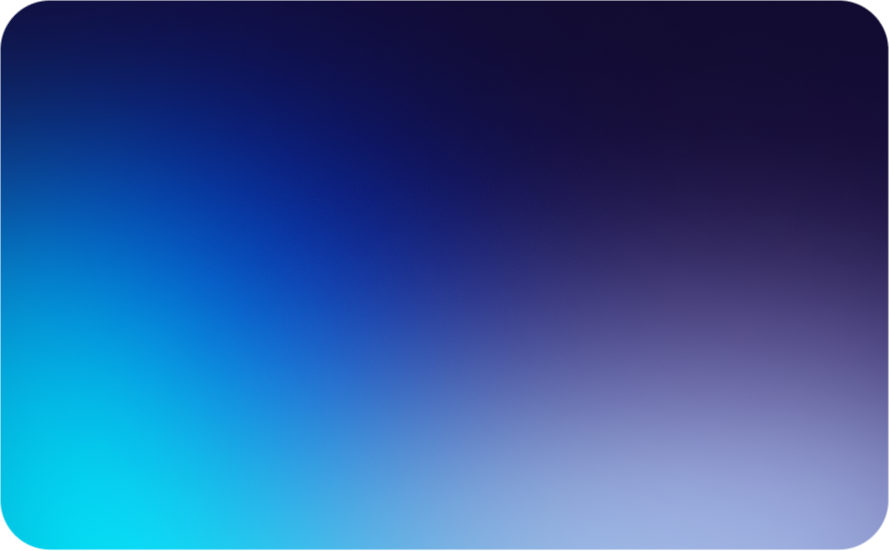
Securing API Endpoints in Base44: Best Practices Guide
In the rapidly evolving landscape of vibe coding, Base44 has emerged as a powerful AI-driven platform that enables users to build fully functional applications through natural language prompts. Launched in 2025, Base44 distinguishes itself with an all-in-one approach that includes built-in authentication, database management, and API functionality. While this integrated approach simplifies development, it also introduces unique security challenges, particularly when it comes to API endpoints.
API endpoints are the gateways through which your application communicates with external services and users. When not properly secured, these endpoints can become vulnerable entry points for attackers seeking to exploit your application. According to recent studies, API attacks have increased by over 300% in the past year, with vibe-coded applications being particularly susceptible due to their rapid development cycles and often overlooked security considerations.
This article provides a comprehensive guide to securing API endpoints in Base44 applications. We’ll examine common API vulnerabilities in vibe-coded applications, demonstrate secure implementation approaches, and provide practical techniques for enhancing API security. By following these practices, you can ensure that your Base44 applications maintain robust protection against unauthorized access while still benefiting from the rapid development that vibe coding enables.
Common API Security Vulnerabilities in Base44 Applications
1. Broken Authentication and Authorization
Base44 includes a built-in authentication system, but when developers instruct the AI to “create an API endpoint,” the resulting code often implements basic functionality without proper authentication checks:
// Example of insecure API endpoint in Base44-generated code
app.get('/api/users', (req, res) => {
// No authentication check
db.collection('users').find({}).toArray((err, users) => {
if (err) return res.status(500).send(err);
res.json(users); // Returns all user data without verification
});
});
This approach creates several security risks:
- Any user can access sensitive data without authentication
- No role-based access control to restrict data based on user permissions
- No validation of API tokens or keys
2. Excessive Data Exposure
Base44’s AI often generates endpoints that return more data than necessary:
// Example of excessive data exposure
app.get('/api/user/:id', (req, res) => {
db.collection('users').findOne({ _id: req.params.id }, (err, user) => {
if (err) return res.status(500).send(err);
res.json(user); // Returns all user data including sensitive fields
});
});
This code is problematic because:
- It returns all user data, including potentially sensitive information like password hashes, payment details, or personal information
- No filtering of response data based on the requester’s permissions
- No data sanitization before sending the response
3. Lack of Rate Limiting
Base44-generated API endpoints typically don’t include rate limiting, making them vulnerable to abuse:
// Example of an API endpoint without rate limiting
app.post('/api/login', (req, res) => {
const { username, password } = req.body;
// Authenticate user
authenticateUser(username, password)
.then(user => {
if (!user) {
return res.status(401).json({ message: 'Invalid credentials' });
}
const token = generateToken(user);
res.json({ token });
})
.catch(err => res.status(500).json({ message: 'Server error' }));
});
This creates security risks such as:
- Vulnerability to brute force attacks
- Potential for denial of service through API flooding
- No protection against scraping or data harvesting
Prompts to Fix API Security Issues in Base44
1. Secure Authentication for API Endpoints
Instead of: “Create an API endpoint to get user data”
Use this prompt:
Implement a secure API endpoint to retrieve user data with proper authentication and authorization. Use JSON Web Tokens (JWT) for authentication with appropriate expiration times. Implement role-based access control to ensure users can only access their own data or data they're authorized to view. Add middleware that validates the JWT on every request, checks user permissions, and implements proper error handling for unauthorized access attempts. Also, ensure the endpoint uses HTTPS and includes appropriate security headers.
This prompt will guide Base44 to generate more secure code like:
// JWT authentication middleware
const authenticateJWT = (req, res, next) => {
const authHeader = req.headers.authorization;
if (!authHeader) {
return res.status(401).json({ message: 'Authentication required' });
}
const token = authHeader.split(' ')[1];
jwt.verify(token, process.env.JWT_SECRET, (err, user) => {
if (err) {
return res.status(403).json({ message: 'Invalid or expired token' });
}
req.user = user;
next();
});
};
// Role-based access control middleware
const authorizeRole = (roles) => {
return (req, res, next) => {
if (!req.user) {
return res.status(401).json({ message: 'Authentication required' });
}
if (!roles.includes(req.user.role)) {
return res.status(403).json({ message: 'Insufficient permissions' });
}
next();
};
};
// Secure API endpoint with authentication and authorization
app.get('/api/users/:id', authenticateJWT, (req, res) => {
// Check if user is requesting their own data or has admin privileges
if (req.params.id !== req.user.id && req.user.role !== 'admin') {
return res.status(403).json({ message: 'Access denied' });
}
db.collection('users').findOne({ _id: req.params.id }, (err, user) => {
if (err) return res.status(500).json({ message: 'Server error' });
if (!user) return res.status(404).json({ message: 'User not found' });
// Return only necessary user data, excluding sensitive information
const { password, securityQuestions, ...userData } = user;
res.json(userData);
});
});
2. Preventing Excessive Data Exposure
Instead of: “Create an API to fetch product data”
Use this prompt:
Implement a secure API endpoint to fetch product data with data filtering and field-level access control. Create a response sanitization layer that removes sensitive or unnecessary fields based on the user's role and permissions. Implement data projection to only return the specific fields requested by the client. Add input validation to prevent SQL/NoSQL injection attacks. Also, implement proper error handling that doesn't expose internal system details and add logging for security events.
This will generate more secure code like:
// Input validation middleware
const validateProductRequest = (req, res, next) => {
const schema = Joi.object({
fields: Joi.string().optional(),
category: Joi.string().alphanum().optional(),
limit: Joi.number().integer().min(1).max(100).optional()
});
const { error } = schema.validate(req.query);
if (error) {
return res.status(400).json({ message: 'Invalid request parameters' });
}
next();
};
// Field-level access control
const getAuthorizedFields = (user, allFields) => {
// Default fields any authenticated user can access
const baseFields = ['id', 'name', 'description', 'category', 'publicPrice'];
// Additional fields for specific roles
if (user.role === 'admin') {
return allFields; // Admins can see everything
} else if (user.role === 'manager') {
return [...baseFields, 'cost', 'margin', 'supplier'];
} else if (user.role === 'sales') {
return [...baseFields, 'inventory', 'discount'];
}
return baseFields;
};
// Secure API endpoint with data filtering
app.get('/api/products', authenticateJWT, validateProductRequest, (req, res) => {
try {
// Parse requested fields or use defaults
const requestedFields = req.query.fields ? req.query.fields.split(',') : [];
// Get fields this user is authorized to see
const authorizedFields = getAuthorizedFields(req.user, [
'id', 'name', 'description', 'category', 'publicPrice',
'cost', 'margin', 'supplier', 'inventory', 'discount',
'internalNotes', 'profitMargin', 'supplierContacts'
]);
// Calculate projection - only include fields that are both requested and authorized
const projection = {};
if (requestedFields.length > 0) {
// User requested specific fields
requestedFields.forEach(field => {
if (authorizedFields.includes(field)) {
projection[field] = 1;
}
});
} else {
// No specific fields requested, return all authorized fields
authorizedFields.forEach(field => {
projection[field] = 1;
});
}
// Build query
const query = {};
if (req.query.category) {
query.category = req.query.category;
}
// Set limit
const limit = req.query.limit || 20;
// Execute query with projection
db.collection('products')
.find(query)
.project(projection)
.limit(limit)
.toArray((err, products) => {
if (err) {
console.error('Database error:', err);
return res.status(500).json({ message: 'Error retrieving products' });
}
res.json({ products });
});
} catch (error) {
console.error('Unexpected error:', error);
res.status(500).json({ message: 'An unexpected error occurred' });
}
});
3. Implementing Rate Limiting and Throttling
Instead of: “Add an API for user registration”
Use this prompt:
Create a secure API endpoint for user registration with comprehensive rate limiting and throttling. Implement IP-based rate limiting to prevent abuse, with a maximum of 5 registration attempts per IP address within a 15-minute window. Add progressive delays between failed attempts and temporary account lockout after multiple failures. Use the express-rate-limit and express-slow-down packages for implementation. Also, implement CAPTCHA verification for registration to prevent automated attacks, and add logging of all registration attempts with appropriate details for security monitoring.
This will generate more secure code like:
const rateLimit = require('express-rate-limit');
const slowDown = require('express-slow-down');
const { body, validationResult } = require('express-validator');
// Rate limiting middleware
const registrationLimiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 5, // 5 requests per window
message: 'Too many registration attempts, please try again later',
standardHeaders: true,
legacyHeaders: false,
keyGenerator: (req) => {
return req.ip; // Use IP address as the key
}
});
// Speed limiting middleware
const registrationSlowDown = slowDown({
windowMs: 15 * 60 * 1000, // 15 minutes
delayAfter: 2, // start slowing down after 2 requests
delayMs: (hits) => hits * 500, // add 500ms delay per hit
keyGenerator: (req) => {
return req.ip; // Use IP address as the key
}
});
// Validation middleware
const validateRegistration = [
body('username').trim().isLength({ min: 3, max: 30 }).withMessage('Username must be between 3 and 30 characters'),
body('email').isEmail().normalizeEmail().withMessage('Valid email is required'),
body('password').isLength({ min: 8 }).withMessage('Password must be at least 8 characters'),
body('captchaToken').notEmpty().withMessage('CAPTCHA verification is required')
];
// Secure registration endpoint with rate limiting and validation
app.post('/api/register',
registrationSlowDown,
registrationLimiter,
validateRegistration,
async (req, res) => {
try {
// Check for validation errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
// Verify CAPTCHA
const captchaValid = await verifyCaptcha(req.body.captchaToken, req.ip);
if (!captchaValid) {
// Log failed CAPTCHA verification
logger.warn({
message: 'Failed CAPTCHA verification',
ip: req.ip,
email: req.body.email,
timestamp: new Date()
});
return res.status(400).json({ message: 'CAPTCHA verification failed' });
}
const { username, email, password } = req.body;
// Check if user already exists
const existingUser = await db.collection('users').findOne({
$or: [{ email }, { username }]
});
if (existingUser) {
// Don't reveal which field caused the conflict
return res.status(409).json({ message: 'Username or email already in use' });
}
// Hash password
const hashedPassword = await bcrypt.hash(password, 12);
// Create new user
const newUser = {
username,
email,
password: hashedPassword,
role: 'user',
createdAt: new Date(),
lastLogin: null
};
await db.collection('users').insertOne(newUser);
// Log successful registration
logger.info({
message: 'User registered successfully',
userId: newUser._id,
email: email,
timestamp: new Date()
});
res.status(201).json({ message: 'Registration successful' });
} catch (error) {
console.error('Registration error:', error);
// Log error
logger.error({
message: 'Registration error',
error: error.message,
stack: error.stack,
timestamp: new Date()
});
res.status(500).json({ message: 'An error occurred during registration' });
}
}
);
// Helper function to verify CAPTCHA
async function verifyCaptcha(token, ip) {
try {
const response = await axios.post('https://www.google.com/recaptcha/api/siteverify', null, {
params: {
secret: process.env.RECAPTCHA_SECRET_KEY,
response: token,
remoteip: ip
}
});
return response.data.success;
} catch (error) {
console.error('CAPTCHA verification error:', error);
return false;
}
}
Best Practices for API Security in Base44 Applications
1. Implement Continuous API Discovery
Base44’s integrated approach can sometimes lead to the creation of “shadow APIs” - endpoints that are generated but not properly documented or secured. Implement a process to:
- Regularly scan your application for all API endpoints
- Document each endpoint’s purpose, inputs, outputs, and security requirements
- Verify that all endpoints adhere to security standards
- Remove or secure any endpoints that aren’t necessary
2. Use Base44’s Built-in Authentication System Properly
Base44 includes a built-in authentication system, but it needs to be properly integrated with your API endpoints:
- Always require authentication for sensitive operations
- Implement role-based access control for different API endpoints
- Use JWT tokens with appropriate expiration times
- Implement token refresh mechanisms for long-lived sessions
- Never store sensitive authentication data in client-side code
3. Implement Proper Error Handling
Secure error handling prevents information leakage while providing useful feedback:
- Return generic error messages to clients that don’t reveal implementation details
- Log detailed error information server-side for debugging
- Use appropriate HTTP status codes
- Validate all input parameters before processing
- Implement global error handling middleware
4. Secure Your API Keys and Secrets
Base44 applications often require integration with third-party services. Protect these credentials:
- Never hardcode API keys or secrets in your code
- Use environment variables for sensitive configuration
- Implement a secrets management solution
- Rotate API keys regularly
- Use different API keys for development and production environments
5. Implement API Versioning
As your application evolves, implement proper API versioning to maintain compatibility:
- Include version information in API URLs (e.g., /api/v1/users)
- Document changes between versions
- Maintain backward compatibility when possible
- Implement deprecation notices for outdated endpoints
- Plan for graceful migration paths
6. Regular Security Testing
Continuously test your API security:
- Perform regular penetration testing
- Use automated security scanning tools
- Implement API fuzzing to test for unexpected inputs
- Conduct code reviews focused on security
- Test for common API vulnerabilities like injection attacks, broken authentication, and excessive data exposure
Conclusion
While Base44’s integrated approach simplifies application development through vibe coding, it’s essential to implement proper security measures for your API endpoints. By using specific, security-focused prompts, you can guide Base44 to generate more secure code that protects your application and its data from common vulnerabilities.
Remember that security is an ongoing process, not a one-time implementation. Regularly review and update your API security practices to address emerging threats and vulnerabilities. By combining the efficiency of vibe coding with robust security practices, you can build applications that are both innovative and secure.
The prompts provided in this article serve as a starting point for securing your Base44 API endpoints. Adapt them to your specific requirements and continue to educate yourself on the latest API security best practices. With diligence and attention to security details, your Base44 applications can provide both rapid development and robust protection against security threats.