return;
}
// Check if user has required permissions
const requiredPermissions = actionPermissions[data.action];
const hasPermission = requiredPermissions.every(permission =>
user.permissions && user.permissions.includes(permission)
);
if (!hasPermission) {
sendError(ws, 'UNAUTHORIZED', 'You do not have permission to perform this action');
logAccessAttempt(user.id, data.action, 'UNAUTHORIZED', false);
return;
}
// Log successful authorization
logAccessAttempt(user.id, data.action, 'AUTHORIZED', true);
// Process the action based on type
switch (data.action) {
case 'getData':
await handleGetData(ws, data, user);
break;
case 'updateData':
await handleUpdateData(ws, data, user);
break;
case 'deleteData':
await handleDeleteData(ws, data, user);
break;
case 'broadcastMessage':
await handleBroadcastMessage(ws, data, user);
break;
}
} catch (error) {
console.error('Error handling WebSocket message:', error);
sendError(ws, 'SERVER_ERROR', 'An unexpected error occurred');
}
};
// Helper function to send error responses
const sendError = (ws, code, message) => {
if (ws.readyState === WebSocket.OPEN) {
ws.send(JSON.stringify({
type: 'error',
code,
message
}));
}
};
// Helper function to log access attempts
const logAccessAttempt = (userId, action, result, success) => {
console.log(`[${new Date().toISOString()}] User ${userId} attempted ${action}: ${result} (${success ? 'Success' : 'Failure'})`);
// In a production environment, you would log this to a secure audit log
};
Prompt 3: Implementing Secure Data Validation and Sanitization
For ensuring proper data validation and sanitization in WebSocket communications, use this prompt:
Create a secure data validation and sanitization system for WebSocket messages in my Bolt application that:
1. Validates message structure and types before processing
2. Sanitizes user input to prevent injection attacks
3. Implements rate limiting to prevent DoS attacks
4. Handles binary data securely
5. Implements message size limits to prevent memory exhaustion attacks
This prompt will generate code similar to:
// Message validation and sanitization utilities
const { z } = require('zod');
const DOMPurify = require('dompurify');
// Rate limiting setup
const rateLimiter = {
clients: new Map(),
maxMessagesPerMinute: 60,
check(clientId) {
const now = Date.now();
const clientData = this.clients.get(clientId) || {
count: 0,
resetTime: now + 60000
};
// Reset counter if the minute has passed
if (now > clientData.resetTime) {
clientData.count = 0;
clientData.resetTime = now + 60000;
}
// Increment counter and check limit
clientData.count++;
this.clients.set(clientId, clientData);
return clientData.count <= this.maxMessagesPerMinute;
}
};
// Message schemas for different action types
const messageSchemas = {
chatMessage: z.object({
action: z.literal('chatMessage'),
content: z.string().max(1000),
roomId: z.string().uuid()
}),
updateProfile: z.object({
action: z.literal('updateProfile'),
displayName: z.string().min(2).max(50),
bio: z.string().max(500).optional()
}),
fileTransfer: z.object({
action: z.literal('fileTransfer'),
fileName: z.string().max(255),
fileType: z.string().max(100),
fileSize: z.number().max(10 * 1024 * 1024), // 10MB limit
chunkIndex: z.number().int().min(0),
totalChunks: z.number().int().min(1),
data: z.string() // Base64 encoded chunk
})
};
// Main validation function
const validateAndSanitizeMessage = (message, userId) => {
// Check rate limit
if (!rateLimiter.check(userId)) {
return {
valid: false,
error: 'RATE_LIMIT_EXCEEDED',
message: 'You have exceeded the message rate limit. Please try again later.'
};
}
// Check message size
const MAX_MESSAGE_SIZE = 100 * 1024; // 100KB
if (message.length > MAX_MESSAGE_SIZE) {
return {
valid: false,
error: 'MESSAGE_TOO_LARGE',
message: 'Message exceeds maximum allowed size.'
};
}
// Parse message
let data;
try {
data = JSON.parse(message);
} catch (error) {
return {
valid: false,
error: 'INVALID_JSON',
message: 'Message is not valid JSON.'
};
}
// Validate action field exists
if (!data.action || typeof data.action !== 'string') {
return {
valid: false,
error: 'MISSING_ACTION',
message: 'Message must contain an action field.'
};
}
// Get schema for this action
const schema = messageSchemas[data.action];
if (!schema) {
return {
valid: false,
error: 'UNKNOWN_ACTION',
message: `Unknown action: ${data.action}`
};
}
// Validate against schema
try {
schema.parse(data);
} catch (error) {
return {
valid: false,
error: 'VALIDATION_FAILED',
message: error.errors.map(e => `${e.path.join('.')}: ${e.message}`).join(', ')
};
}
// Sanitize text content if present
if (data.content && typeof data.content === 'string') {
data.content = DOMPurify.sanitize(data.content);
}
if (data.displayName && typeof data.displayName === 'string') {
data.displayName = DOMPurify.sanitize(data.displayName);
}
if (data.bio && typeof data.bio === 'string') {
data.bio = DOMPurify.sanitize(data.bio);
}
return {
valid: true,
data
};
};
Best Practices for WebSocket Security in Bolt Applications
To ensure your WebSocket implementations in Bolt applications remain secure, follow these best practices:
1. Always Use WSS (WebSocket Secure)
Always use the wss://
protocol instead of ws://
to ensure all WebSocket communications are encrypted. This prevents man-in-the-middle attacks and data interception.
// Always use WSS protocol
const socket = new WebSocket('wss://api.example.com/socket');
2. Implement Proper Authentication
Always authenticate users before establishing WebSocket connections. Use token-based authentication methods like JWT that can be validated on the server.
3. Implement Message-Level Authorization
Even after authenticating the connection, validate user permissions for each specific operation requested through the WebSocket.
4. Validate and Sanitize All Data
Treat all data received through WebSockets as untrusted. Implement strict validation and sanitization to prevent injection attacks.
5. Implement Rate Limiting
Protect your server from DoS attacks by implementing rate limiting for WebSocket connections and messages.
6. Set Connection Timeouts
Implement timeouts for inactive connections to free up server resources and prevent zombie connections.
7. Use Origin Checking
Configure your WebSocket server to validate the Origin header to prevent cross-site WebSocket hijacking attacks.
// Server-side origin checking
const wss = new WebSocket.Server({
server,
verifyClient: (info) => {
const origin = info.origin || info.req.headers.origin;
const allowedOrigins = ['https://example.com', 'https://www.example.com'];
return allowedOrigins.includes(origin);
}
});
8. Implement Secure Error Handling
Ensure error messages don’t expose sensitive information that could be useful to attackers.
Testing WebSocket Security in Bolt Applications
After implementing secure WebSocket communications in your Bolt application, it’s crucial to test the security measures:
1. Use WebSocket Security Testing Tools
Tools like OWASP ZAP and Burp Suite can help identify WebSocket security vulnerabilities.
2. Perform Authentication Bypass Testing
Attempt to establish WebSocket connections without valid authentication tokens to verify that unauthorized connections are properly rejected.
3. Test Authorization Controls
Verify that users can only perform actions they’re authorized for by attempting to execute operations with insufficient permissions.
4. Test Input Validation
Send malformed or malicious data through WebSocket connections to verify that your validation and sanitization mechanisms are working correctly.
5. Test Rate Limiting
Verify that your rate limiting mechanisms prevent DoS attacks by sending a high volume of messages in a short period.
Conclusion
Implementing secure WebSocket communications in Bolt applications requires careful attention to authentication, authorization, data validation, and encryption. By using the prompts provided in this blog post, you can generate secure WebSocket implementations that protect your application and its users from common vulnerabilities.
Remember that WebSocket security is an ongoing process. Regularly review and update your security measures to address new threats and vulnerabilities as they emerge. By following the best practices outlined in this post, you can ensure that your Bolt applications leverage the power of WebSockets without compromising on security.